Question
Modify the following Project to: 1.. Store the data in Binary file and access it in Random Access mode. //The opening logic will be provided
Modify the following Project to:
1.. Store the data in Binary file and access it in Random Access mode. //The opening logic will be provided below
2. Replace Class with Structure for Employee and Department.
3. Inside each structure, replace all string variables with array of characters.
4. Make Employee and Department editable or so that it should be able to be modified. This means, the user should be able to edit a given Employee and Department that was previously inputted. You can allow the user to edit Employee name, age etc and assign him/her to different department, similarly department name and department head can be changed. However, do not allow the user to edit Employee ID in Employee file and Department ID in department file.
5. Please note that unlike in the following project, the code will not be stored in arrays. Instead, it should be written to the file as soon as you collect the data from the user. If you are editing a record, read it from the file, collect new data from the user, store the record back to the file in the same place it was found inside the file. That means, the menu will not have options to save data to file or read data from file. Also, this should provide the ability for user to create unlimited number of employees and departments unlike in the following project where it was allowed only limited number of departments and employees.
----------------------------------------------------------------------------------------------------------------
This is for the department options:
fstream departments("departments.dat", ios::in |ios::out | ios::binary); if (departments.fail()) { departments.open("departments.dat", ios::out|ios::in | ios::binary| ios::trunc ); if (departments.fail()) { cout << "Error opening deparment file...."; return 0; } }
This is for the employee options:
fstream employees("employees.dat", ios::in |ios::out | ios::binary); if (employees.fail()) { employees.open("employees.dat", ios::out|ios::in | ios::binary| ios::trunc ); if (employees.fail()) { cout << "Error opening employee file...."; return 0; } }
----------------------------------------------------------------------------------------------------------------
This is the code that is provided:
#include #include #include using namespace std; class Departments{ string departmentID,departmentName,departmentHeadName; public: Departments(){} Departments(string ID,string name){ departmentID=""; departmentName=""; departmentHeadName=""; } Departments(string ID,string name,string head){ departmentID=ID; departmentName=name; departmentHeadName=head; } void setDepartmentID(string ID){ departmentID=ID; } void setDepartmentName(string name){ departmentName=name; } void setDepartmentHeadName(string head){ departmentHeadName=head; } string getDepartmentID(){ return departmentID; } string getDepartmentName(){ return departmentName; } string getDepartmentHeadName(){ return departmentHeadName; } };
class Employee{ string employeeID, employeeName, employeeDepartmentID; int employeeAge; double employeeSalary; public: Employee(){} Employee(string ID, string name){ employeeID=ID; employeeName=name; } Employee(string ID, string name,int age){ employeeID=ID; employeeName=name; employeeAge=age; } Employee(string ID, string name,int age, double salary){ employeeID=ID; employeeName=name; employeeAge=age; employeeSalary=salary; } Employee(string ID, string name,int age, double salary,string department){ employeeID=ID; employeeName=name; employeeAge=age; employeeSalary=salary; employeeDepartmentID=department; } void setEmployeeID(string ID){ employeeID=ID; } void setEmployeeName(string name){ employeeName=name; } void setEmployeeAge(int age){ employeeAge=age; } void setEmployeeSalary(double salary){ employeeSalary=salary; } void setEmployeeDepartment(string dept){ employeeDepartmentID=dept; }
string getEmployeeID(){ return employeeID; } string getEmployeeName(){ return employeeName; } int getEmployeeAge(){ return employeeAge; } double getEmployeeSalary(){ return employeeSalary; } string getEmployeeDepartment(){ return employeeDepartmentID; } };
int main(){ Employee e[7]; Departments d[3]; int ch=0, employeeAge, i=0, j=0; string departmentID,departmentName,departmentHeadName; string employeeID, employeeName, employeeDepartmentID; double employeeSalary;
do{ cout<<" 1. Create Department 2. Create Employee"; cout<<" 3. Write the data to the file 4. Retrieve data from file"; cout<<" 5. Display Report 6.Exit Enter your choice : "; cin>>ch; switch(ch){ case 1: if(i<3){ cout<<" Enter the Department Id : "; cin.ignore(); getline(cin,departmentID); d[i].setDepartmentID(departmentID); cout<<" Enter the Department Name : "; cin.ignore(); getline(cin,departmentName); d[i].setDepartmentName(departmentName); cout<<" Enter the Department HeadName : "; cin.ignore(); getline(cin,departmentHeadName); d[i].setDepartmentHeadName(departmentHeadName); i++; } else cout<<" The array is full, you can not add any more Departments"; break; case 2: if(j<7){ cout<<" Enter the Employee Id : "; cin.ignore(); getline(cin,employeeID); e[j].setEmployeeID(employeeID); cout<<" Enter the Employee Name : "; cin.ignore(); getline(cin,employeeName); e[j].setEmployeeName(employeeName); cout<<" Enter the Employee Department ID : "; cin.ignore(); getline(cin,employeeDepartmentID); e[j].setEmployeeDepartment(employeeDepartmentID); cout<<" Enter the Employee Age : "; cin>>employeeAge; e[j].setEmployeeAge(employeeAge); cout<<" Enter the Employee Salary : "; cin>>employeeSalary; e[j].setEmployeeSalary(employeeSalary); j++; } else cout<<" The array is full, you can not add any more Employees"; break; case 3: { ofstream myfile; myfile.open ("example.txt"); for(int k=0;k<3;k++){ myfile<<" "< myfile<<" "< myfile<<" "< } myfile.close(); break; } case 5: cout<<" Report : "; for(int k=0;k<3;k++){ cout<<" "< cout<<" "< cout<<" "< } break;
case 6: exit(1); break; default : cout<<" Invalid option"; break; } }while(ch != 6);
return 0; }
----------------------------------------------------------------------------------------------------------------
The following test cases must be identical to the final solution:
TEST CASE1: Human Resources Menu //This is what the menu should look like - word for word. 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 1 Enter the NEW Department Data: Dept ID: 1 Dept Name: Sales Head of Dept Name: Anna Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 2 Enter the NEW Employee Data: Employee ID: 1 Employee Name: John Employee Salary: $45000 Employee Age: 23 Department ID: 1 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 5 Salary Report By Department Dept : Sales // This is how the information should display Total Salary : $45000 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 2 Enter the NEW Employee Data: Employee ID: 2 Employee Name: Susan Employee Salary: $50000 Employee Age: 30 Department ID: 1 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 5 Salary Report By Department Dept : Sales Total Salary : $95000 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 1 Enter the NEW Department Data: Dept ID: 2 Dept Name: Marketing Head of Dept Name: Marcus Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 2 Enter the NEW Employee Data: Employee ID: 3 Employee Name: Adam Employee Salary: $60000 Employee Age: 30 Department ID: 2 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 5 Salary Report By Department Dept : Sales Total Salary : $95000 Dept : Marketing Total Salary : $60000 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 3 Which record to EDIT: Please choose one of the following... 1 to 2 : 2 //When modifying THIS should absolutely be incorporated. Display Department Details: Dept ID : 2 Dept Name : Marketing Dept Head : Marcus EDIT the Department Data: Dept Name: Global Marketing Head of Dept Name: Butler Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 5 Salary Report By Department Dept : Sales Total Salary : $95000 Dept : Global Marketing Total Salary : $60000 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 4 Which record to EDIT: Please choose one of the following... 1 to 3 : 3 Display Employee Details: ID : 3 Name : Adam Salary : $60000 Age : 30 Dept : 2 Edit the Employee Data: Employee Name: Adam Employee Salary: $75000 Employee Age: 31 Department ID: 1 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 5 Salary Report By Department Dept : Sales Total Salary : $170000 Dept : Global Marketing Total Salary : $0 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 9 Please enter a valid choice (1 - 6): 6 Thank you, goodbye. TEST CASE2: Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 1 Enter the NEW Department Data: Dept ID: 1 Dept Name: HumanResources Head of Dept Name: Maria Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 1 Enter the NEW Department Data: Dept ID: 1 Please enter a unique Deptartment ID: 2 Dept Name: Marketing Head of Dept Name: Samy Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 2 Enter the NEW Employee Data: Employee ID: 1 Employee Name: John Employee Salary: $45000 Employee Age: 35 Department ID: 9 Please enter an existing Deptartment ID: 1 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 2 Enter the NEW Employee Data: Employee ID: 1 Please enter a unique Employee ID: 2 Employee Name: Susan Employee Salary: $70000 Employee Age: 25 Department ID: 2 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 5 Salary Report By Department Dept : HumanResources Total Salary : $45000 Dept : Marketing Total Salary : $70000 Human Resources Menu 1. Create Department 2. Create Employee 3. Edit Department 4. Edit Employee 5. Display Salary Report 6. -- Quit -- Please make a selection : 9 Please enter a valid choice (1 - 6): 6 Thank you, goodbye.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
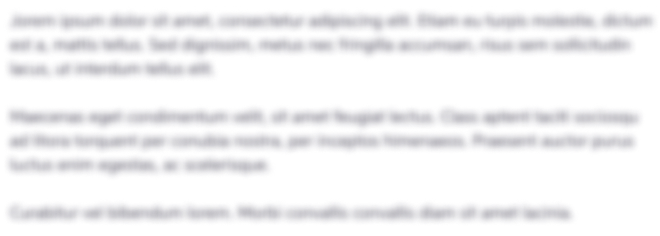
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started