Question
Lab1Ques2.zip contains the driver code (Lab1_Driver.java) that creates instances of Car and Truck types, and other necessary classes. Execute the code and then answer the
Lab1Ques2.zip contains the driver code (Lab1_Driver.java) that creates instances of Car and Truck types, and other necessary classes. Execute the code and then answer the following questions as comments in Lab1_Driver.java.
Briefly explain why the output of the code does not display the details of the Truck and Car instances. Then provide the code that should be added in the Car, Truck and Vehicle classes, so that the objects are displayed as shown in the sample output. Use this and super keywords as necessary for code reuse.
Uncomment the commented code block in driver file and provide a one sentence explanation of why each of the last two statements are invalid and a possible fix for each statement.
Car.java
public class Car extends Vehicle {
private int numOfDoors;
// example using this/super keyword
public Car() {
this("unknown", "unknown", 0);
}
public Car(String vehicleID, String manufacturer, int numDoors) {
super(vehicleID, manufacturer);
this.numOfDoors = numDoors;
}
}
Insuarable.java
public interface Insurable {
public String getLicencePlate();
public void setLicencePlate(String s);
}
Lab1_Driver.java
public class Lab1_Driver {
public static void main(String[] args) {
Insurable[] arr = new Insurable[4];
Truck t1 = new Truck("567", "Honda", 260);
Truck t2 = new Truck("890", "Ford", 300);
Car c1 = new Car("234", "Honda", 2);
Car c2 = new Car("432", "Hyundai", 4);
t1.setLicencePlate("AAA 111");
t2.setLicencePlate("BBB 222");
c1.setLicencePlate("CCC 333");
c2.setLicencePlate("DDD 444");
arr[0]=t1;
arr[1]=t2;
arr[2]=c1;
arr[3]=c2;
//Print the details of the Cars and Trucks instances (for Question 2a)
for (Insurable i : arr) {
System.out.println(i);
}
//Commented block for Question 2b
/*Car c3 = new Car("432", "Hyundai", 4);
Vehicle c4 = new Car("432", "Hyundai", 4);
Car cc = c4;
Vehicle c5 = new Vehicle("432", "Hyundai");*/
}
}
Truck.java
public class Truck extends Vehicle {
private int horsepower;
// example using implicit super() call to no-arg constructor
public Truck() {
horsepower = 0;
}
public Truck(String vehicleID, String manufacturer, int horsepower) {
super(vehicleID, manufacturer);
this.horsepower = horsepower;
}
}
Vehicle.java
public abstract class Vehicle implements Insurable {
protected String vehicleID;
protected String manufacturer;
protected String licencePlateNum;
public Vehicle() {
this("unknown", "unknown");
}
public Vehicle(String vehicleID, String manufacturer) {
this.vehicleID = vehicleID;
this.manufacturer = manufacturer;
this.licencePlateNum = " ";
}
public String getLicencePlate() {
return licencePlateNum;
}
public void setLicencePlate(String lpn) {
licencePlateNum = lpn;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
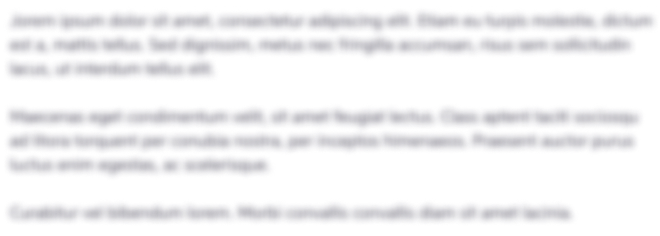
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started