Question
LINKEDLIST3: public class LinkedList3 { private class Node { private T data; private Node link; //reference public Node() { data = null; link = null;
LINKEDLIST3: public class LinkedList3 { private class Node { private T data; private Node link; //reference public Node() { data = null; link = null; } public Node (T newData, Node linkValue) { data = newData; link = linkValue; } } private Node head; public LinkedList3() { head = null; } public void addToStart(T itemData) { head = new Node(itemData,head); } public boolean deleteHeadNode() { if(head != null) { head = head.link; return true; } else return false; } public int size() { int count = 0; Node position = head; while(position != null) { count++; position = position.link; } return count; } public boolean contains(T item) { return (find(item) != null); } private Node find(T target) { Node position = head; T itemAtPosition; while(position != null) { itemAtPosition = position.data; if(itemAtPosition.equals(target)) return position; position = position.link; } return null; } public void outputList() { Node position = head; while(position != null) { System.out.println(position.data); position = position.link; } } public boolean isEmpty() { return (head == null); } public void clear() { head = null; }
public boolean equals(Object otherObject) { if(otherObject == null) return false; else if(getClass() != otherObject.getClass()) return false; else { LinkedList3 otherList = (LinkedList3)otherObject; if (size()!= otherList.size()) return false; Node position = head; Node otherPosition = otherList.head; while(position != null) { if(!(position.data.equals(otherPosition.data))) return false; position = position.link; otherPosition = otherPosition.link; } return true; } } }
EXAMPLE OF GENERICLinkedlistdemo: public class GenericLinkedListDemo { public static void main(String[] args) { // TODO Auto-generated method stub LinkedList3
(1) Please create a generic linked list with node.You can use LinkedList3 for this project. 2) Please create a Student class by yourself: (a) private instance variables: String first name, String last name, String student ID, Birthday (date, month, year) ( this could be a class: Date) (b) constructor with parameters (c) toString() function to return the data (3) Please create a driver class: a. Create an empty linked list first with Student type. b. Create 4 student objects with name, student ID and birthrate. c. Add each student to the linked list by calling the method: addToStart() d. Print the whole list by calling the method: outputList() e. Print the size of the list by calling the method: size()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
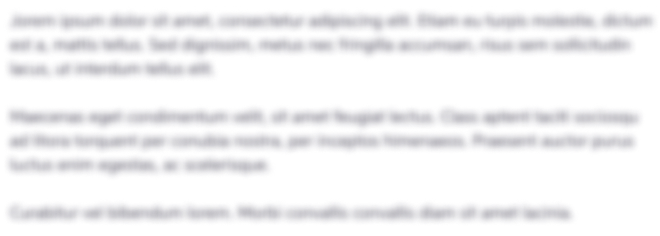
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started