Question
name_list.c: #include #include #include #include #include rm_library.h #include name_list.h void initialize_name_list(sNAME_LIST *name_list) //============================================== // The empty name_list has both, front and rear pointers set to
name_list.c:
#include
#include
#include
#include
#include "rm_library.h"
#include "name_list.h"
void initialize_name_list(sNAME_LIST *name_list)
//==============================================
// The empty name_list has both, front and rear pointers set to NULL because the list does NOT contain any nodes, i.e. names.
// Of course, the count field is set to ZERO for the same reason.
{
static char pname[] = "initialize_name_list()";
if ( name_list != NULL ) { // Check for undefinedon-existent "name_list".
name_list->front = NULL; // set front of list pointer to NULL pointer.
name_list->rear = NULL; // set rear of list pointer to NULL pointer.
name_list->count = 0.0; // list has ZERO names initially.
}else {
sprintf(msg[0], "%s%s", "In Function: ", pname);
sprintf(msg[1], "%s", "Internal Error: List not Initialized because it does not exist!");
report_message(msg, MSG_ERROR);
}
return;
}
int free_name_list(sNAME_LIST *name_list )
//=========================================
// Given the Single-Linked list "name_list", this subprogram CLEANS the list. That is, it frees all of the nodes in the list,
// including the header node. It returns a count of the number of nodes freed, including the list header node.
{
static char pname[] = "free_name_list()";
int n_freed; // Count of number of nodes "freed", including header node.
sNODE *p_ntf; // Pointer to next "Node-To-Free", or node to "clean".
n_freed = 0;
if ( name_list != NULL ) { // Check for undefinedon-existent "name_list".
if ( (name_list->front != NULL) &&
(name_list->rear != NULL) &&
(name_list->count > 0 ) ) { // Check for empty list
// ==> List is NOT empty!
while ( name_list->front != name_list->rear ) {
// Traverse the name_list from front to rear, and free every node encountered.
p_ntf = name_list->front; // p_ntf points to the next node to free. This is always the first node in the list.
name_list->front = p_ntf->next; // "de-link" (i.e. drop/remove) the p_ntf node from the list.
// The node following the p_ntf node is now at the front of the list.
free(p_ntf); // free the de-linked p_ntf node.
n_freed++; // update freed counter.
}
free( name_list->front ); // Free the last remaining node in the list.
n_freed++;
}else {
sprintf(msg[0], "%s%s", "In Function: ", pname);
sprintf(msg[1], "%s", "List is EMPTY! Only header node freed.");
report_message(msg, MSG_INFO);
}
free(name_list); // Free header node.
name_list = NULL;
n_freed++;
}else {
sprintf(msg[0], "%s%s", "In Function: ", pname);
sprintf(msg[1], "%s", "Internal Error: List not freed because it does not exist!");
report_message(msg, MSG_ERROR);
}
return (n_freed);
}
int output_name_list(sNAME_LIST *name_list) // Linked list of names.
//==========================================
{
static char pname[] = "output_name_list()";
int n_printed; // Number of names output/printed.
sNODE *p_scan; // Scan pointer used to traverse the "name_list".
if ( name_list != NULL ) { // Check for undefinedon-existent "name_list".
if ( (name_list->front != NULL) &&
(name_list->rear != NULL) &&
(name_list->count > 0 ) ) { // Check for empty list.
// ===>List is NOT EMPTY, so let's print it's contents.
printf(NL);
printf("List of Names with duplicates REMOVED: "); // Always nice to label the output with a descriptive heading.
printf("====================================== ");
n_printed = 0;
p_scan = name_list->front; // Set p_scan to point to the first node in the last.
while ( n_printed count ) { // Traverse the name_list from beginning to end and output
// the data content of each node.
printf("%s %s ", p_scan->name.first_name,
p_scan->name.last_name);
n_printed++; // Update the counter to keep track of the no. of names printed.
p_scan = p_scan->next; // Update the scan pointer to point to the next node.
}
}else {
sprintf(msg[0], "%s%s", "In Function: ", pname);
sprintf(msg[1], "%s", "List is EMPTY! Nothing to output/print.");
report_message(msg, MSG_INFO);
}
}else {
sprintf(msg[0], "%s%s", "In Function: ", pname);
sprintf(msg[1], "%s", "Internal Error: List not printed because it does not exist!");
report_message(msg, MSG_ERROR);
}
return (n_printed);
}
name_list.h:
#ifndef NAME_LIST_H_
#define NAME_LIST_H_
#define MAX_FIRST_NAME_LNG 41 // Max. size of First Name field +end byte.
#define MAX_LAST_NAME_LNG MAX_FIRST_NAME_LNG // Max. size of Last Name field +end byte.
#define MAX_MIDDLE_NAME_LNG MAX_FIRST_NAME_LNG // Max. size of Middle Name field +end byte.
typedef struct { // Name Structure storing the First, Last, and Middle name of a person's name.
char first_name[MAX_FIRST_NAME_LNG]; // Stores the person's first name.
char last_name [MAX_LAST_NAME_LNG ]; // Stores the person's last name.
} sNAME; // sNAME is the name given to the person's name structure
typedef struct node{ // List node structure.
sNAME name; // Compound field storing the person's name.
struct node *next; // Pointer to an sNODE node.
} sNODE; // sNODE is the name given to the node structure.
typedef struct {
sNODE *front; // Pointer to the FIRST nodeame of the sNAME_LIST.
sNODE *rear; // Pointer to the LAST nodeame of the sNAME_LIST.
int count; // Count of number of nodesames are present in the sNAME_LIST.
} sNAME_LIST;
//===== SUBPROGRAM PROTOTYPES ===============================================================================================
// Subprogram to initialize the "name_list".
void initialize_name_list(sNAME_LIST *name_list);
// Subprogram to free (i.e. Clean) all the nodes in the "name_list".
int free_name_list(sNAME_LIST *name_list);
// Subprogram to print the Data content of the "name_list".
int output_name_list(sNAME_LIST *name_list);
// Subprogram to insert a name in the list, but only if the name is NOT
// already in the list.
void insert_name_list(sNAME_LIST *name_list,
sNODE *node );
#endif
main.c:
Correct Output:
names.txt:
Heider Ali Rick Magnotta Mitchel Brown Tyler Buttons David Brown Roy Shannon Shawn Davis Tom Elliot Adamo Iannucci Paige Kerrigan Shawn Davis Lucy Lee David Brown Roxana Paslawski Tom Elliot Rick Magnotta Morgan Pekova Morgan Pekova
Your assigned task is to write the code for the "insert_name_list(...)" subprogram in the name_list.c file, whose prototype is shown below and is defined in the name_list.h header file. void insert_name_list(sNAME_LIST *name_list, // The linked list of names. sNODE *node ); // The node containing the name. The purpose of the "insert_name_list(...)" subprogrm is to build a single-linked list of names, with the following provisions: - A given name is inserted in the "name_list" ONLY if the name is not already present in the list. - If a name is already present in the list, then the "insert_name_list(...)" routine simply discards the given node by freeing it's storage. - A new node is always inserted at the end of the list, that is, it is appended to the list. - Stop searching the list of names as soon as the target name is found to be present in the list. - An appropriate error message is printed if the "name_list" does not exist, or is undefined. Compile and execute the project to test your code. You MUST NOT alter any of the given code. Your list must be constructed so that it will work with the given source code. When you complete your tasks, submit the name_list.c file to the dropbox. Correct Output: List of Names with duplicates REMOVED: Heider Ali Rick Magnotta Mitchel Brown Tyler Buttons David Brown Roy Shannon Shawn Davis Tom Elliot Adamo Iannucci Paige Kerrigan Lucy Lee Roxana Paslawski Morgan Pekova ::> MSG: The list contains 13 unique names. |||| : Number of names printed = 13 END: Program Terminated. || : Have a great dayStep by Step Solution
There are 3 Steps involved in it
Step: 1
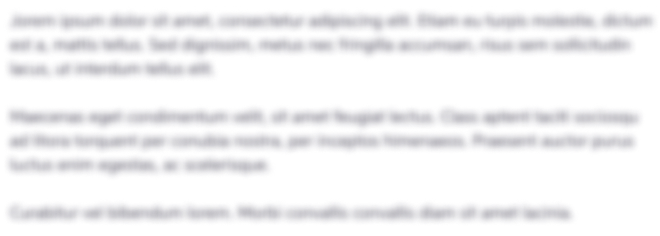
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started