Kernel module overview, Loading and removing kernel module
Please give me the screen shots, and codes of the input and output of all the commands step by step that show the module was loaded, worked and removed. I will thumb up
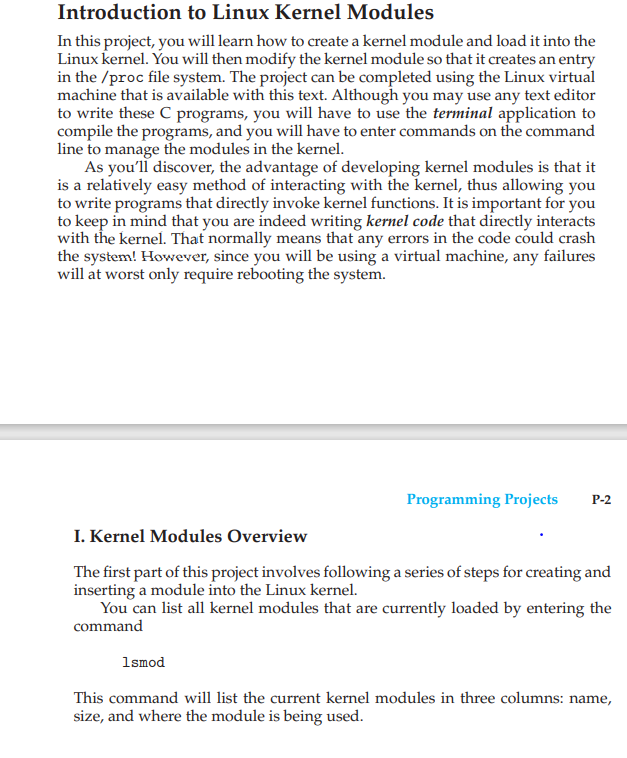
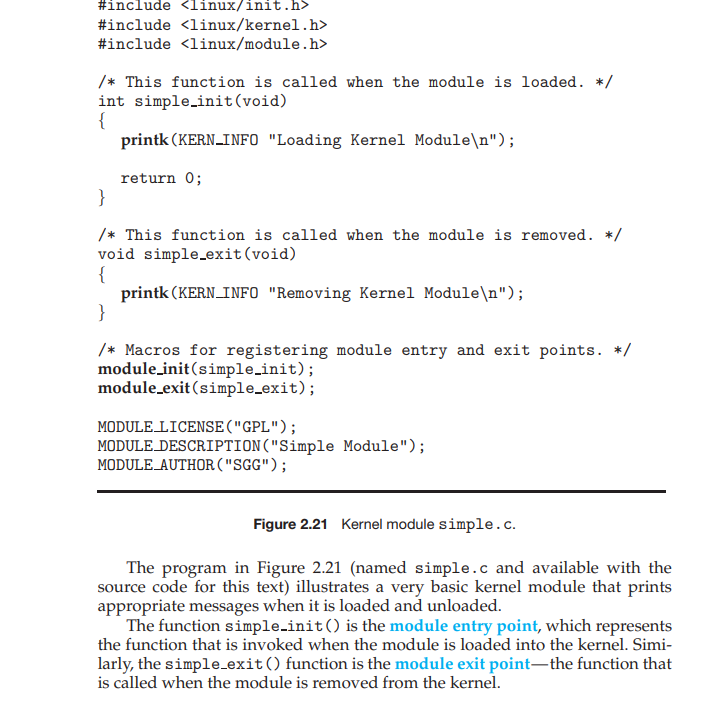
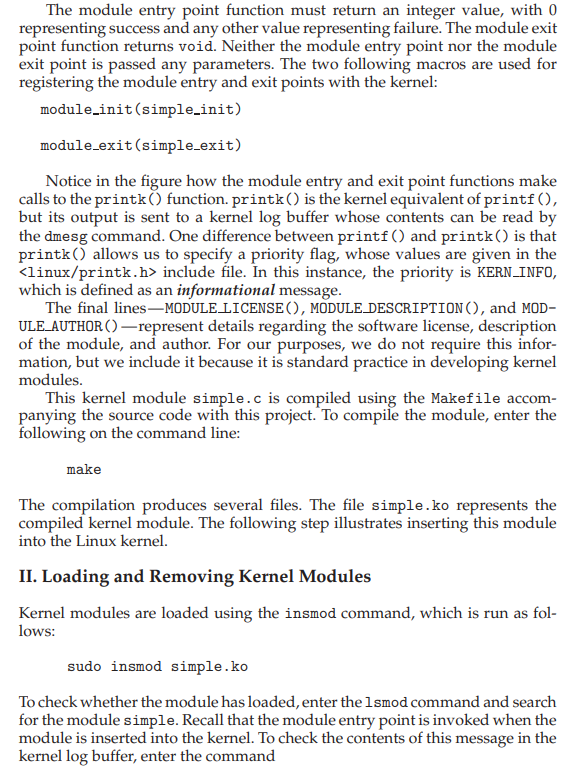
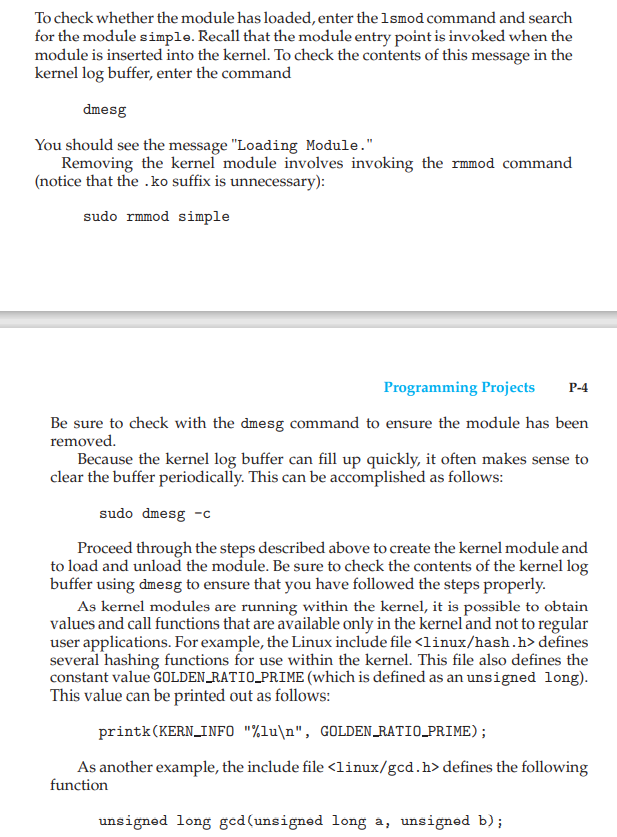
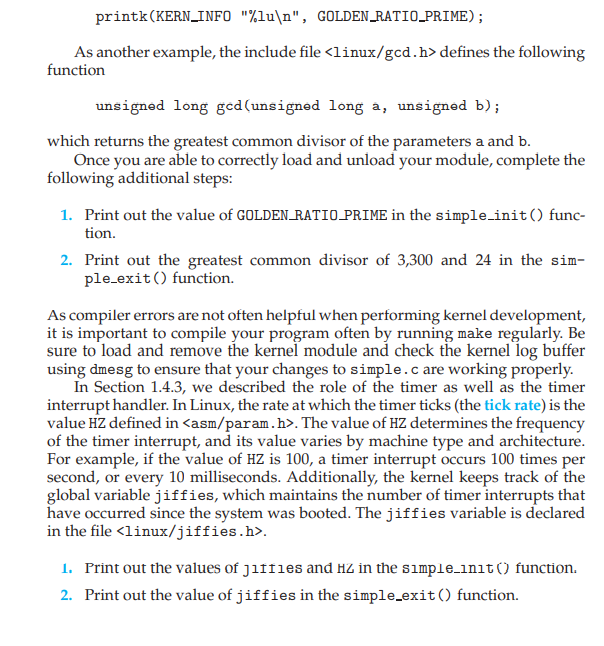
Before proceeding to the next set of exercises, consider how you can use the different values of jiffies in simple init() and simple exit() to determine the number of seconds that have elapsed since the time the kernel module was loaded and then removed.
Introduction to Linux kernel Modules In this project, you will learn how to create a kernel module and load it into the Linux kernel. You will then modify the kernel module so that it creates an entry in the /proc file system. The project can be completed using the Linux virtual machine that is available with this text. Although you may use any text editor to write these C programs, you will have to use the terminal application to compile the programs, and you will have to enter commands on the command line to manage the modules in the kernel. As you'll discover, the advantage of developing kernel modules is that it is a relatively easy method of interacting with the kernel, thus allowing you to write programs that directly invoke kernel functions. It is important for you to keep in mind that you are indeed writing kernel code that directly interacts with the kernel. That normally means that any errors in the code could crash the system! However, since you will be using a virtual machine, any failures will at worst only require rebooting the system. P-2 Programming Projects I. Kernel Modules Overview The first part of this project involves following a series of steps for creating and inserting a module into the Linux kernel. You can list all kernel modules that are currently loaded by entering the command Ismod This command will list the current kernel modules in three columns: name, size, and where the module is being used. #include
#include #include /* This function is called when the module is loaded. */ int simple_init(void) { printk (KERN_INFO "Loading Kernel Module "); return 0; } /* This function is called when the module is removed. */ void simple_exit(void) { printk (KERN_INFO "Removing Kernel Module "); } /* Macros for registering module entry and exit points. */ module_init(simple_init); module_exit (simple_exit); MODULE LICENSE("GPL"); MODULE DESCRIPTION("Simple Module"); MODULE_AUTHOR("SGG"); Figure 2.21 Kernel module simple.c. The program in Figure 2.21 (named simple.c and available with the source code for this text) illustrates a very basic kernel module that prints appropriate messages when it is loaded and unloaded. The function simple.init() is the module entry point, which represents the function that is invoked when the module is loaded into the kernel. Simi- larly, the simple_exit() function is the module exit pointthe function that is called when the module is removed from the kernel. The module entry point function must return an integer value, with 0 representing success and any other value representing failure. The module exit point function returns void. Neither the module entry point nor the module exit point is passed any parameters. The two following macros are used for registering the module entry and exit points with the kernel: module_init(simple_init) module_exit(simple_exit) Notice in the figure how the module entry and exit point functions make calls to the printk() function.printk() is the kernel equivalent of printf(), but its output is sent to a kernel log buffer whose contents can be read by the dmesg command. One difference between printf() and printk() is that printk() allows us to specify a priority flag, whose values are given in the include file. In this instance, the priority is KERN_INFO, which is defined as an informational message. The final linesMODULE LICENSE(), MODULE DESCRIPTION (), and MOD- ULE_AUTHOR() represent details regarding the software license, description of the module, and author. For our purposes, we do not require this infor- mation, but we include it because it is standard practice in developing kernel modules. This kernel module simple.c is compiled using the Makefile accom- panying the source code with this project. To compile the module, enter the following on the command line: make The compilation produces several files. The file simple.ko represents the compiled kernel module. The following step illustrates inserting this module into the Linux kernel. II. Loading and Removing Kernel Modules Kernel modules are loaded using the insmod command, which is run as fol- lows: sudo insmod simple.ko To check whether the module has loaded, enter the lsmod command and search for the module simple. Recall that the module entry point is invoked when the module is inserted into the kernel. To check the contents of this message in the kernel log buffer, enter the command To check whether the module has loaded, enter the lsmod command and search for the module simple. Recall that the module entry point is invoked when the module is inserted into the kernel. To check the contents of this message in the kernel log buffer, enter the command dmesg You should see the message "Loading Module." Removing the kernel module involves invoking the rmmod command (notice that the .ko suffix is unnecessary): sudo rmmod simple P-4 Programming Projects Be sure to check with the dmesg command to ensure the module has been removed. Because the kernel log buffer can fill up quickly, it often makes sense to clear the buffer periodically. This can be accomplished as follows: sudo dmesg -c Proceed through the steps described above to create the kernel module and to load and unload the module. Be sure to check the contents of the kernel log buffer using dmesg to ensure that you have followed the steps properly. As kernel modules are running within the kernel, it is possible to obtain values and call functions that are available only in the kernel and not to regular user applications. For example, the Linux include file defines several hashing functions for use within the kernel. This file also defines the constant value GOLDEN_RATIO_PRIME (which is defined as an unsigned long). This value can be printed out as follows: printk(KERN_INFO "%lu ", GOLDEN_RATIO_PRIME); As another example, the include file defines the following function unsigned long ged(unsigned long a, unsigned b); printk(KERN_INFO "%lu ", GOLDEN_RATIO_PRIME); As another example, the include file defines the following function unsigned long ged(unsigned long a, unsigned b); which returns the greatest common divisor of the parameters a and b. Once you are able to correctly load and unload your module, complete the following additional steps: 1. Print out the value of GOLDEN_RATIO PRIME in the simple_init() func- tion. 2. Print out the greatest common divisor of 3,300 and 24 in the sim- ple_exit() function. As compiler errors are not often helpful when performing kernel development, it is important to compile your program often by running make regularly. Be sure to load and remove the kernel module and check the kernel log buffer using dmesg to ensure that your changes to simple.c are working properly. In Section 1.4.3, we described the role of the timer as well as the timer interrupt handler. In Linux, the rate at which the timer ticks (the tick rate) is the value HZ defined in . The value of HZ determines the frequency of the timer interrupt, and its value varies by machine type and architecture. For example, if the value of HZ is 100, a timer interrupt occurs 100 times per second, or every 10 milliseconds. Additionally, the kernel keeps track of the global variable jiffies, which maintains the number of timer interrupts that have occurred since the system was booted. The jiffies variable is declared in the file . 1. Print out the values of jifties and HZ in the simple_init() function. 2. Print out the value of jiffies in the simple_exit() function. Introduction to Linux kernel Modules In this project, you will learn how to create a kernel module and load it into the Linux kernel. You will then modify the kernel module so that it creates an entry in the /proc file system. The project can be completed using the Linux virtual machine that is available with this text. Although you may use any text editor to write these C programs, you will have to use the terminal application to compile the programs, and you will have to enter commands on the command line to manage the modules in the kernel. As you'll discover, the advantage of developing kernel modules is that it is a relatively easy method of interacting with the kernel, thus allowing you to write programs that directly invoke kernel functions. It is important for you to keep in mind that you are indeed writing kernel code that directly interacts with the kernel. That normally means that any errors in the code could crash the system! However, since you will be using a virtual machine, any failures will at worst only require rebooting the system. P-2 Programming Projects I. Kernel Modules Overview The first part of this project involves following a series of steps for creating and inserting a module into the Linux kernel. You can list all kernel modules that are currently loaded by entering the command Ismod This command will list the current kernel modules in three columns: name, size, and where the module is being used. #include #include #include /* This function is called when the module is loaded. */ int simple_init(void) { printk (KERN_INFO "Loading Kernel Module "); return 0; } /* This function is called when the module is removed. */ void simple_exit(void) { printk (KERN_INFO "Removing Kernel Module "); } /* Macros for registering module entry and exit points. */ module_init(simple_init); module_exit (simple_exit); MODULE LICENSE("GPL"); MODULE DESCRIPTION("Simple Module"); MODULE_AUTHOR("SGG"); Figure 2.21 Kernel module simple.c. The program in Figure 2.21 (named simple.c and available with the source code for this text) illustrates a very basic kernel module that prints appropriate messages when it is loaded and unloaded. The function simple.init() is the module entry point, which represents the function that is invoked when the module is loaded into the kernel. Simi- larly, the simple_exit() function is the module exit pointthe function that is called when the module is removed from the kernel. The module entry point function must return an integer value, with 0 representing success and any other value representing failure. The module exit point function returns void. Neither the module entry point nor the module exit point is passed any parameters. The two following macros are used for registering the module entry and exit points with the kernel: module_init(simple_init) module_exit(simple_exit) Notice in the figure how the module entry and exit point functions make calls to the printk() function.printk() is the kernel equivalent of printf(), but its output is sent to a kernel log buffer whose contents can be read by the dmesg command. One difference between printf() and printk() is that printk() allows us to specify a priority flag, whose values are given in the include file. In this instance, the priority is KERN_INFO, which is defined as an informational message. The final linesMODULE LICENSE(), MODULE DESCRIPTION (), and MOD- ULE_AUTHOR() represent details regarding the software license, description of the module, and author. For our purposes, we do not require this infor- mation, but we include it because it is standard practice in developing kernel modules. This kernel module simple.c is compiled using the Makefile accom- panying the source code with this project. To compile the module, enter the following on the command line: make The compilation produces several files. The file simple.ko represents the compiled kernel module. The following step illustrates inserting this module into the Linux kernel. II. Loading and Removing Kernel Modules Kernel modules are loaded using the insmod command, which is run as fol- lows: sudo insmod simple.ko To check whether the module has loaded, enter the lsmod command and search for the module simple. Recall that the module entry point is invoked when the module is inserted into the kernel. To check the contents of this message in the kernel log buffer, enter the command To check whether the module has loaded, enter the lsmod command and search for the module simple. Recall that the module entry point is invoked when the module is inserted into the kernel. To check the contents of this message in the kernel log buffer, enter the command dmesg You should see the message "Loading Module." Removing the kernel module involves invoking the rmmod command (notice that the .ko suffix is unnecessary): sudo rmmod simple P-4 Programming Projects Be sure to check with the dmesg command to ensure the module has been removed. Because the kernel log buffer can fill up quickly, it often makes sense to clear the buffer periodically. This can be accomplished as follows: sudo dmesg -c Proceed through the steps described above to create the kernel module and to load and unload the module. Be sure to check the contents of the kernel log buffer using dmesg to ensure that you have followed the steps properly. As kernel modules are running within the kernel, it is possible to obtain values and call functions that are available only in the kernel and not to regular user applications. For example, the Linux include file defines several hashing functions for use within the kernel. This file also defines the constant value GOLDEN_RATIO_PRIME (which is defined as an unsigned long). This value can be printed out as follows: printk(KERN_INFO "%lu ", GOLDEN_RATIO_PRIME); As another example, the include file defines the following function unsigned long ged(unsigned long a, unsigned b); printk(KERN_INFO "%lu ", GOLDEN_RATIO_PRIME); As another example, the include file defines the following function unsigned long ged(unsigned long a, unsigned b); which returns the greatest common divisor of the parameters a and b. Once you are able to correctly load and unload your module, complete the following additional steps: 1. Print out the value of GOLDEN_RATIO PRIME in the simple_init() func- tion. 2. Print out the greatest common divisor of 3,300 and 24 in the sim- ple_exit() function. As compiler errors are not often helpful when performing kernel development, it is important to compile your program often by running make regularly. Be sure to load and remove the kernel module and check the kernel log buffer using dmesg to ensure that your changes to simple.c are working properly. In Section 1.4.3, we described the role of the timer as well as the timer interrupt handler. In Linux, the rate at which the timer ticks (the tick rate) is the value HZ defined in . The value of HZ determines the frequency of the timer interrupt, and its value varies by machine type and architecture. For example, if the value of HZ is 100, a timer interrupt occurs 100 times per second, or every 10 milliseconds. Additionally, the kernel keeps track of the global variable jiffies, which maintains the number of timer interrupts that have occurred since the system was booted. The jiffies variable is declared in the file . 1. Print out the values of jifties and HZ in the simple_init() function. 2. Print out the value of jiffies in the simple_exit() function