Question
Javadoc is used to keep documents for both classes and methods. Question 1 options: True False Question 2 (1 point) Non-static method may be referenced
Javadoc is used to keep documents for both classes and methods.
Question 1 options:
True | |
False |
Question 2 (1 point)
Non-static method may be referenced by class name like MyClass.method1().
Question 2 options:
True | |
False |
Question 3 (1 point)
Methods with void as return type should not return any value.
Question 3 options:
True | |
False |
Question 4 (1 point)
When a double typed value is passed to a method that takes an int typed parameter. It will be accepted and the value will be converted to int type.
Question 4 options:
True | |
False |
Question 5 (1 point)
'this' is not available in static method because static method do not have its own object.
Question 5 options:
True | |
False |
Question 6 (1 point)
Switch statement is the only option to handle multi-way branch.
Question 6 options:
True | |
False |
Question 7 (1 point)
In the scope of this course, java access modifier (i.e. public or private) is mandatory for both instance variable (static or non-static class level variable) declaration.
Question 7 options:
True | |
False |
Question 8 (1 point)
Both String typed and array typed variables have same 'length' field to access its length.
Question 8 options:
True | |
False |
Question 9 (1 point)
The local variables of main method may be accessed by any other methods in the same class.
Question 9 options:
True | |
False |
Question 10 (1 point)
The new operator in Java is used to create a new method.
Question 10 options:
a) True | |
b) False |
Multiple Choice
Question 11 (1.5 points)
What are the equivalent statements of the one line statement:
count++;
Question 11 options:
count = count + 1; | |
count += 1; | |
count ++= 1; | |
count = 1 + count; |
Question 12 (1.5 points)
Given a class named MyClass and its variable myObject1. Choose all correct way(s) to call the public non-static method named method1 of MyClass from another class?
Question 12 options:
MyClass.method1() | |
method1(myObject1) | |
myObject1.method1 | |
myObject1.method1() |
Question 13 (1.5 points)
Choose the correct statement about class constructor.
Question 13 options:
Constrictor is always private. | |
Constructor may be overloaded. | |
new keyword is used to call a class constructor to construct an object of the specified class type. | |
Constructor needs no return type to be specified in its definition. |
Question 14 (1.5 points)
Assume that we have declared an array:
int[] arr = {10, 3, 4, 1, 6};
What is the value of arr[arr[4] - arr[2]]?
Question 14 options:
index out of bound error. | |
2 | |
3 | |
4 |
Question 15 (1.5 points)
Given the code:
public class Test1 { private int method1(int x, int y) { return x + y; }
private int method2(int x, int y) { if (x > y) return x; else return y; }
public static void main(String[] args) { Test1 test1 = new Test1(); System.out.println(test1.method1(test1.method2(3, 5), 10)); } }
What is the output?
Question 15 options:
13 | |
10 | |
15 | |
8 |
Question 16 (1.5 points)
Assume that your main method called method A and B, method A called method C and method B called method D. What happens right after method D returns?
Question 16 options:
The control returns to method B | |
The control returns to main method | |
The program ends | |
The control returns to method A |
Question 17 (1.5 points)
What is the value of the expression:
(int) (7.5 / 3) + 2.0
Question 17 options:
4.5 | |
4 | |
4.0 | |
5.5 |
Save
Question 18 (1.5 points)
The correct expression to check if an index i is an valid index for an array array1?
Question 18 options:
array1.length > i && i >= 0 | |
i <= array1.length - 1 && i >= 0 | |
array1.length > i || i >= 0 | |
array1.length() > i && i >= 0 |
Question 19 (1.5 points)
The way to prevent the value of a local variable from being changed afterwards is to:
Question 19 options:
use the keyword 'final' | |
name the variable using ALL_CAPITAL_CASE | |
use the keyword 'private' | |
use the keyword 'static' |
Question 20 (1.5 points)
Object variables store ____.
Question 20 options:
| |||
| |||
| |||
|
Code Comprehension
Question 21 (5 points)
public class Test1 { public static void main(String[] args) { int a = 7; int b = 8; int c = 5; if (a < b) { if (b <= c) System.out.println("One"); else if (a + b >= c) System.out.println("Two"); else System.out.println("Three"); } else { System.out.println("Four"); } } }
Question 22 (5 points)
public class Test1 { public static void main(String[] args) { for (int i = 5; i > 0; i -= 2) { System.out.print(i + " "); for (int j = 3; j <= i; j++) System.out.print(j + " "); System.out.println(); } } }
Question 23 (5 points)
public class Test1 { public static void main(String[] args) { int x = 18; int y = (x + 2) % 3; switch (y) { case 1: System.out.println("A"); break; case 2: System.out.println("B"); break; case 3: System.out.println("C"); break; default: System.out.println("No of the above"); } } }
Question 24 (5 points)
public class Test1 { public static int method1(int x, int y) { return x + y; } public static int method2(int x, int y) { if (x > y) return x * y; else return x - y; } public static void main(String[] args) { int result = method2(method1(3, 8), 15); System.out.println(result); } }
Question 25 (5 points)
public class Test1 { public static void main(String[] args) { String text = "HELLO WORLD!"; System.out.println("The position is " + text.indexOf("WORLD")); System.out.println(text.substring(0, 1).toUpperCase() + text.substring(1).toLowerCase()); System.out.println(text.substring(0, text.indexOf("O"))); if (text.charAt(5) == 'O') System.out.println("The char at index 5 is O!"); else System.out.println("The char at index 5 is not O!"); } }
Coding Questions
Question 26 (10 points)
Write a method called findMin that returns the smallest element in a partially filled integer array. The parameters of the method are the array called values and the number of elements is stored in integer variable size.
Hint: do not use the length of the array, use size.
Question 27 (15 points)
Create a Java static method named averageOfEven that takes an array of integers as a parameter and returns adouble value representing the average of the values in the array that are even numbers. Your method must work for an int array of any size. Example: If the array contains [13, 48, 16, 99], the method would return (48 + 16) / 2 = 32 as double value.
Hint: the amount of even numbers need to be counted. Example values should not appear in your code.
Question 28 (25 points)
Create a class named Order that stores information about purchases in a store. The details of the class are listed below. Please note the the description instance variable should be no larger than 80 characters.
NOTES: Skip methods that are not mentioned here. Only write the instance variables, constructors and methods asked below, any additional code will not be graded. Import statements or comments are not required.
a) Instance variables (all private except constant) i) TAXRATE a double typed constant that stores the current tax rate. Initialize this value to 0.075. ii) id an int representing the store internal ID number of the item. iii) description a String describing the item. Must be no more than 80 characters. iv) quantity an int representing the number of items purchased. v) price a double representing the cost of each item before taxes.
b) Constructors i) Default constructors that sets all instance variables to default values (0 for numeric variables and empty string for strings). You may also choose to call the parameterized constructor; ii) A parameterized constructor that sets the instance variables to the parameters. So there will be four parameters corresponding to four instance variables. Call mutator to set the value of instance variables rather than assignment if you want validation to be applied.
c) Methods (all public) i) Accessor method for id, quantity and price; ii) Mutator method for id, quantity and price; iii) Mutator method for description. - If the deception is greater than 80 characters, display an error message "Description too long!" and set the description instance variable to the first 80 characters; iv) totalCost a method that returns a double value representing the total cost of the order. Total cost includes the quantity purchased and the tax. So the formula will be quantity * price * (1 + TAXRATE).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
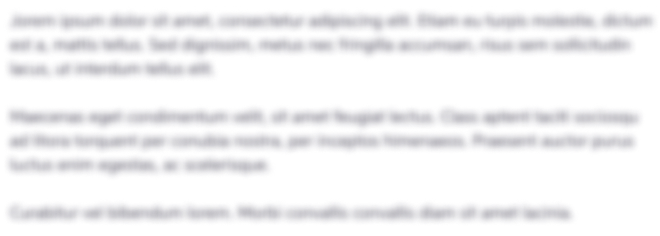
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started