Question
modify the ArrayException.java to include exception handling. The program prompts a user to input integer values into a 10-element array. It will then ask the
modify the ArrayException.java to include exception handling. The program prompts a user to input integer values into a 10-element array. It will then ask the user to input either 1) Index (0-9) and return the value stored at this index or 2) Value (int) which searches the array all occurrences of the value if found.
Modify this program to include exception handling for the following exceptions:
1) If the user enters an invalid index, display an appropriate error message. Hint: Use try and catch for the ArrayIndexOutOfBoundsException
2) If the user enters an invalid input (i.e. "Hello" instead of an integer). Hint: Use try and catch for the InputMismatchException
3) If the number entered to search the array is not found the program should throw a custom NumberNotFound exception
this is the code i have so far package arrayexception; import java.util.Scanner; /** Computer Science II Programming Assignment #2 * * @author * @since 1-28-2019 * * The following code example will prompt the user to enter 10 integers into an array * It will then ask the user to input either * 1) Index (0-9) Return the value stored at this index * 2) Value (int) Searches the array all occurrences of the value if found * * Modify this program to include exception handling for the following exceptions * 1) If the user enters an invalid index, display an appropriate error message * Use try and catch for the ArrayIndexOutOfBoundsException * * 2) If the user enters an invalid input (i.e. "Hello" instead of an integer) * Use try and catch for the InputMismatchException * * 3) EXTRA CREDIT 20%: * If the number entered to search the array is not found, generate a custom * NumberNotFound exception */ public class ArrayException { public static void main(String[]args) { Scanner input = new Scanner(System.in); // create an array to search int[] array = new int[10]; int response; int index; int value; // Prompt the user to input 10 integers and store them in the array System.out.println("Enter 10 integers"); // Fill the array with user input. for (int i = 0; i < array.length; i++) { System.out.printf("Enter an integer for index# %d: ", i); array[i] = input.nextInt(); } // Prompt the user to choose between searching by value or searching by index. System.out.printf("%n1)Search by index 2)Search by value "); response = input.nextInt(); //Search by index if (response == 1) { //user inputs index System.out.printf("Enter an Index: "); index = input.nextInt(); //Output the value stored at that index System.out.printf("Integer at index %d: %d", index, array[index]); } //Search by value if (response == 2) { //user inputs value System.out.printf("Enter a value: "); value = input.nextInt(); //for loop searches for value in the array for (int i = 0; i < array.length; i++) { //If the value is found, index and value will be displayed. if (array[i] == value) { System.out.printf("%nValue %d is found at index %d", value, i); } } } input.close(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
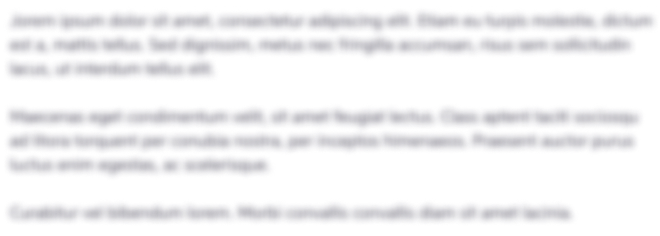
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started