Question
More on Inheritance Suppose we wish to store several different animals in an array. We do not know ahead of time what animals they will
More on Inheritance
Suppose we wish to store several different animals in an array. We do not know ahead of time what animals they will be. C++ will allow us to create a vector of pointers to Animal, and store in that vector any object of type Animal or a descendant of Animal.
Use the Animal.h and Dog.h files and get the display.h file. Then write a C++ program that reads in an integer N. This is followed by N lines, where each line contains a character, a string, and an integer, separated by spaces. The character will either have the value A or D, indicating whether the animal described on this line is a generic animal or a dog. The string and the integer describe the animal's name and age, respectively.
For each line of input, instantiate the appropriate object and push it to the vector of pointers to Animal that you have created. Once you have pushed all the animals to the vector, call the display function, found in display.h, with your vector passed as an argument.
SAMPLE OUTPUT 2 A Rex 7 Creating Generic Animal D Snoopy 4 Creating Generic Animal Creating Dog Rex Snoopy
Animal.h
#ifndef ANIMAL_H
#define ANIMAL_H
#include
#include
#include
using namespace std;
class Animal {
protected:
string name;
int age;
public:
Animal() {
cout << "Creating Generic Animal" << endl;
name = "Generic Animal";
age = 0;
}
~Animal() {
cout << "Deleting Generic Animal" << endl;
}
string getName() {
return name;
}
void setName(string name) {
this->name = name;
}
int getAge() {
return age;
}
void setAge(int age) {
this->age = age;
}
void feed() {
cout << "Some food, please!" << endl;
}
};
#endif
Dog.h
#ifndef DOG_H
#define DOG_H
#include "Animal.h"
class Dog : public Animal {
public:
Dog() {
cout << "Creating Dog" << endl;
}
Dog(string name, int age) {
cout << "Creating Dog" << endl;
this->name = name;
this->age = age;
}
~Dog() {
cout << "Deleting Dog" << endl;
}
void feed() {
cout << "Dog food, please!" << endl;
}
};
#endif
display.h
#ifndef DISPLAY_H
#define DISPLAY_H
void display( const vector
{
for( int i = 0; i < list.size(); i++ )
cout << list[i]->getName() << endl;
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
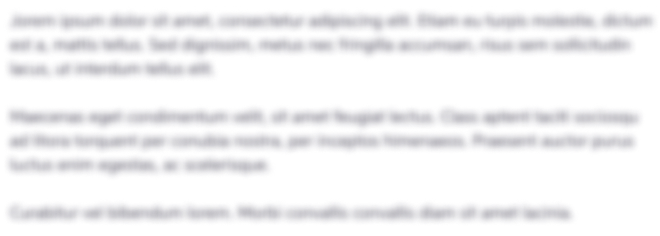
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started