Question
____________________________ My purchase class is: import java.util.Scanner; Public class Purchase { private String name; private int groupCount; //Part of price, like the 2 in 2
____________________________
My purchase class is:
import java.util.Scanner;
Public class Purchase
{
private String name;
private int groupCount; //Part of price, like the 2 in 2 for $1.99.
private double groupPrice;//Part of price, like the $1.99
// in 2 for $1.99.
private int numberBought; //Number of items bought.
public void setName(String newName)
{
name = newName;
}
/**
Sets price to count pieces for $costForCount.
For example, 2 for $1.99.
*/
public void setPrice(int count, double costForCount)
{
if ((count <= 0) || (costForCount <= 0))
{
System.out.println("Error: Bad parameter in setPrice.");
System.exit(0);
}
else
{
groupCount = count;
groupPrice = costForCount;
}
}
public void setNumberBought(int number)
{
if (number <= 0)
{
System.out.println("Error: Bad parameter in setNumberBought.");
System.exit(0);
}
else
numberBought = number;
}
/**
Reads from keyboard the price and number of a purchase.
*/
public void readInput( )
{
Scanner keyboard = new Scanner(System.in);
System.out.println("Enter name of item you are purchasing:");
name = keyboard.nextLine( );
System.out.println("Enter price of item as two numbers.");
System.out.println("For example, 3 for $2.99 is entered as");
System.out.println("3 2.99");
System.out.println("Enter price of item as two numbers, now:");
groupCount = keyboard.nextInt( );
groupPrice = keyboard.nextDouble( );
while ((groupCount <= 0) || (groupPrice <= 0))
{ //Try again:
System.out.println("Both numbers must be positive. Try again.");
System.out.println("Enter price of item as two numbers.");
System.out.println("For example, 3 for $2.99 is entered as");
System.out.println("3 2.99");
System.out.println("Enter price of item as two numbers, now:");
groupCount = keyboard.nextInt( );
groupPrice = keyboard.nextDouble( );
}
System.out.println("Enter number of items purchased:");
numberBought = keyboard.nextInt( );
while (numberBought <= 0)
{ //Try again:
System.out.println("Number must be positive. Try again.");
System.out.println("Enter number of items purchased:");
numberBought = keyboard.nextInt( );
}
}
/**
Displays price and number being purchased.
*/
public void writeOutput( )
{
System.out.println(numberBought + " " + name);
System.out.println("at " + groupCount +
" for $" + groupPrice);
}
public String getName( )
{
return name;
}
public double getTotalCost( )
{
return (groupPrice / groupCount) * numberBought;
}
public double getUnitCost( )
{
return groupPrice / groupCount;
}
public int getNumberBought( )
{
return numberBought;
}
}
_____________________________
Assume the following method is added to the class Purchase. Assume the Purchase class has an explicate constructor that has parameters for all the instance variables.
public Purchase testQuestion ( Purchase p1, int newX)
{
newX = 20;
this.setName(pears");
p1.setName(oranges);
p1 = new Purchase (kiwi, 6, 3.00, 3);
Purchase p2 = new Purchase (banana, 5, 4.50, 10);
System.out.println(this.getName());
System.out.println(p1.getName());
System.out.println(p2.getName());
return p2;
}
The Question is:
Referring to the above method, list in the margin to the right the values that would be printed by System.out.println() when the following PurchaseDemo is executed.
public class PurchaseDemo
{
public static void main(String[] args)
{
Purchase oneSale = new Purchase(apples, 4, 3.50, 6);
Purchase twoSale = new Purchase(grapes, 8, 1.99, 4 );
Purchase threeSale;
int x = 10;
System.out.println(oneSale.getName());
System.out.println(twoSale.getName());
threeSale = oneSale.testQuestion(twoSale, x);
System.out.println(oneSale.getName());
System.out.println(twoSale.getName());
System.out.println(threeSale.getName());
System.out.println(x);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
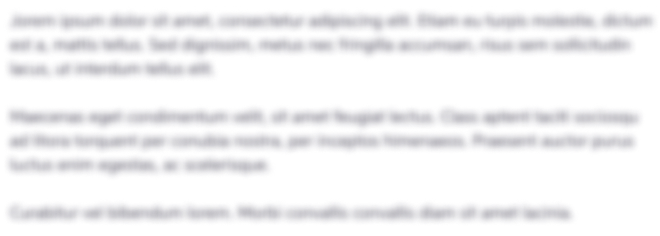
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started