Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Language = Python Hi, could you please fill in the following methods to complete this directed graph class? The test cases are included. Thanks. import
Language = Python Hi, could you please fill in the following methods to complete this directed graph class? The test cases are included. Thanks.
import heapq from collections import deque class DirectedGraph: """ Class to implement directed weighted graph - duplicate edges not allowed - loops not allowed - only positive edge weights - vertex names are integers """ def __init__(self, start_edges=None): """ Store graph info as adjacency matrix DO NOT CHANGE THIS METHOD IN ANY WAY """ self.v_count = 0 self.adj_matrix = [] # populate graph with initial vertices and edges (if provided) # before using, implement add_vertex() and add_edge() methods if start_edges is not None: v_count = 0 for u, v, _ in start_edges: v_count = max(v_count, u, v) for _ in range(v_count + 1): self.add_vertex() for u, v, weight in start_edges: self.add_edge(u, v, weight) def __str__(self): """ Return content of the graph in human-readable form DO NOT CHANGE THIS METHOD IN ANY WAY """ if self.v_count == 0: return 'EMPTY GRAPH ' out = ' |' out += ' '.join(['{:2}'.format(i) for i in range(self.v_count)]) + ' ' out += '-' * (self.v_count * 3 + 3) + ' ' for i in range(self.v_count): row = self.adj_matrix[i] out += '{:2} |'.format(i) out += ' '.join(['{:2}'.format(w) for w in row]) + ' ' out = f"GRAPH ({self.v_count} vertices): {out}" return out # ------------------------------------------------------------------ # def add_vertex(self) -> int: """ TODO: Write this implementation """ self.adj_matrix.append() def add_edge(self, src: int, dst: int, weight=1) -> None: """ TODO: Write this implementation """ pass def remove_edge(self, src: int, dst: int) -> None: """ TODO: Write this implementation """ pass def get_vertices(self) -> []: """ TODO: Write this implementation """ pass def get_edges(self) -> []: """ TODO: Write this implementation """ pass def is_valid_path(self, path: []) -> bool: """ TODO: Write this implementation """ pass def dfs(self, v_start, v_end=None) -> []: """ TODO: Write this implementation """ def bfs(self, v_start, v_end=None) -> []: """ TODO: Write this implementation """ def has_cycle(self): """ TODO: Write this implementation """ pass def dijkstra(self, src: int) -> []: """ TODO: Write this implementation """ # use a priority queue to order our search. pass def _enqueue(self, val): self.adj_matrix.append(val) def _dequeue(self, val): self.adj_matrix.pop(val) if __name__ == '__main__': print(" PDF - method add_vertex() / add_edge example 1") print("----------------------------------------------") g = DirectedGraph() print(g) for _ in range(5): g.add_vertex() print(g) edges = [(0, 1, 10), (4, 0, 12), (1, 4, 15), (4, 3, 3), (3, 1, 5), (2, 1, 23), (3, 2, 7)] for src, dst, weight in edges: g.add_edge(src, dst, weight) print(g) print(" PDF - method get_edges() example 1") print("----------------------------------") g = DirectedGraph() print(g.get_edges(), g.get_vertices(), sep=' ') edges = [(0, 1, 10), (4, 0, 12), (1, 4, 15), (4, 3, 3), (3, 1, 5), (2, 1, 23), (3, 2, 7)] g = DirectedGraph(edges) print(g.get_edges(), g.get_vertices(), sep=' ') print(" PDF - method is_valid_path() example 1") print("--------------------------------------") edges = [(0, 1, 10), (4, 0, 12), (1, 4, 15), (4, 3, 3), (3, 1, 5), (2, 1, 23), (3, 2, 7)] g = DirectedGraph(edges) test_cases = [[0, 1, 4, 3], [1, 3, 2, 1], [0, 4], [4, 0], [], [2]] for path in test_cases: print(path, g.is_valid_path(path)) print(" PDF - method dfs() and bfs() example 1") print("--------------------------------------") edges = [(0, 1, 10), (4, 0, 12), (1, 4, 15), (4, 3, 3), (3, 1, 5), (2, 1, 23), (3, 2, 7)] g = DirectedGraph(edges) for start in range(5): print(f'{start} DFS:{g.dfs(start)} BFS:{g.bfs(start)}') print(" PDF - method has_cycle() example 1") print("----------------------------------") edges = [(0, 1, 10), (4, 0, 12), (1, 4, 15), (4, 3, 3), (3, 1, 5), (2, 1, 23), (3, 2, 7)] g = DirectedGraph(edges) edges_to_remove = [(3, 1), (4, 0), (3, 2)] for src, dst in edges_to_remove: g.remove_edge(src, dst) print(g.get_edges(), g.has_cycle(), sep=' ') edges_to_add = [(4, 3), (2, 3), (1, 3), (4, 0)] for src, dst in edges_to_add: g.add_edge(src, dst) print(g.get_edges(), g.has_cycle(), sep=' ') print(' ', g) print(" PDF - dijkstra() example 1") print("--------------------------") edges = [(0, 1, 10), (4, 0, 12), (1, 4, 15), (4, 3, 3), (3, 1, 5), (2, 1, 23), (3, 2, 7)] g = DirectedGraph(edges) for i in range(5): print(f'DIJKSTRA {i} {g.dijkstra(i)}') g.remove_edge(4, 3) print(' ', g) for i in range(5): print(f'DIJKSTRA {i} {g.dijkstra(i)}')
Step by Step Solution
There are 3 Steps involved in it
Step: 1
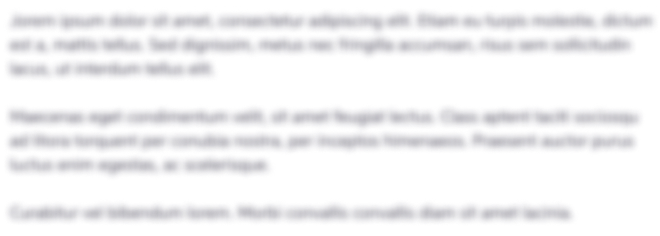
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started