Question
Need assistance with a Python program using classes. Several type of shapes will need to be defined: circle, rectangle, and right triangle. These shapes translate
Need assistance with a Python program using classes. Several type of shapes will need to be defined: circle, rectangle, and right triangle. These shapes translate directly to classes. Each shape has its own data, a draw method and a get area method. Once the classes have been written, a program will need to be written that will randomly create 15 shapes and have the shapes draw themselves. Assignment instructions attached with a sample run. Please also include comments explaining what everything does.
This assignment will give you practice on writing classes and understanding inheritance. You will define several type of shapes: circle, rectangle, and right triangle. These shapes translates directly to classes. Each shape has its own data, a draw method and a get area method. Once you have written the classes, you will write a program that will randomly create 15 shapes and have the shapes draw themselves. The screen shown below is the result of a sample run.
Shape
has a class variable turtle, initialized to a Turtle object.
Circle
inherits Shape
has a center point, a tuple of x and y
has a radius
has a draw method
has a get_area method
Rectangle
inherits Shape
has an upper_left point
has a lower_right point
has draw method
has get_area method
RightTriangle
inherits Shape
has two points A and B.
has draw method
has get_area method
main function
Generates 15 random shapes.
Draw them
Print their total area
If you are not sure how to start, theres a partial solution on the back you can study. If possible, start from scratch on your own. Heres the link to different operations you can perform with turtle graphics: https://docs.python.org/3.3/library/turtle.html?highlight=turtle
from turtle import Turtle
class Shape():
turtle = Turtle()
class Circle(Shape):
def __init__(self, center, radius): self.center = center self.radius = radius
def get_area(self): return 3.14* self.radius*self.radius
def draw(self): Shape.turtle.penup() Shape.turtle.setposition(self.center[0], self.center[1] - self.radius) Shape.turtle.pendown() Shape.turtle.circle(self.radius) Shape.turtle.penup()
def random_shapes(count):
def random_point(): return (random.randint(-200,200), random.randint(-200,200))
shapes = []
for i in range(1, count+1): shape_type = random.randint(1, 3) if shape_type == 1: shapes+=[Circle(random_point(), random.randint(1,200))] elif shape_type == 2: shapes+=[Rectangle(random_point(), random_point())] else: shapes+=[RightTriangle(random_point(), random_point())]
return shapes
def main():
shapes = random_shapes(15) total_area = 0 for s in shapes: s.draw() total_area += s.get_area()
input("Hit
main()
Sample Output
Step by Step Solution
There are 3 Steps involved in it
Step: 1
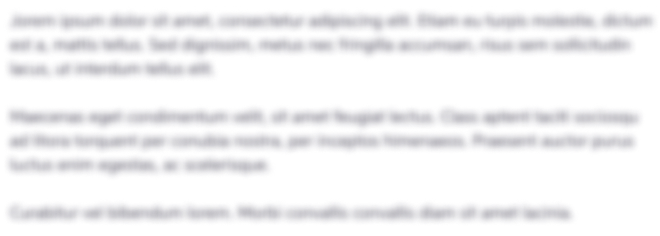
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started