Question
Modify Project 1 (knuts.c) (Write a C program that converts knuts to sickles and galleons (the currency of the Harry Potter novels). The user will
Modify Project 1 (knuts.c) (Write a C program that converts knuts to sickles and galleons (the currency of the Harry Potter novels). The user will enter the total number of knuts. There are 29 knuts in one sickle and 17 sickles in one galleon.) So it includes the following function: void convert(int total_knuts, int *galleons, int *sickles, int *knuts); The function determines the number of galleons, sickles, and knuts for the amount of the total number of knuts. The galleons parameter points to a variable in which the function will store the number of galleons required. The sickles and knuts parameters are similar. Modify the main function so it calls convert to compute the smallest number of galleons, sickles, and knuts. The main function should display the result. Name your program knuts2.c.
Here is the knuts program
#include
#include
#define SICKLE 29
#define GALLEON 493
//this program will take the amount of knuts and convert the knuts into galleons sickles and knuts
int main()
{
int remainder1, remainder2, s, g, knuts;//local variables
printf(" Enter the amount of knuts: ");//ask user to input desired amount of knuts
scanf("%d", &knuts);
if(knuts < 0 || knuts > 1000000000)//input must be between zero and one billion, otherwise it will display error message and exit the program
{
printf("Error");
exit(0);
}
g = knuts/GALLEON;
remainder1 = knuts%GALLEON;
s = remainder1/SICKLE;
remainder2 = remainder1%SICKLE;
printf("The amount of knuts is equal to %d galleon and %d sickle and %d knuts", g, s, remainder2);//displays total amount of galleon(s) sickle(s) and knut(s)
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
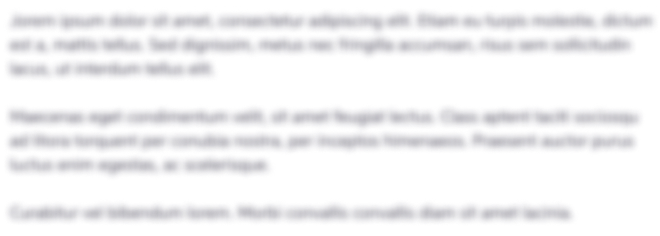
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started