Question
Module 2 Program - Deck of Cards In this project You will write two classes Deck and Card. The interface (ie, list of methods) will
Module 2 Program - Deck of Cards
In this project You will write two classes Deck and Card. The interface (ie, list of methods) will be provided for you for Deck but it will be up to you to design the interface for Card.
Deck
Deck should have three constructors. The constructor with two parameters should accept two String[] parameters and create enough cards so that every pair of strings -- one from the first array and one from the second array -- is represented exactly once. The constructor with one parameter should accept one String[] parameter and create one card for each string in the array. Finally, the no-argument constructor should call the two-argument constructor with the first array of strings being {"A","2","3","4","5","6","7","8","9","10","J","Q","K"} and the second array being either {"","","",""} or {"H","D","S","C"} (it's up to you to choose which). You can use \u2665, \u2666, \u2660, and \u2663 in your strings to represent these four unicode characters (eg, "\u2665" is a String literal with one heart character in it).
next should take no parameters and should return the next undealt Card object in the deck. If there are no cards left to deal, an IllegalStateException should be thrown. hasNext should take no parameters and return true if a call to next would succeed. remaining should take no parameters and return how many cards are left to be dealt. size should take no parameters and return how many cards are in the Deck. shuffle should take no parameters, reset the number of cards dealt to zero, and randomly reorder the cards in the deck.
Note that the Deck should be unshuffled and ready to deal when created and the initial order of the cards in the deck is specified by the constructor. A deck constructed as ({"A","B","C"}, {"1","2"}) should result in a deck order of A1, A2, B1, B2, C1, C2, ready to deal A1.
Deck should use an array to hold its cards and the array should be exactly the same size as the number of cards in the deck.
Card
You should follow the steps for designing a class seen during lecture. You are free to design the class with any methods you see fit, but that everything else in the class should be marked private. For testing purposes Card must have a toString that returns cards as "[The Chariot]" (ie, open square brace, string representing card, close square brace, with no added whitespace). Furthermore, two-valued cards should have a comma between its two values like "[10,]".
You should write Card first and test it independently of Deck to make sure it works as you'd like. Once you believe it works correctly, move on to Deck.
Example
The following code should print true when run.
String[] array1 = {"A","B","C"}; String[] array2 = {"1","2"}; Deck cards = new Deck(array1, array2); Card curCard = cards.next(); String cardsString = curCard.toString(); while (cards.hasNext()) { curCard = cards.next(); cardsString = cardsString + " " + curCard.toString(); } System.out.println(cardsString.equals("[A,1] [A,2] [B,1] [B,2] [C,1] [C,2]"));
Submission
Step by Step Solution
There are 3 Steps involved in it
Step: 1
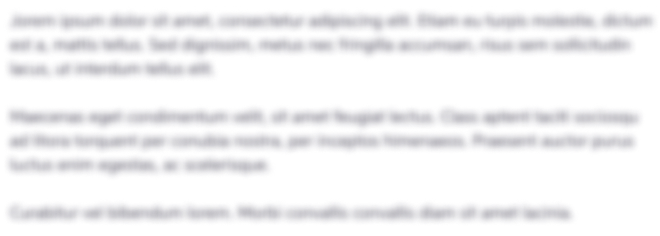
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started