Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Make a class ComputerTrainer that is a subclass of PokemonTrainer. It should do everything PokemonTrainer does, except this class will be able to populate itself
- Make a class ComputerTrainer that is a subclass of PokemonTrainer. It should do everything PokemonTrainer does, except this class will be able to populate itself with randomly generated Pokemon, and choose Moves randomly instead of having to get input from the user. You will need the below classes for the final code, but ONLY edit the ones required. DO NOT CREATE NEW CLASS DO NOT CREATE NEW CLASS
DO NOT CREATE NEW CLASSÂ
Finish ComputerTrainer.java, and EDIT PokemonTrainer.java to battle the user against the newly created ComputerTrainer.
ComputerTrainer.java EDIT THIS CLASS BY THE SPECIFICATIONS
We've gotten the class started for you. Your job is to implement the following methods: public class ComputerTrainer extends PokemonTrainer { // private constants // Possible pokemon names and move names to generate random Pokemon private static final String[] POKEMON_NAMES = {"Pikachu", "Bulbasaur", "Charmander", "Squirtle"}; private static final String[] MOVE_NAMES = {"Tailwhip", "Bodyslam", "Splash", "Shock"}; private static final int MAX_DAMAGE = 25; private static final int MAX_MOVES = 4; private PokemonImages images = new PokemonImages(); // Write the ComputerTrainer class here! // Write the Constructor that sets the name of the ComputerTrainer // and adds 2 randomly generated Pokemon to itself public ComputerTrainer(String name) { // fill this in } /* * Adds a randomly generated Pokemon to this ComputerTrainer's * collection of Pokemon. A ComputerTrainer can only have 2 * Pokemon. This method returns true if there was room for the * new Pokemon and it was successfully added, false otherwise. */ public boolean addRandomPokemon() { // fill this in } // Returns a Move randomly chosen from the set of Moves // that this trainer's current Pokemon knows. // If all Pokemon have fainted, returns null. public Move chooseRandomMove() { Pokemon currentBattlingPokemon = getNextPokemon(); // fill this in } }
PokemonSimulator.java MODIFY THIS PROGRAM SO IT BATTLES A USER VS THE COMPUTER OPPONENT
public class PokemonSimulation extends ConsoleProgram { private PokemonImages images = new PokemonImages(); public void run() { System.out.println("Welcome to Pokemon Simulator!"); System.out.println("Set up first Pokemon Trainer: "); String name = readLine("Trainer, what is your name? "); System.out.println("Hello " + name + "!"); System.out.println(); PokemonTrainer trainer1 = new PokemonTrainer(name); trainerSetUp(trainer1); System.out.println(); System.out.println("Set up second Pokemon Trainer: "); String name2 = readLine("Trainer 2, what is your name? "); System.out.println("Hello " + name2 + "!"); System.out.println(); PokemonTrainer trainer2 = new PokemonTrainer(name2); trainerSetUp(trainer2); } private void trainerSetUp(PokemonTrainer t) { boolean addNewPokemon = true; int pokeNum = 2; for (int i = 0; i < pokeNum; i++) { if (i == 0) { readLine("Choose your first pokemon: "); String pokeName = readLine("Enter the name of your pokemon: "); Pokemon p = new Pokemon(pokeName); String pokeImage = images.getPokemonImage(pokeName); System.out.println("You chose: " + pokeImage); System.out.println(pokeName + " (Health: 100/100)"); int maxMoves = 4; for (int y = 0; y < maxMoves; y++) { System.out.println(); String addMove = readLine("Would you like to teach " + pokeName + " a new move? (yes/no) "); if (addMove.equals("yes")) { String moveName = readLine("Enter the name of the move: "); String damage = readLine("How much damage does this move do? (Max damage = 25) "); System.out.println(pokeName + " learned " + moveName + " (" + damage + " Damage)!"); } else if (addMove.equals("no")) { y = maxMoves; } } System.out.println(pokeName + " has learned all of their moves"); } else { readLine("Choose your second pokemon: "); String pokeName = readLine("Enter the name of your pokemon: "); Pokemon p = new Pokemon(pokeName); String pokeImage = images.getPokemonImage(pokeName); System.out.println("You chose: " + pokeImage); System.out.println(pokeName + " (Health: 100/100)"); int maxMoves = 4; for (int m = 0; m < maxMoves; m++) { System.out.println(); String addMove = readLine("Would you like to teach " + pokeName + " a new move? (yes/no) "); if (addMove.equals("yes")) { String moveName = readLine("Enter the name of the move: "); String damage = readLine("How much damage does this move do? (Max damage = 25) "); System.out.println(pokeName + " learned " + moveName + " (" + damage + " Damage)!"); } else if (addMove.equals("no")) { m = maxMoves; System.out.println(pokeName + " has learned all of their moves"); } } } } } }
DO NOT MODIFY ANY OF THE BELOW CLASSES, DO NOT MAKE ANY EXTRA CLASSES. ONLY EDIT COMPUTERTRAINER AND POKEMONSIMULATION.
Move.java
public class Move { private static final int MAX_DAMAGE = 25; private String name; private int damage; public Move(String name, int damage) { this.name = name; this.damage = Math.min(damage, MAX_DAMAGE); } public String getName() { return name; } public int getDamage() { return damage; } public String toString() { return getName() + " (" + getDamage() + " Damage)"; } }
Pokemon.java
import java.util.ArrayList; public class Pokemon { /** * instance variables */ String pokemonName; int health; ArrayList moves = new ArrayList(); /** * stores image */ String image; /** * Sets pokemon name and health */ Pokemon(String name) { this.pokemonName = name; this.health = 100; } /** * constructor with image */ Pokemon(String name, String image) { this(name); this.image = image; } /** * Getter for the image * @return image */ public String getImage() { return image; } /** * setter for image */ public void setImage(String image) { this.image = image; } void addMoves(Move other) { moves.add(other); System.out.println("Move Added to collection"); } public String getName() { return this.pokemonName; } public int getHealth() { return this.health; } public boolean hasFainted() { if(this.health <= 0) { return true; } else return false; } public boolean canLearnMoreMoves() { if(this.moves.size() < 4) { return true; } else return false; } public boolean learnMove(Move move) { if(this.canLearnMoreMoves()) { addMoves(move); return true; } else return false; } /** * Remove the Move `move` from this Pokemon's * collection of Moves, if it's there. * @param Move move */ public void forgetMove(Move move) { if (this.moves.remove(move)) System.out.println("Move removed "); } /** * Return a String containing the name and health * of this Pokemon * Also returns the image if it exists * Example: "Pikachu (Health: 85 / 100)" * @return String name + health + image(if exists) of pokemon */ public String toString() { return ((this.image != null)?this.image:"") + this.pokemonName + "(Health:" + this.health + "/100)"; } /** * Returns an ArrayList of all the Moves this Pokemon knows */ public ArrayList getMoves() { return this.moves; } public boolean knowsMove(Move move) { return this.moves.contains(move); } public boolean knowsMove(String moveName) { for (Move move: moves) { if (move.getName().equalsIgnoreCase(moveName)) return true; } return false; } public boolean attack(Pokemon opponent, Move move) { if (knowsMove(move)) { move = moves.get(moves.indexOf(move)); opponent.health -= move.getDamage(); return true; } return false; } public boolean attack(Pokemon opponent, String moveName) { for (Move move: moves) { if (move.getName().equalsIgnoreCase(moveName)) return attack(opponent, move); } return false; } }
PokemonTrainer.java
import java.util.ArrayList; public class PokemonTrainer { // private constants private static final int MAX_POKEMON = 2; // attributes private String name; private ArrayList pokemons = new ArrayList(); public PokemonTrainer(String name) { this.name = name; } /** * Adds Pokemon p to the PokemonTrainer's collection of Pokemon. * a player is only allowed MAX_POKEMON pokemons, so it returns true if a pokemon * has been added and false otherwise * @return true if a pokemon has been succesfully added * @return false if a pokemon cannot be added */ public boolean addPokemon(Pokemon p) { if (pokemons.size() < MAX_POKEMON) { pokemons.add(p); return true; } return false; } /** * Returns true if all of the PokemonTrainer's Pokemon * have fainted, false otherwise. * @return true if all pokemon of a trainer has fainted * @return false if all pokemon of a trainer haven't fainted */ public boolean hasLost() { for (Pokemon pokemon : pokemons) if (!pokemon.hasFainted()) return false; return true; } /** * Returns the first Pokemon that has not yet fainted * from this PokemonTrainer's collection of Pokemon. * If every Pokemon has fainted, this method returns null. * @return first pokemon that hasn't fainted in collection * @return null if all pokemon have fainted */ public Pokemon getNextPokemon() { for (Pokemon pokemon : pokemons) if (!pokemon.hasFainted()) return pokemon; return null; } public String toString() { return this.name; } }
PokemonImages.java
import java.util.*; import java.io.*; // This is the PokemonImages class // It acts as a dictionary to get ASCII art for a given pokemon name // Feel free to explore this code, it uses FileReader and BufferedReader // to read in Pokemon images from the "pokemonImages.txt" file. // DO NOT CHANGE THIS CODE, the program may not work if you do. public class PokemonImages { private HashMap pokedex; private static final String IMAGE_FILE = "pokemonImages.txt"; public PokemonImages() { pokedex = new HashMap(); loadImages(IMAGE_FILE); } public String getPokemonImage(String name) { return pokedex.get(name.toLowerCase()); } public String getPokemonImage(Pokemon pokemon) { String name = pokemon.getName().toLowerCase(); return pokedex.get(name); } public void setPokemonImage(String pokemonName, String image) { if(pokemonName != null && image != null) { pokedex.put(pokemonName, image); } } private void loadImages(String filename) { // Try to do the following code, but there may be errors // when reading the file. try { // Create the BufferedReader to read from the file where the // ASCII Art is stored BufferedReader input = new BufferedReader(new FileReader(filename)); String line = input.readLine(); String currentImage = ""; String currentPokemon = null; // Read each line of the file and add each pokemon name and image // to the HashMap while(line != null) { // Comment line, we can skip it if(line.startsWith("$$")) { line = input.readLine(); continue; } // The "##" indicates a new pokemon name // Store the old pokemon if there was one and start a new pokemon else if(line.startsWith("##")) { if(currentPokemon != null) { pokedex.put(currentPokemon, currentImage); } // Get the name of the new pokemon currentPokemon = line.substring(2).toLowerCase(); currentImage = ""; } else { currentImage += line + " "; } line = input.readLine(); } // Fencepost situation // The last pokemon has not been added yet, add it to the HashMap if(currentPokemon != null) { pokedex.put(currentPokemon, currentImage); } input.close(); } // If there were any errors when reading the file // they will be handled by these `catch` clauses catch (FileNotFoundException e) { System.out.println("Couldn't open file: " + filename); } catch (IOException e) { System.out.println("There was an error while reading the file: " + filename); e.printStackTrace(); } } }
PokemonImages.txt
$$ This file holds ASCII Images for a couple Pokemon $$ $$ Image courtesy of http://pokemon.wikia.com/wiki/Bulbasaur $$ Converted to ASCII Art with http://manytools.org/hacker-tools/convert-images-to-ascii-art/ ##Bulbasaur ((( .(((/(((((((((((((( ((((( ((((((((((((((( (( /(((((( ((((((((((((((( (( ((((((((((((((/### .(( ./((/. (#### .((((((((( (((((*################# *#### ((((((((((((*((( (##############.,,, ##### ( (((((((((((//((((##############*,, ####### (/(((((((((((((( .((######### ##, ##### ### # /(,(((((((((((((( ((#### @,@ #########,, ##@,@# //((((((((((((( ## ((### @ ,@, #######( ,,#/@, /// ((((((((( ,,.#######@@ ,@,, ############,,, * ///,*//// ,,,,, #######@@ ,,,,.############ (### ,//// # ,,,,.################################ ##################. (#############. ## ######### ####### #################### # ,. *## #########((( ########. .#### #.,,,,(###### .,####*(((((((((((((((###### ###( (### ##,,,,#####(((((((((((((((#### .# (######## (##,,.####(((((((((((( (((###.,,# ((######*(### #### (((((( ,((####/ # *(((((((( (#######*((####, .((######% &@,& /@ ( #/# , ((,@# $$ Image courtesy of http://pokemon.wikia.com/wiki/Charmander $$ Converted to ASCII Art using http://manytools.org/hacker-tools/convert-images-to-ascii-art/ ##Charmander ,%%(((((,. ,#(((((((((((. ,/((((((((/##.(((((* //(((((((((...../((((( .,,*.(((((**..*#((((((( .,,,,,.(((((((((((((( # %.,,,./,(((((((((((* && , ..###/*((((((((((% &&&&&( ,((((%.,,##/.(((((((((((/ *,. %&&/#( .(((((///(((((///((((**%%#(((((, &&&&//// /((((((((*(/(((((((((((((((((, (&////// .(((((/&&&&%/(((((((((((((. ##*(*/& .((,&&&&&&&,(((((((//, (, *&&&&&&&&&/((((//(/ (, %&&&&&&&&&*(((((((( *(* ,&&&&&&&&&((((((((. ,((( *&&&&&&&&&&(((((((((, ,((((% .%%((,&&&&&&&&&&,((((((((* .,(((((%, .%(((((*&&&&&&&&,(((((((((*/((((((,%* /(*,, ,(((////*&&&&((((((((((/,//((*(%* #*((((((///////(%%%/((((((((((/,*%%%%. */////////,. /////(((((///*%%(. .///// /////////% .. ./////, /,,/,/,, %,%#,,. $$ Image courtesy of http://pokemon.wikia.com/wiki/Squirtle $$ Converted to ASCII Art using http://manytools.org/hacker-tools/convert-images-to-ascii-art/ ##Squirtle @@(*******@@ @@**************@ @******/ &*********@ @******% %%/*******@*@ @ @******@%@%@*********, @**&@ @******/&%@@********@% &***@*@*@&****/*************@@@ @//*@*****/**********@********@ @@*****%@ @///******@*****************@ @**********& @(@///*******%*************@ @************@ @(((&////****@,,,*/@@((&@/***@ //************@#((((&////**@,,,,,,,,,,,@********@ ///*****@*****##(((( (//,&,,,,@,,,,,,,,********* @///////@****@#@((( (,,,,,,,,,,,,,,,,,(/////***@ @@//#@******@##@# @,,,,,,,,,,,,,,@,,,@ @/////*****@##@,*/,,,,,,@@,,,,,,#,,,, @/////****##/****@,,,,,,,#@,,,,,,,@ @#/////*@*******%,,,,,,,,,,,,,@**@ @@(%*********@*******/**@*****@ %********/@******@@/*******@ ********/@ @////*****@@ @*********/ @////////@ /*****% %//@@ $$ Image courtesy of http://pocket-monstersbr.tumblr.com/ $$ Converted to ASCII Art using http://manytools.org/hacker-tools/convert-images-to-ascii-art/ ##Pikachu @&&&( (@&&& @@&..*& (&../&&& %&.....&. &.....#& &......#. ,&@&&&@%, %......& *&................,...,&/ ./...............&, /@/..& &..,*........#&...( /@*........% &.%&%&......(@@&..&%#..............% (........*......,.(...,.............& #(((/..,%###(..*((((*.../**,,.........& @(#....(///.....(......**********(%&@& &......*....,.......@, (.....,..,,./......**(. #.................&***(. .,....(........,(*******/, .,....*...........**% &/(./..../...........###& &*,../..,/..../.(,(...%&. #**...,,,,....(**..../ /**************/*,.& &%,**,...#@#*(*/&*
Randomizer.java
import java.util.Random; public class Randomizer{ public static Random theInstance = null; public Randomizer(){ } public static Random getInstance(){ if(theInstance == null){ theInstance = new Random(); } return theInstance; } /** * Return a random boolean value. * @return True or false value simulating a coin flip. */ public static boolean nextBoolean(){ return Randomizer.getInstance().nextBoolean(); } /** * This method simulates a weighted coin flip which will return * true with the probability passed as a parameter. * * @param probability The probability that the method returns true, a value between 0 to 1 inclusive. * @return True or false value simulating a weighted coin flip. */ public static boolean nextBoolean(double probability){ return Randomizer.nextDouble() < probability; } /** * This method returns a random integer. * @return A random integer. */ public static int nextInt(){ return Randomizer.getInstance().nextInt(); } /** * This method returns a random integer between 0 and n, exclusive. * @param n The maximum value for the range. * @return A random integer between 0 and n, exclusive. */ public static int nextInt(int n){ return Randomizer.getInstance().nextInt(n); } /** * Return a number between min and max, inclusive. * @param min The minimum integer value of the range, inclusive. * @param max The maximum integer value in the range, inclusive. * @return A random integer between min and max. */ public static int nextInt(int min, int max){ return min + Randomizer.nextInt(max - min + 1); } /** * Return a random double between 0 and 1. * @return A random double between 0 and 1. */ public static double nextDouble(){ return Randomizer.getInstance().nextDouble(); } /** * Return a random double between min and max. * @param min The minimum double value in the range. * @param max The maximum double value in the rang. * @return A random double between min and max. */ public static double nextDouble(double min, double max){ return min + (max - min) * Randomizer.nextDouble(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Heres the completed ComputerTrainer class java import javautilArrayList public class ComputerTrainer extends PokemonTrainer private constants Possible pokemon names and move names to generate random P...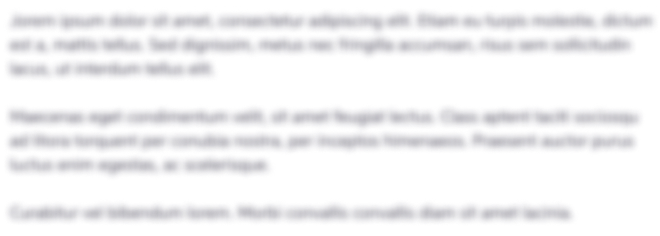
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started