Question
Modify the ArrayBag class given in Chapter 3 to meet the following specs: ? Write a member function replace that replaces a given item in
|
** Header file for an array-based implementation of the ADT bag. @file ArrayBag.h */ # ifndef _ARRAY_BAG # define _ARRAY_BAG # include "BagInterface.h" template < class ItemType > class ArrayBag: public BagInterface < ItemType > { private: static const int DEFAULT_CAPACITY = 6; // Small size to test for a full bag ItemType items [DEFAULT_CAPACITY]; // Array of bag items int itemCount; // Current count of bag items int maxItems; // Max capacity of the bag // Returns either the index of the element in the array items that // contains the given target or -1, if the array does not contain // the target. int getIndexOf (const ItemType & target) const; public: ArrayBag (); int getCurrentSize () const; bool isEmpty () const; bool add (const ItemType & newEntry); bool remove (const ItemType & anEntry); void clear (); bool contains (const ItemType & anEntry) const; int getFrequencyOf (const ItemType & anEntry) const; vector < ItemType > toVector () const; } ; // end ArrayBag # include "ArrayBag.cpp" # endif |
Listing 3-2 A program that tests the core methods of the class ArrayBag |
# include < iostream > # include < string > # include "ArrayBag.h" using namespace std; void displayBag (ArrayBag < string > & bag) { cout << "The bag contains " << bag.getCurrentSize () << " items:" << endl; vector < string > bagItems bag.toVector (); int numberOfEntries = (int) bagItems.size (); for (int i = 0 ; i < numberOfEntries ; i++) { cout << bagItems [i] << " "; } // end for cout << endl << endl; } // end displayBag void bagTester (ArrayBag < string > & bag) { cout << "isEmpty: returns " << bag.isEmpty () << "; should be 1 (true)" << endl; displayBag (bag); string items [] = {"one", "two", "three", "four", "five", "one"}; cout << "Add 6 items to the bag: " << endl; for (int i = 0 ; i < 6 ; i++) { bag.add (items [i]); } // end for displayBag (bag); cout << "isEmpty: returns " << bag.isEmpty () << "; should be 0 (false)" << endl; cout << "getCurrentSize: returns " << bag.getCurrentSize () << "; should be 6" << endl; cout << "Try to add another entry: add(\"extra\") returns " << bag.add ("extra") << endl; } // end bagTester int main () { ArrayBag < string > bag; cout << "Testing the Array-Based Bag:" << endl; cout << "The initial bag is empty." << endl; bagTester (bag); cout << "All done!" << endl; return 0; } // end main |
Modify the ArrayBag class given in Chapter 3 to meet the following specs: ? Write a member function replace that replaces a given item in a given bag with another given item. The function should return a boolean value to indicate whether the replacement was successful. Page 3 of 3 ? Write a recursive array-based implementation of the method toVector for the class ArrayBag. ? Write a client function that merges two bags into a new third bag. Do not destroy the original two bags
Step by Step Solution
There are 3 Steps involved in it
Step: 1
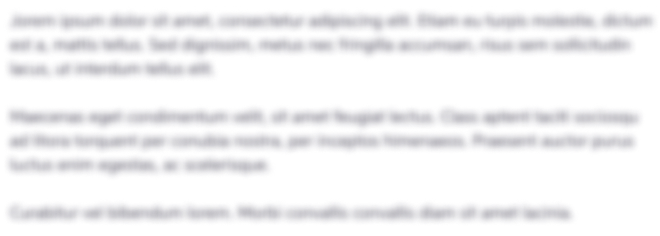
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started