Question
.Make sure it follows time complexity. The Bag ADT is an abstraction of a multiset, i.e. a set where an item can be present in
.Make sure it follows time complexity. The Bag ADT is an abstraction of a multiset, i.e. a set where an item can be present in the bag more than once. Locations of items are not relevant in a bag, so operations such as removeItemAt(int location) or retrieveItemAt(int location) are not allowed. Implement an array-based Bag as described in the UML class diagram below.
ArrayBag |
-String[] bag -int length -int SIZE |
+ArrayBag +void add(String s) +int count(String s) +boolean isEmpty() +void remove(String s) +String toString() |
Main |
+static void main(String[] args) +Main() |
Guidelines
-You are allowed to use all of the code given in the lectures (for example, the implementation of the methods of the ArrayList class).
-Classes from the Java Collection Framework,e.g. java.util.ArrayList, are not allowed for use in your implementation.
-Students are required to structure the code as indicated in the UML class diagram
-Several files accompany this project description:
assignment 1 test set.txt: a test file,
Main.java: a complete implementation of the Mainclass (tests your code using file assignment 1test set.txt),
ArrayBag.java: the framework of the ArrayBagclass, with the toString method implemented,
output.pdf: a file with the output produced by the Mainclass provided.
-Students are required to use the ArrayBag class framework included, completing all the methods as indicated in the Javadoc comment section of each method.The toString method provided is to be used in all output.
Fill in the methods for ArrayBag.java ArrayBag.java
/** * The class ArrayBag implements an array-based bag. * */ public class ArrayBag { /** * Default constructor. Initial bag capacity is 1. * */ public ArrayBag() { }
/** * Adds new element to the bag. If the array used to store the bag items is * full, it will be expanded by doubling its size. If the array is not full, * the method will take O(1) time. * * @param s new element to be added to the bag. */ public void add(String s) { }
/** * Determines the number of occurrences in the bag of a given element. * The method takes O(length) time. * * @param s given element * @return number of times the given element occurs in the bag. */ public int count(String s) { }
/** * Determines if bag is empty. The method takes O(1) time. * * @return true if bag contain no elements, false otherwise. */ public boolean isEmpty() { }
/** * Removes all occurrences of a given element. The method will take O(1) time * in removing each occurrence of the given element. * * @param s element to be removed. */ public void remove(String s) { }
/** * Constructs a String description of the bag. * * @return String containing the bag elements. */ public String toString() { String str = "";
for (int i=0; i if (length != 0) return "Bag: {" + str + bag[length-1] + "}"; else return "Bag: {" + str + "}"; } private String[] bag; //array that stores the bag elements private int length; //number of elements in the bag private int SIZE; //bag capacity } Main.java public class Main { public static void main(String[] args) { new Main(); } public Main() { ArrayBag bag = new ArrayBag(); File file = new File("assignment 1 test set.txt"); try { Scanner in = new Scanner(file); String operation = "", item = ""; int entryNumber = 0; while (in.hasNextLine()) { entryNumber++; operation = in.next(); if (!operation.equals("IS_EMPTY")) { item = in.next(); System.out.println(" " + operation + " " + item); } else System.out.println(" " + operation); switch(operation) { case "ADD": bag.add(item); System.out.println(bag); break; case "COUNT": System.out.println("Number of \"" + item + "\": " + bag.count(item)); break; case "IS_EMPTY": System.out.println(bag.isEmpty()); break; case "REMOVE": bag.remove(item); System.out.println(bag); break; default: System.out.println("Operation \"" + operation + "\" unknown at line " + entryNumber); System.exit(1); } } } catch(FileNotFoundException e) { System.out.println("File not found!"); System.exit(1); } } } Assignment 1 test set IS_EMPTY ADD Cabbage ADD Cucumber ADD Broccoli COUNT Cucumber ADD Banana ADD Broccoli ADD Guava REMOVE Cucumber REMOVE Cucumber REMOVE Cabbage ADD Guava REMOVE Coconut ADD Guava COUNT Guava REMOVE Banana REMOVE Guava ADD Orange COUNT Banana REMOVE Orange ADD Broccoli IS_EMPTY REMOVE Broccoli IS_EMPTY REMOVE Broccoli
Step by Step Solution
There are 3 Steps involved in it
Step: 1
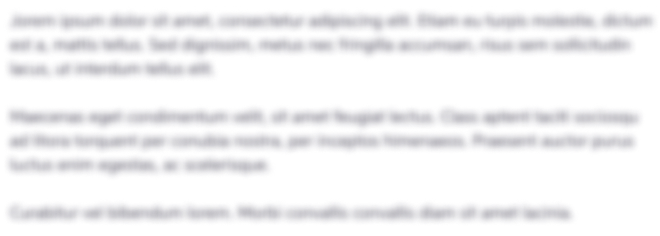
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started