Question
---------------------------------- LifeTestCases? public class LifeTestCases { //A class that stores a set of test configurations for the Game of Life //as a simple 2D array
----------------------------------
LifeTestCases?
public class LifeTestCases { //A class that stores a set of test configurations for the Game of Life //as a simple 2D array of Strings. X's are living cells, any other // character is a dead cell. private static String[][] testBoards = { //1. A test case that should die out after exactly 8 generations { " ", " XXX ", " XXXXX ", " XXXX ", " "}, //2. A glider gun {"........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", ".............XX.........................", "............X...X.......................", "...........X.....X.......X..............", ".XX........X...X.XX....X.X..............", ".XX........X.....X...XX.................", "............X...X....XX............XX...", ".............XX......XX............XX...", ".......................X.X..............", ".........................X..............", "........................................"}, //3. Eight gliders that collide to form a glider gun {"...........................X....................................................", "...........................X.X................X.................................", "...........................XX.................X.X...............................", "...X.X..........X.............................XX................................", "....XX...........XX.............................................................", "....X...........XX..............................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", ".............XX............................XX...................................", "..............XX...........................X.X.........XXX......................", ".............X.............................X...........X........................", "..............................X.........................X.......................", "..............................XX................................................", ".............................X.X................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................", "................................................................................"}, //4. A "harvester" {"........................................", "......................................XX", ".....................................X.X", "....................................X...", "...................................X....", "..................................X.....", ".................................X......", "................................X.......", "...............................X........", "..............................X.........", ".............................X..........", "............................X...........", "...........................X............", "..........................X.............", ".........................X..............", "........................X...............", ".......................X................", "......................X.................", ".....................X..................", "....................X...................", "...................X....................", "..................X.....................", ".................X......................", "................X.......................", "...............X........................", "..............X.........................", ".............X..........................", "............X...........................", "...........X............................", "..........X.............................", ".........X..............................", "........X...............................", ".......X................................", "......X.................................", ".XXXXX..................................", ".XXXX...................................", ".X.XX...................................", "........................................", "........................................", "........................................"}, //5. An "r-pentomino". It is unstable for 1103 generations if the board is infinite. {"........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "..................XX....................", ".................XX.....................", "..................X.....................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................", "........................................"} }; //This will convert the selected test case from an array of Strings into //the 2D array of booleans used in Assignment 2. public static boolean[][] getTest(int index){ String[] board = testBoards[index]; boolean[][] result = new boolean[board.length][board[0].length()]; for(int r=0; r ---------------------------- LifeWindow? import javax.swing.*; import java.awt.*; import java.awt.event.*; //This is needed for the mouse and key events public class LifeWindow extends JFrame { //JFrame means "window", in effect //Constants to control everything final static int DEFAULT_WINDOW_WIDTH = 800; final static int DEFAULT_WINDOW_HEIGHT = 1000; final static int TEXT_AREA_SIZE = 200; //Pixels at the bottom to draw text in final static int MARGIN = 20; //Margin around the edges of the board in the top half final static Color TOP_COLOUR = new Color(192,192,192); //Light Grey final static Color BOARD_COLOUR = new Color(240,240,170); //Light yellow final static Color TEXT_AREA_COLOUR = new Color(255,255,255); //White final static int TEXT_LINE_SPACING = 32; //Distance from one line to the next, in pixels final static int TEXT_MARGIN = 16; //Left margin for the text at the bottom final static int INSET = 2; //Small inset (in pixels) all around the circle drawn in a cell final static int SPEED = 200; //Time between generations when on "play" (in msecs) final static int SMALL = 10; //The size of board generated if the user selects "small" final static int MEDIUM = 20; //and medium final static int LARGE = 40; //and large private LifePanel wholeWindow; //A JPanel is the content within a window private int width, height; //For convenience. The drawing area, in pixels. private int squareSize; //The size of each square, in pixels private int lifeRows,lifeCols; //The size of the life board, in squares (cells) private LifeBoard theBoard; //The board itself. This class must be defined properly. private boolean running; //True if playing, false if paused. //These objects allow the simulation to run automatically at the indicated SPEED private ActionListener doOneGeneration; private Timer myTimer; //Constructor public LifeWindow(int rows, int cols) { setTitle("Game of Life"); setSize(DEFAULT_WINDOW_WIDTH,DEFAULT_WINDOW_HEIGHT); lifeRows = rows; lifeCols = cols; theBoard = new LifeBoard(randomBooleanArray(rows,cols)); //Everything is now set up by creating and using a whole lot of Objects wholeWindow = new LifePanel(this); //LifePanel is defined below add(wholeWindow); wholeWindow.addMouseListener(new HandleMouse()); addKeyListener(new HandleKeys()); setFocusable(true); requestFocusInWindow(); setVisible(true); running=false; doOneGeneration = new ActionListener(){ public void actionPerformed(ActionEvent event) { theBoard.nextGeneration(); repaint(); }; }; myTimer = new Timer(SPEED,doOneGeneration); }//SampleGraphicsWindow constructor public static void main(String[] args){ //Simple main program for testing. Just create a window. LifeWindow test = new LifeWindow(10,15); }//main private class LifePanel extends JPanel { private LifeWindow myWindow; public LifePanel(LifeWindow window){ myWindow = window; } public void paintComponent(Graphics g){ /* This is where all the drawing commands will go. * Whenever the window needs to be drawn, or redrawn, * this method will automatically be called. */ //Set all of the size variables to their current values, //which may change as a result of window resizing setSizes(g); int divider = height-TEXT_AREA_SIZE; g.setColor(TEXT_AREA_COLOUR); g.fillRect(0,0,width-1,height-1); //Draw the whole window the text area colour g.setColor(TOP_COLOUR); g.fillRect(0,0,width,divider); //Redraw the top half in its colour g.setColor(BOARD_COLOUR); g.fillRect(MARGIN,MARGIN,lifeCols*squareSize,lifeRows*squareSize); //Then the board //Draw a dividing line g.setColor(Color.BLACK); g.drawLine(0,divider,width-1,divider); //Draw the instructions at the bottom g.setFont(new Font(Font.SANS_SERIF,Font.PLAIN,24)); g.drawString("Click on a cell to toggle it, or press a key:", TEXT_MARGIN, divider+TEXT_LINE_SPACING); g.drawString("P:play/pause G:next generation R:randomize", TEXT_MARGIN, divider+2*TEXT_LINE_SPACING); g.drawString("Test boards - 1 to "+LifeTestCases.numTests(), TEXT_MARGIN, divider+3*TEXT_LINE_SPACING); g.drawString("Blank boards - S:small M:medium L:large", TEXT_MARGIN, divider+4*TEXT_LINE_SPACING); //Draw a grid for the board for(int r=0; r= MARGIN+lifeCols*squareSize) return -1; else return (xClick-MARGIN)/squareSize; } private int getRow(int yClick){ //Convert the y coordinate of a mouse click into a row number if(yClick else if(typed=='s'){ setNewBoard(SMALL); } else if(typed=='m'){ setNewBoard(MEDIUM); } else if(typed=='l'){ setNewBoard(LARGE); } }//keyTyped private void setNewBoard(int size){ theBoard = new LifeBoard(size,size); lifeRows = size; lifeCols = size; repaint(); } private void setNewBoard(boolean[][] newState){ theBoard = new LifeBoard(newState); lifeRows = newState.length; lifeCols = newState[0].length; repaint(); } }//private inner class HandleKeys }//class LifeWindow
Step by Step Solution
There are 3 Steps involved in it
Step: 1
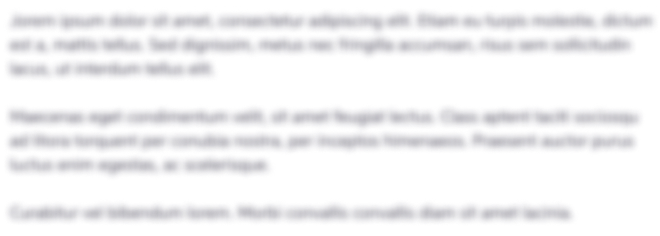
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started