Question
MUST BE IN C# Write in c# Classes you must create: Define a Vehicle class. It should have attributes for make, model, color, current mileage,
MUST BE IN C#
Write in c#
Classes you must create:
Define a Vehicle class.
It should have attributes for make, model, color, current mileage, original cost, and a boolean that keeps track of whether the vehicle is currently rented.
It should have getters/setters for each attribute.
It must have a constructor to set all attributes.
It should have an override for toString or ToString which returns a string similar to:
Available: Blue Nissan Versa with 105 miles
Rented: Blue Nissan Versa with 105 miles
Define an Economy_Car class. It should inherit from Vehicle.
It should have an attribute daily_rental_rate.
Create a constructor which takes in make, model, color, current mileage, original cost, and daily rental rate.
Create an override for toString/ToString which prints:
Economy Car: Rented: Blue Nissan Versa with 105 miles
Note most of this is just the text that your parent class prints.
Define a Midsize_Car class. It should inherit from Vehicle.
It should have an attribute daily_rental_rate.
Create a constructor which takes in make, model, color, current mileage, original cost, and daily rental rate.
Create an override for toString/ToString which prints:
Midsized Car: Available: Green Dodge Avenger with 15720 miles
Note: Most of this is just the text that your parent class prints.
Define a SUV class. It should inherit from Vehicle.
It should have an attribute daily_rental_rate.
Create a constructor which takes in make, model, color, current mileage, original cost, and daily rental rate.
Create an override for toString/ToString which prints:
SUV: Available: Silver Toyota RAV4 with 432 miles
Note: Most of this is just the text that your parent class prints. Driver Program:
DRIVER PROGRAM.
In your driver class, create an ArrayList/List of Vehicles.
Add each of the vehicles in the table above to your ArrayList/List.
Create a method show_cars which takes in the ArrayList/List and prints out all of the vehicles in a menu like this:
0. Return
1. Economy Car: Available: Blue Nissan Versa with 105 miles
2. Economy Car: Available: White Toyota Yaris with 8422 miles
3. Midsized Car: Available: Green Dodge Avenger with 15720 miles
4. Midsized Car: Available: Yellow Ford Focus with 2368 miles
5. SUV: Available: Silver Toyota RAV4 with 432 miles
Create a method rent_cars
Using a loop, prompt the user to: Choose a car to rent:. Call the show_cars() method above to produce a menu.
Read in a response, so long as its not 0, mark the chosen car as rented.
Create a method return_cars
Using a loop, prompt the user to: Choose a car to rent:. Call the show_cars() method above to produce a menu.
Read in a response, so long as its not 0, mark the chosen car as available. Produce a main menu that looks like this:
1. Rent cars
2. Return cars
3. Quit
Read in a choice and call the appropriate method above as long as the user doesnt choose 3.
SAMPLE OUTPUT:
1. Rent cars
2. Return cars
3. Quit
1 Choose a car to rent:
0. Return
1. Economy Car: Available: Blue Nissan Versa with 105 miles
2. Economy Car: Available: White Toyota Yaris with 8422 miles
3. Midsized Car: Available: Green Dodge Avenger with 15720 miles
4. Midsized Car: Available: Yellow Ford Focus with 2368 miles
5. SUV: Available: Silver Toyota RAV4 with 432 miles
1
Choose a car to rent:
0. Return
1. Economy Car: Rented: Blue Nissan Versa with 105 miles
2. Economy Car: Available: White Toyota Yaris with 8422 miles
3. Midsized Car: Available: Green Dodge Avenger with 15720 miles
4. Midsized Car: Available: Yellow Ford Focus with 2368 miles
5. SUV: Available: Silver Toyota RAV4 with 432 miles
3
Choose a car to rent:
0. Return
1. Economy Car: Rented: Blue Nissan Versa with 105 miles
2. Economy Car: Available: White Toyota Yaris with 8422 miles
3. Midsized Car: Rented: Green Dodge Avenger with 15720 miles
4. Midsized Car: Available: Yellow Ford Focus with 2368 miles
5. SUV: Available: Silver Toyota RAV4 with 432 miles
0
1. Rent cars
2. Return cars
3. Quit
2
Choose a car to return:
0. Return
1. Economy Car: Rented: Blue Nissan Versa with 105 miles
2. Economy Car: Available: White Toyota Yaris with 8422 miles
3. Midsized Car: Rented: Green Dodge Avenger with 15720 miles
4. Midsized Car: Available: Yellow Ford Focus with 2368 miles
5. SUV: Available: Silver Toyota RAV4 with 432 miles
1
Choose a car to return:
0. Return
1. Economy Car: Available: Blue Nissan Versa with 105 miles
2. Economy Car: Available: White Toyota Yaris with 8422 miles
3. Midsized Car: Rented: Green Dodge Avenger with 15720 miles
4. Midsized Car: Available: Yellow Ford Focus with 2368 miles
5. SUV: Available: Silver Toyota RAV4 with 432 miles
3 Choose a car to return:
0. Return
1. Economy Car: Available: Blue Nissan Versa with 105 miles
2. Economy Car: Available: White Toyota Yaris with 8422 miles
3. Midsized Car: Available: Green Dodge Avenger with 15720 miles
4. Midsized Car: Available: Yellow Ford Focus with 2368 miles
5. SUV: Available: Silver Toyota RAV4 with 432 miles
0
1. Rent cars
2. Return cars
3. Quit 3
Background: A rental car company has hired you to build an inventory system to keep track of their vehicles. The company, Acme Car Rentals, rents 3 classes of cars: Economy, Midsized and SUVs. Each rents for a different price. Their initial inventory is as follows: Type Make Model Color Current Mileage Original Cost Rental Rate Nissan Versa Blue 105 $14,500 $25/day Economy Economy Midsized Toyota Yaris White 8422 $17,500 $25/day Dodge Avenger Green 15720 $15,000 $45/day Midsized Ford Focus Yellow 2368 $14,500 $45/day SUV Toyota Rav4 Silver 432 $26,150 $80/dayStep by Step Solution
There are 3 Steps involved in it
Step: 1
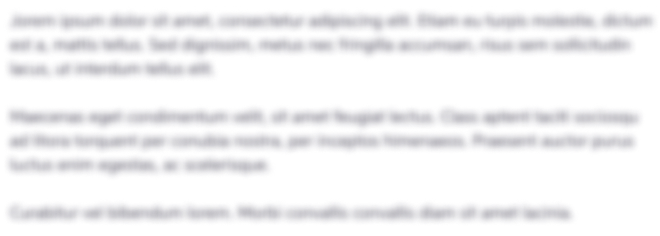
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started