Answered step by step
Verified Expert Solution
Question
1 Approved Answer
list.c #include list.h void add(LIST **head, LIST **tail, void *data) { // TODO implement the function } void clearIteratively(LIST **head, LIST **tail) { if (*head
list.c
#include "list.h"
void add(LIST **head, LIST **tail, void *data)
{
// TODO implement the function
}
void clearIteratively(LIST **head, LIST **tail)
{
if (*head == NULL)
return;
LIST *currNode = *head;
LIST *nextNode = NULL;
do
{
nextNode = currNode->next;
if (currNode->data != NULL)
free(currNode->data);
free(currNode);
currNode = nextNode;
} while (currNode != NULL);
*head = NULL;
*tail = NULL;
}
void clearRecursively(LIST **currNode, LIST **tail)
{
// TODO implement the function
}
void delete(LIST **head, LIST **tail, void *data)
{
// TODO implement the function
}
person.c
#include "person.h"
LIST *head = NULL, *tail = NULL;
void inputPersonalData(PERSON *person)
{
// TODO implement the function
// or check out part 9 of the c tutorial ;)
}
void addPersonalDataToDatabase(PERSON *person)
{
// TODO Implement the function
}
void displayDatabase()
{
// TODO Implement the function
}
void displayPerson(PERSON *person)
{
// TODO Implement the function
// hmmmm seems familiar....
}
PERSON *findPersonInDatabase(char *name)
{
// TODO Implement the function
return NULL; // if not found
}
void removePersonFromDatabase(char *name)
{
// TODO Implement the function
}
void clearDatabase()
{
// TODO Implement the function
}
person.h
#ifndef PERSON_H_
#define PERSON_H_
#include
#include
#include
#include "list.h"
typedef char NAME[41];
typedef struct date
{
int month;
int day;
int year;
} DATE;
typedef struct person
{
NAME name;
int age;
float height;
DATE bday;
} PERSON;
void inputPersonalData(PERSON *person);
void addPersonalDataToDatabase(PERSON *person);
void displayDatabase();
void clearDatabase();
void displayPerson(PERSON *person);
PERSON *findPersonInDatabase(char *name);
void removePersonFromDatabase(char *name);
#endif /* PERSON_H_ */
#include
#include
#include
#include "person.h"
#define DEF_NUM 1
#include
#include
#include
#include "person.h"
#define DEF_NUM 1
int main(void)
{
// TODO replace stdin with the file input from ../in.txt
PERSON *person;
int num;
puts("Enter the initial number of records:");
if (scanf("%d", &num)
num = DEF_NUM;
printf(" --> Reading Personnel Records... ");
while (num-- > 0)
{
person = (PERSON *) malloc(sizeof(PERSON));
inputPersonalData(person);
addPersonalDataToDatabase(person);
}
printf(" --> Displaying Database... ");
displayDatabase();
puts(" --> Searching database for Maya... ");
PERSON *maya = findPersonInDatabase("Maya");
if (maya == NULL)
puts(" Maya not found... ");
else
{
displayPerson(maya);
puts(" --> Removing Maya from database... ");
removePersonFromDatabase("Maya");
}
displayDatabase();
// added to test finding and removing the last element
puts(" --> Searching database for Frank... ");
PERSON *frank = findPersonInDatabase("Frank");
if (frank == NULL)
puts(" Frank not found... ");
else
{
displayPerson(frank);
puts(" --> Removing Frank from database");
removePersonFromDatabase("Frank");
}
displayDatabase();
puts(" --> Removing Miro from database... ");
removePersonFromDatabase("Miro");
displayDatabase();
puts(" --> Adding new record to database... ");
person = (PERSON *) malloc(sizeof(PERSON));
inputPersonalData(person);
addPersonalDataToDatabase(person);
displayDatabase();
puts(" --> Clearing database... ");
clearDatabase();
displayDatabase();
puts(" --> Adding new record to database... ");
person = (PERSON *) malloc(sizeof(PERSON));
inputPersonalData(person);
addPersonalDataToDatabase(person);
displayDatabase();
clearDatabase();
}




C PROGRAM HELP
create an application which:
reads a specified number of personnel records from the standard input.
creates a database (in linked list form) of the data.
implemenets utilities to add to, delete from, search, display and clear the database.
Linked Lists
The skeleton of a linked list implementation is provided in list.h and list.c. Check out the struct definition of a LIST node in list.h to get an idea how data will be stored.
Your task is to complete and test this implementation. This will require you to fill out the definitions for the following functions in list.c:
void add(LIST **head, LIST **tail, void *data);
void clearRecursively(LIST **currNode, LIST **tail);
void delete(LIST **head, LIST **tail, void *data);
You will also need to make an additional main function to test the LIST utilities.
add :
The goal of the add function is to create a new linked list node, populate it with the data provided in the void *data, and append it to the linked list with head (i.e. the start of the list) referenced by LIST **head and tail (i.e. the end of the list) referenced by LIST **tail.
The following cases must be handled:
The list is empty (so head and tail are NULL).
The list has at least one element (so neiter head nor tail is NULL).
delete :
The goal of the delete function is to free the node containing the specified data pointer (and its contents), and then remove said node from the list.
The following cases must be handled:
The list is empty.
The list is not empty, but the specified data is not in the list.
The specified data is in the list.
The node being deleted is the head.
It is the only node (there is only 1 node).
It is not the only node (there are multiple nodes).
The node being deleted is neither the nead nor the tail.
The node being deleted is the tail (but you don't need to worry about it being the head too, because that case was handled above).
You may wish to draw pictures of each of the cases above to determine what needs to be done in each case.
Note that whenever the list is empty, the head and tail should both be NULL; otherwise, the head is the start of the list and the tail is the end of the list.
clearRecursively:
The goal of this function is to free every node in the list (and its content) and set the head and tail to NULL.
This is the recursive version of clearIteratively, which is provided.
Personnel Database
Once the linked list utilities are complete and tested, you are ready to move on to person.c, which contains skeletons for the following functions:
void inputPersonalData(PERSON *person);
void addPersonalDataToDatabase(PERSON *person);
void displayDatabase();
void displayPerson(PERSON *person);
PERSON *findPersonInDatabase(char *name);
void removePersonFromDatabase(char *name);
void clearDatabase();
These functions should work as follows:
inputPersonalData:
takes as input a pointer to allocated space for a PERSON.
populates the space referenced by that pointer with data gotten from stdin (which you've overwritten in the main with freopen to stream from ../in.txt.
addPersonalDataToDatabase:
takes as input a pointer to a populated instance of the PERSON struct.
adds this pointer to the linked list referenced by the LIST *s head and tail in person.c.
displayDatabase:
goes through the linked list referenced by head and tail, calling displayPerson on each data pointer in the list.
note that this will require casting each list node's data field as a PERSON * (it is stored as a generic void *).
displayPerson
takes as input a PERSON *.
displays the referenced data.
findPersonInDatabase:
takes as input a char *, the name of the person to be deleted.
finds the first occurence of a PERSON * stored in the linked list whose referenced name is the same as the input name and returns that PERSON *.
if no such person is in the database, returns NULL.
deletePersonFromDatabase:
takes as input a char *, the name of the person to be deleted.
finds the first person in the list with that name (if one exists) and deletes them.
clearDatabase:
deletes the linked list referenced by head and tail.
1-liner, use a utility defined in list.c.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
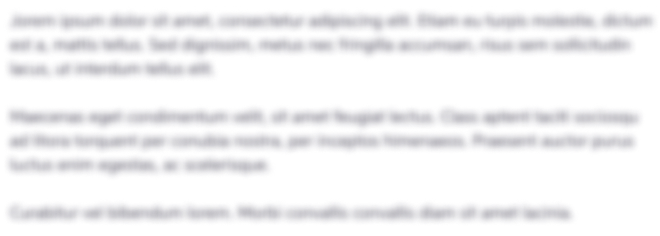
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started