Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Make sure to avoid memory leaks. Use the following main file: #include #include /** * If you don't have a typedef on your data structure
Make sure to avoid memory leaks. Use the following main file:
#include#include /** * If you don't have a typedef on your data structure then you can use * "struct set" everywhere. */ int main() { set s1, s2; //--- Initializing sets ---// init(&s1); init(&s2); //--- Inserting elements ---// insert(&s1, 1); insert(&s1, 2); insert(&s1, 3); insert(&s1, 2); insert(&s1, 3); insert(&s1, 3); insert(&s2, 2); insert(&s2, 3); insert(&s2, 4); insert(&s2, 5); //--- Checking set elements ---// if (s1.size != 3) printf("The size of s1 should be 3. "); if (s2.size != 4) printf("The size of s2 should be 4. "); //--- Computing intersection ---// set* s = set_intersection(&s1, &s2); if (s->size != 2 || !contains(s, 2) || !contains(s, 3)) printf("The intersection should be 2, 3. "); dest(s); free(s); //--- Computing union ---// s = set_union(&s1, &s2); if (s->size != 5 || !contains(s, 1) || !contains(s, 2) || !contains(s, 3) || !contains(s, 4) || !contains(s, 5)) printf("The intersection should be 1, 2, 3, 4, 5. "); dest(s); free(s); //--- Destructing original sets ---// dest(&s1); dest(&s2); }
Read from file (3 points)
Modify the sample program above so it reads the integers from files:
numbers1.txt ------------ 1 2 3 2 3 3 numbers2.txt ------------ 2 3 4 5
Command line arguments (2 points)
Read the file names from command line arguments that contain the integers:
$ ./a.out numbers1.txt numbers2.txt
Modularity (3 points)
Separate the program to translation units. Function definitions should go to separate translation units to which a header file belongs. Don't forget header guards.
Set data structure In this task you need to create a set data structure. This set contains integers, but all integer is stored only once. For example if you insert 1, 3, 2,3,5,5, 3 then the set contains 1, 23, 5 by the end. Set implementation (7 points) Create a struct named set. This should have two members: items :A pointer to an array allocated in heap memory. This contains the elements of the set. You may assume that the set will never contain more than 100 elements. You can hard-code this number in a macro token but that results 2 less points in the grading. size: Current size of the set. Implement the following operations: init(): This gets a pointer to a set and sets its size to 0 and items to NULL pointer (if you're not using a fixed buffer size). dest(): This function gets a pointer to a set and destructs it, i.e. deallocates the array of elements from the heap. If you're using fixed buffer size then this function does nothing. contains(): This function gets a pointer to a set and an integer. The function returns true if the integer is among the elements, false otherwise. (You can also use 1 and 0 for representing boolean values.) insert(): This function gets a pointer to a set and an integer. The function inserts the integer to the set if it is not there yet. If you're not using fixed buffer size then the array size is incremented by one in the heap and the new element is inserted there. Don't forget to increment the size attribute as well. set_union(): This function gets two pointers to set s and returns another pointer to a set that contains the union of first two. The resulting set should be created in the heap memory. set_intersection() : (Leave this to the end. Start implementing this operation if you're done with everything else.) This function gets two pointers to set s and returns another pointer to a set that contains the intersection of first two. The resulting set should be created in the heap memoryStep by Step Solution
There are 3 Steps involved in it
Step: 1
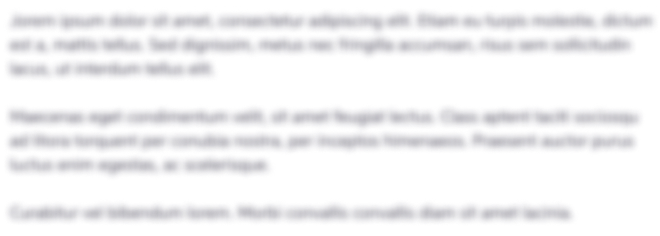
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started