Question
Modify the program where I have comment indicated write your codes in the following template functions: insertAtFront, insertAtBack, removeFromFront No need to change anything else.
Modify the program where I have comment indicated write your codes in the following template functions: insertAtFront, insertAtBack, removeFromFront No need to change anything else.
Comments sections in link below
file:///home/chronos/u-ef0046b68a3a1d5366672f3b5076bc6710d2ca06/Downloads/LinkedListLab.pdf
Full program
Page of 11
ZOOM
#include
#include
using namespace std;
template< typename T > class List; // forward declaration
template< typename NODETYPE >
class ListNode
{
public:
friend class List< NODETYPE >; // make List a friend
ListNode( const NODETYPE & ); // constructor
NODETYPE getData() const; // return the data in the node
// set nextPtr to nPtr
void setNextPtr( ListNode *nPtr )
{
nextPtr = nPtr;
} // end function setNextPtr
// return nextPtr
ListNode *getNextPtr() const
{
return nextPtr;
} // end function getNextPtr
private:
NODETYPE data; // data
int key; // used for key for the list
ListNode *nextPtr; // next node in the list
}; // end class ListNode
// constructor
template< typename NODETYPE >
ListNode< NODETYPE >::ListNode( const NODETYPE &info )
{
data = info;
nextPtr = 0;
} // end constructor
// return a copy of the data in the node
template< typename NODETYPE >
NODETYPE ListNode< NODETYPE >::getData() const
{
return data;
} // end function getData
template< typename NODETYPE >
class List
{
public:
List(); // default constructor
List( const List< NODETYPE > & ); // copy constructor
~List(); // destructor
void insertAtFront( const NODETYPE &, int );
void insertAtBack( const NODETYPE &, int );
bool removeFromFront( NODETYPE & );
bool removeFromBack( NODETYPE & );
bool isEmpty() const;
void print() const;
void printPtrFunc( );
NODETYPE * getInfo(int myKey);
// return nextPtr
ListNode< NODETYPE > *getFirstPtr() const
{
return firstPtr;
} // end function getNextPtr
protected:
ListNode< NODETYPE > *firstPtr; // pointer to first node
ListNode< NODETYPE > *lastPtr; // pointer to last node
// Utility function to allocate a new node
ListNode< NODETYPE > *getNewNode( const NODETYPE &, int );
}; // end class template List
// default constructor
template< typename NODETYPE >
List< NODETYPE >::List()
{
firstPtr = lastPtr = 0;
} // end constructor
// copy constructor
template< typename NODETYPE >
List< NODETYPE >::List( const List
{
firstPtr = lastPtr = 0; // initialize pointers
ListNode< NODETYPE > *currentPtr = copy.firstPtr;
// insert into the list
while ( currentPtr != 0 )
{
insertAtBack( currentPtr->data );
currentPtr = currentPtr->nextPtr;
} // end while
} // end List copy constructor
// destructor
template< typename NODETYPE >
List< NODETYPE >::~List()
{
if ( !isEmpty() ) // List is not empty
{
cout << "Destroying nodes ... ";
ListNode< NODETYPE > *currentPtr = firstPtr;
ListNode< NODETYPE > *tempPtr;
while ( currentPtr != 0 ) // delete remaining nodes
{
tempPtr = currentPtr;
// cout << tempPtr->data << ' ';
currentPtr = currentPtr->nextPtr;
delete tempPtr;
} // end while
} // end if
cout << " All nodes destroyed ";
} // end destructor
// Insert a node at the front of the list
template< typename NODETYPE >
void List< NODETYPE >::insertAtFront( const NODETYPE &value,
int key)
{
ListNode
if ( isEmpty() ) // List is empty
firstPtr = lastPtr = newPtr;
else // List is not empty
{
/*
* write code to implement insert at front
* 1. the new node needs to point to the first node
* 2. first node needs to point to the new node
* */
} // end else
} // end function insertAtFront
// Insert a node at the back of the list
template< typename NODETYPE >
void List< NODETYPE >::insertAtBack( const NODETYPE &value,
int key)
{
ListNode< NODETYPE > *newPtr = getNewNode( value, key );
if ( isEmpty() ) // List is empty
firstPtr = lastPtr = newPtr;
else // List is not empty
{
/*
* write code to implement insert at back
* 1. next pointer of the last node points to the new node
* 2. last node needs to point to the new node
* */
} // end else
} // end function insertAtBack
// Delete a node from the front of the list
template< typename NODETYPE >
bool List< NODETYPE >::removeFromFront( NODETYPE &value )
{
if ( isEmpty() ) // List is empty
return false; // delete unsuccessful
else
{
ListNode< NODETYPE > *tempPtr = firstPtr;
/*
* check to see if first pointer is the same as last point
* if it is the same, that means there is only one node, so
* set both pointer to NULL
* if it is not the same, that means there is more than one node
* in the linked list. So, make the the first node pointer points
* to the the next node of the first node
*
* Write your code to implement the remove the 1st node
*
* */
value = tempPtr->data; // data being removed
delete tempPtr;
return true; // delete successful
} // end else
} // end function removeFromFront
// delete a node from the back of the list
template< typename NODETYPE >
bool List< NODETYPE >::removeFromBack( NODETYPE &value )
{
if ( isEmpty() )
return false; // delete unsuccessful
else
{
ListNode< NODETYPE > *tempPtr = lastPtr;
if ( firstPtr == lastPtr )
firstPtr = lastPtr = 0;
else
{
ListNode< NODETYPE > *currentPtr = firstPtr;
while ( currentPtr->nextPtr != lastPtr )
currentPtr = currentPtr->nextPtr;
lastPtr = currentPtr;
currentPtr->nextPtr = 0;
} // end else
value = tempPtr->data;
delete tempPtr;
return true; // delete successful
} // end else
} // end function removeFromBack
// Is the List empty?
template< typename NODETYPE >
bool List< NODETYPE >::isEmpty() const
{
return firstPtr == 0;
} // end function isEmpty
// Return a pointer to a newly allocated node
template< typename NODETYPE >
ListNode< NODETYPE > *List< NODETYPE >::getNewNode(
const NODETYPE &value, int)
{
ListNode< NODETYPE > *ptr = new ListNode< NODETYPE >( value );
return ptr;
} // end function getNewNode
// Display the contents of the List
template< typename NODETYPE >
void List< NODETYPE >::print() const
{
if ( isEmpty() ) // empty list
{
cout << "The list is empty ";
return;
} // end if
ListNode< NODETYPE > *currentPtr = firstPtr;
// cout << "The list is: ";
while ( currentPtr != 0 ) // display elements in list
{
int i;
string s;
double d;
char c;
/*
* check to make the data type is the same
* */
if (typeid(currentPtr->data).name() == typeid(i).name() ||
typeid(currentPtr->data).name() == typeid(d).name() ||
typeid(currentPtr->data).name() == typeid(s).name() ||
typeid(currentPtr->data).name() == typeid(c).name())
{
// data value is a simple data type and can be printed
cout << currentPtr->data << ' ';
}
else {
cout <<"Can't print - Not a simple data type (int, string, char,
double) ";
}
currentPtr = currentPtr->nextPtr;
} // end while
cout << " ";
} // end function print
// Display the contents of the List
template< typename NODETYPE >
NODETYPE * List< NODETYPE >::getInfo(int myKey)
{
if ( isEmpty() ) // empty list
{
cout << "The list is empty ";
return NULL;
} // end if
ListNode< NODETYPE > *currentPtr = firstPtr;
// cout << "The list is: ";
while ( currentPtr != 0 ) // display elements in list
{
if (currentPtr->key == myKey ) // found
return (& currentPtr->data);
currentPtr = currentPtr->nextPtr;
} // end while
return NULL; // can't find
} // end function print
class Wine
{
public:
Wine();
Wine(string name, int vintage, int score, double price, string type);
void setInfo(string name, int vintage, int score,
double price, string type);
void setPrice(double price);
string getName() const;
int getPrice() const;
void printInfo();
private:
string name;
int vintage;
int score;
double price;
string type;
};
Wine::Wine()
{
price = 0;
}
Wine::Wine(string name, int vintage, int score, double price, string type)
{
this->name = name;
this->vintage = vintage;
this->score = score;
this->price = price;
this->type = type;
}
void Wine::setInfo(string name, int vintage, int score, double price,
string type)
{
this->name = name;
this->vintage = vintage;
this->score = score;
this->price = price;
this->type = type;
}
void Wine::setPrice(double price)
{
this->price = price;
}
string Wine::getName() const
{
return name;
}
int Wine::getPrice() const
{
return price;
}
void Wine::printInfo()
{
cout <<" Wine: " << name << " ; Type: " << type;
cout <<" Price: " << price << " ; Score: " << score << "Year: "
<< vintage << endl;
}
class Person
{
public:
Person();
Person(string pname, int page);
void set_name(string n) {name = n;};
void set_age(int a) {age = a;};
void set_info(string n, int a) {name = n; age=a;};
string get_name() const;
int get_age() const;
void printInfo() { cout <<"Name: "< cout << "\tAge: "< private: string name; int age; /* 0 if unknown */ }; Person::Person() { } Person::Person(string pname, int page) { name = pname; age = page; } string Person::get_name() const { return name; } int Person::get_age() const { return age; } template< typename NODETYPE > void printNoteInfo ( List< NODETYPE > & nodeList) { NODETYPE *wp; wp = (NODETYPE *) nodeList.getInfo(0); //get node based on key // wp->printInfo(); ListNode< NODETYPE > *currentPtr; currentPtr = nodeList.getFirstPtr(); // cout << "The node list is: "; //print out all the info in linked list while ( currentPtr != 0 ) // display elements in list { wp = (NODETYPE *) currentPtr; //convert to correct data type wp->printInfo(); currentPtr = currentPtr->getNextPtr(); } // end while } void printWineInfo ( List< Wine > & wineList) { Wine *wp; wp = (Wine *) wineList.getInfo(0); //get node based on key // wp->printInfo(); ListNode< Wine > *currentPtr; currentPtr = wineList.getFirstPtr(); cout << "The Wine list is: "; //print out all the info in linked list while ( currentPtr != 0 ) // display elements in list { wp = (Wine *) currentPtr; //convert to correct data type wp->printInfo(); currentPtr = currentPtr->getNextPtr(); } // end while } void printPersonInfo(List< Person > & personList) { Person *f; f = (Person *) personList.getInfo(0); //get node based on key // f->printInfo(); ListNode< Person > *currentPtr; currentPtr = personList.getFirstPtr(); cout << "The Employee list is: "; //print out all the info in linked list while ( currentPtr != 0 ) // display elements in list { f = (Person *) currentPtr; //convert to correct data type f->printInfo(); currentPtr = currentPtr->getNextPtr(); } // end while } int main() { Person p; List< Person > personList; p.set_info("Ron", 22); /* cout << p.get_name() << " is " << p.get_age() << " years old. "; */ personList.insertAtFront( p,0 ); p.set_info("Sha", 30); personList.insertAtBack( p,1 ); /* * the printPersonInfo is customized print function only for * Person data type, but it can't print any other data type * */ // cout <<" Can only print Person data type: "; printPersonInfo(personList); /* * Using template to print the linkedlist so it works will all * data type * */ cout << " Using template to print Person linked list " ; printNoteInfo (personList); Wine w; List< Wine > wineList; w.setInfo("Prisoner", 2014, 92, 44.99, "Red"); wineList.insertAtBack(w, 0); w.setInfo("Vermentino", 2014, 85, 27, "White"); wineList.insertAtFront(w, 1); w.setInfo("Stags Chardonnay Carneros", 2013, 89, 45, "White"); wineList.insertAtBack(w, 2); w.setInfo("Castello Barone Reserve Cabernet", 2011, 92, 92, "Red"); wineList.insertAtBack(w, 3); w.setInfo("Harlan Estate Bordeaux", 2011, 97, 850, "Red"); wineList.insertAtFront(w, 4); w.setInfo("Futo Bordeaux Red", 2009, 97, 324.99, "Red"); wineList.insertAtBack(w, 5); // cout <<" Can only print Wine data type: "; printWineInfo (wineList); /* * Using template to print the linkedlist so it works will all * data type * */ cout << " Using template to print Wine linked list " ; printNoteInfo (wineList); } // end main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
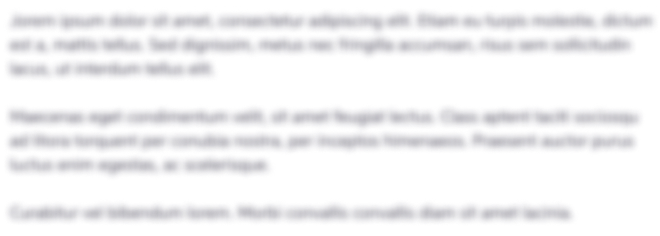
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started