Question
Language concepts 1. What is a variable? A) A name and a type B) A name and a value C) A name, a type, and
Language concepts
1. What is a variable?
A) A name and a type
B) A name and a value
C) A name, a type, and a value
D) A name, a type, and a memory location
2. All objects have what in common?
A) They have a name
B) They have a determinate value
C) They have a size
D) They can be assigned to
3. Which of the following is mandatory for a function definition?
A) A function body
B) A return value
C) A parameter
D) An enclosing class
4. Which expression performs a function call?
A) *f
B) &f
C) f[0]
D) f()
5. What is the meaning of the return value of the main function?
A) Non-zero indicates program success, zero indicates program failure.
B) Zero indicates program success, non-zero indicates program failure.
6. A function with a return type of void signifies
A) The function does not return
B) The function has no side-effects
C) The function has no return value
D) The function requires no arguments
7. A sequence of operators and their operands, followed by a semicolon is a(n):
A) Selection statement
B) Declaration statement
C) Compound statement
D) Expression statement
8. All assignment and compound assignment expressions return:
A) The left operand
B) The right operand
C) A temporary value
D) Nothing
9. All non-assignment, arithmetic operators, when used on values of type int or double, return:
A) The left operand
B) The right operand
C) A temporary value
D) Nothing
10. The return type of a comparison operator is:
A) bool
B) char
C) int
D) double
11. A unary operator requires how many operands?
A) 0
B) 1
C) 2
D) 3
12. What variable scope has the longest lifetime?
A) Block scope
B) Global scope
C) Class scope
D) Function scope
13. A variable whose initial value cannot be replaced must be declared as:
A) volatile
B) static
C) const
D) auto
14. When a value undergoes conversion, what happens?
A) The value gets moved
B) The value changes
C) A new value with the same type gets created
D) A new value with a different type gets created
15. C-style cast expressions and functional cast expressions are examples of:
A) Explicit conversions
B) Implicit conversions
C) Standard conversions
D) User-defined conversions
16. Which of the following describes a conversion where no information can be lost?
A) double to int
B) int to char
C) bool to int
D) int to double
17. Variables declared directly within a class are called:
A) Properties
B) Data members
C) Member variables
D) Attributes
18. Functions declared directly within a class are called:
A) Member functions
B) Subprocedures
C) Methods
D) Operations
19. A private member of a class can be accessed from:
A) Any function
B) Any function inside the same class
C) Any private function
D) Any private function inside the same class
20. A public member of a class can be accessed from:
A) Any function
B) Any function inside the same class
C) Any public function
D) Any public function inside the same class
21. The kinds of statements that repeatedly execute code are generally known as:
A) Cycles
B) Loops
C) Repetitions
D) Recurrences
22. Which of the following is always true about referrences?
A) A reference must be declared with an initializer
B) A reference does not store anything
C) A reference stores an address
D) A reference can refer to multiple variables over it's lifetime
23. A pointer with the value 0 is known as:
A) A dangling pointer
B) An empty pointer
C) A void pointer
D) A null pointer
24. A condition is convertible to:
A) void*
B) int
C) bool
D) string
25. Another term for a compound statement is:
A) An aggregate
B) A fragment
C) A sequence
D) A block
Standard library concepts
26. Which of the following is a valid expression that returns the number of elements in a vector
A) sizeof(v)
B) v.length()
C) v.size()
D) v.count()
27. When integer literals are used as arguments, almost all of the common mathematical functions return a value of type:
A) int
B) float
C) double
D) long double
28. What is the name of the function that raises a number to a power?
A) exp
B) pow
C) raise
D) x^y
29. Which of the following is a valid operator that performs stream insertion?
A) <<
B) <-
C) >>
D) ->
30. Which of the following is a valid operator that performs stream extraction?
A) <<
B) <-
C) >>
D) ->
31. Which of the following is a valid expression that erases all elements from a vector named v?
A) v.reset()
B) v.erase_all()
C) v.empty()
D) v.clear()
32. Which of the following is a valid expression that returns the first element of a vector named v?
A) v.begin()
B) v.first()
C) v.front()
D) v[1]
33. Which of the following is a valid expression that returns the last element of a vector named v?
A) v.last()
B) v.back()
C) v.end()
D) v[v.size()]
34. What is the name of the function that reads a line from an input stream?
A) readline
B) readln
C) getline
D) getln
35. The rand function never returns a value...
A) Less than 0
B) Less than or equal to 0
C) Less than 1
D) Less than or equal to 1
36. The string type represents:
A) A sequence of bits
B) A sequence of bytes
C) A sequence of characters
D) A sequence of integers
37. One valid set of arguments to string::erase is:
A) A starting index and an index past the last element to erase
B) A starting index and an index at the last element to erase
C) A starting index and the number of elements that should remain after the operation
D) A starting index and the number of elements to erase
38. What is the name of the function that can convert an integer into a string?
A) itos
B) itoa
C) int_to_string
D) to_string
39. What is the name of the function that can convert a string to an integer?
A) stoi
B) atoi
C) string_to_int
D) to_int
40. Assuming c is a variable of type char, which of the following is a valid expression that extracts the next character, including whitespace, from cin and assigns it to c?
A) cin >> c
B) cin.get(c)
C) cin.getc(c)
D) cin.getchar(c)
41. Assuming s is a variable of type string, which of the following is a valid expression that extracts the next word (non-whitespace characters) from cin and assigns it to s?
A) cin >> s
B) cin.getw(s)
C) cin.getword(s)
D) cin.gets(s)
42. If cin is associated with the keyboard, what key combination signals the end of all further user input without necessarily terminating the program?
A) CTRL-C
B) CTRL-D
C) CTRL-Z
D) CTRL-\
43. What does assert do?
A) Aborts the program if the given expression is false
B) Aborts the program if the given expression is true
C) Exits the program if the given expression is false
D) Exits the program if the given expression is true
44. What is the name of the function that exchanges the values between two variables of the same type?
A) switch
B) xchg
C) swap
D) exchange
45. The expression time(0) returns a value that represents the current time in...
A) Microseconds
B) Nanoseconds
C) Milliseconds
D) Seconds
Code analyzation:
46. What does the following code print?
int k = 1; cout << k++ << ' '; cout << ++k << endl;
47. What does the following code print?
int k = 5; cout << --k << ' '; cout << k-- << endl;
48. What does the following code print?
int x = 1; int y = 0; { int x = 2; y = x + x; { int y = 4; x = y + 1; } } cout << x << ' ' << y << endl;
49. What does the following code print?
int x = 0; { int x = 1; cout << x << ' '; } cout << x << endl;
50. What does the following code print?
int x = true; double y = int(5.4) + 0.1 * true + false; cout << (x - y) << endl;
51. What does the following code print?
int x = 3.4; double d = false; cout << (x + d) << endl;
52. What does the following code print?
cout << (100 % 5) << endl;
53. What does the following code print?
cout << (31 % 2) << endl;
54. What does the following code print?
class T { public: T() { cout << 'A'; } char f() { return 'B'; } char g(char x) { if (x == 'A') return 'C'; else return 'D'; } }; int main() { T t, u; t.g('B'); u.f(); t.g('A'); }
55. What does the following code print?
class T { public: T() { data = 'X'; cout << 'A'; } void f() const { cout << 'B' << data; } char g(char x) { cout << 'C' << x; return x; } char data; }; int main() { T a; a.f(); a.data = a.g('Z'); a.g('Q'); a.f(); return 0; }
Coding
56. Write C++ code that reads 2 floating-point numbers and displays the sum of those numbers.
57. Write C++ code that reads 1 line and displays the length of that line.
58. Define the body of is_even, this function shall return true if the value of x is an even integer; false shall be returned otherwise.
bool is_even(int x) { }
59. Define the body of sum_of_double, this function shall return the sum of the values found in v or 0 if v is empty.
double sum_of_doubles(const vector
60. Define the body of find_double, this function shall return the index of the first occurrence of the value of x found in one of the elements of v or -1 if no such value was found. Do not perform function calls in your code.
int find_double(const vector
61. Complete the following program; this program should display a random integer value between a minimum and maximum value (inclusive) specified by the user.
#include
62. Define the body of factorial, this function shall return the factorial of x or 1 if x is less than or equal to 1. Examples: factorial of 0 is 1, factorial of 1 is 1, factorial of 3 is 3 * 2 * 1 = 6, factorial of 4 is 4 * 3 * 2 * 1 = 24, factorial of 5 is 5 * 4 * 3 * 2 * 1 = 120.
int factorial(int x) { }
63. Define the body of is_prime, this function shall return true if x is a prime integer and false otherwise.
bool is_prime(int x) { }
It's a study guide but i want a refrence if possible Thank you so much.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
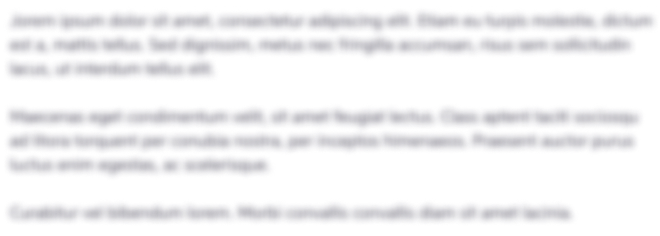
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started