NEED HELP ASAP WITH JAVA ASSIGNMENT! This is a java assignment, I am nearly complete but I am struggling in particular with the harbor class, and I would like to see you complete it so I can compare it to my own work and see where I need to make changes. Attached are screenshots of the assignment, which have directions. Please make sure you implement all classes, including an "OuterMain" class. I would appreciate many comments on your code so I can understand better what I need to do. Please also make sure to implement the two interfaces that were provided in the instructions. Here are the images:


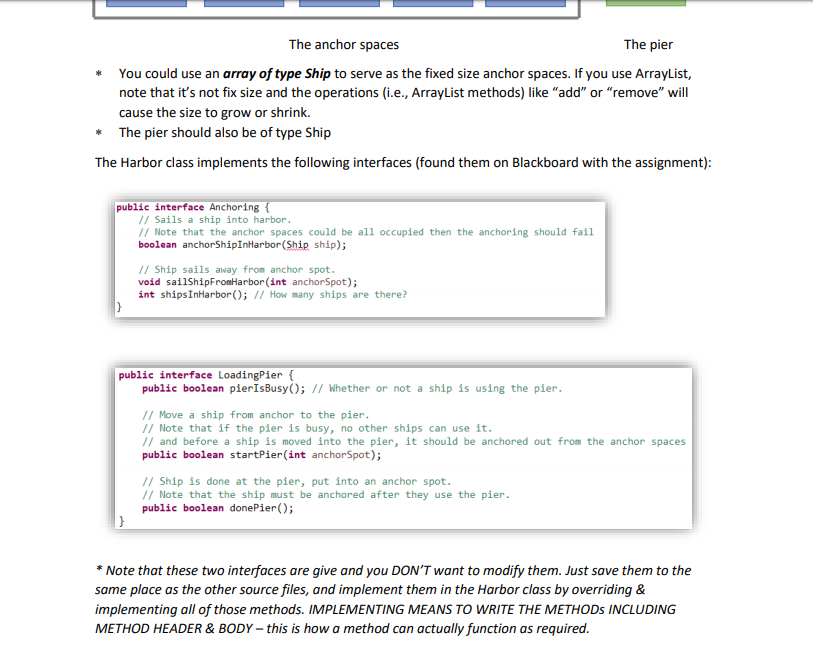

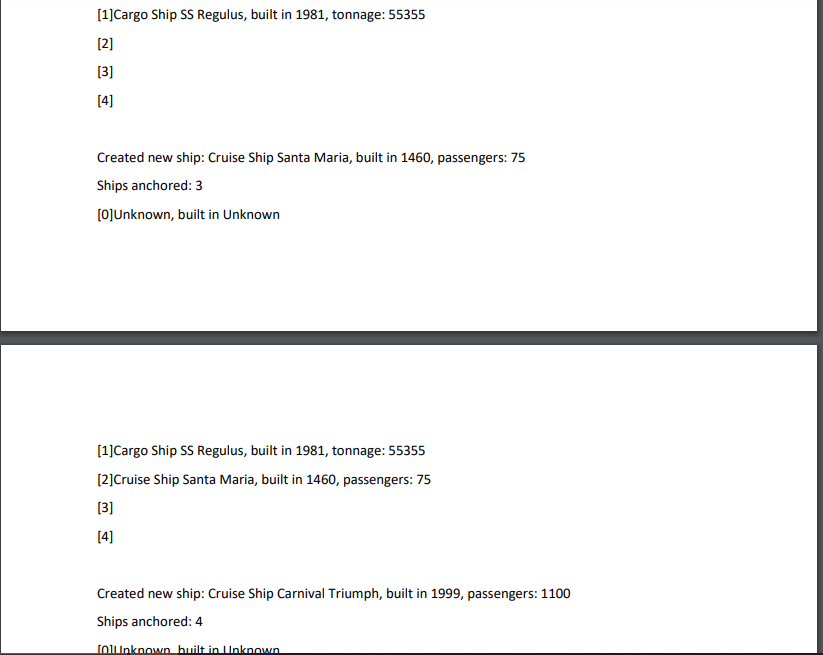




Due Friday, Feb 21h on Blackboard Submission: Zip the whole project folder and submit to BB A Harbor has enough anchor space for 5 ships and one pier for loading/unloading. A Ship comes as either a CruiseShip or a CargoShip. We will need to create classes for Ship, Cruise Ship, CargoShip, Harbor, and an OuterMain to simulate scenarios in a busy harbor. 1. Class Description 1.1. Ship Design a Ship class that has the following members: A field for the name of the ship (a string) A field for the year that the ship was built (an int) Constructors o for all fields provided o for no built year provided (set the built year to -1) o for no name provided (set name to "") o for neither provided Appropriate getters and setters A toString method that displays the ship's name and the year it was built. If the ship has no name or a negative year of build, "unknown" should be used instead. 1.2. Cruise Ship Design a Cruise Ship class that is derived from the Ship class. The Cruise Ship class should have the following members: A field for the maximum number of passengers (an int) Constructors o for all data provided o for only passengers provided o any other as needed Appropriate setters and getters A toString method that overrides the according method in the superclass. It should additionally display the maximum number of passengers. 1.3. CargoShip Design a CargoShip class that is derived from the Ship class. The CargoShip class should have the following members: A field for the cargo capacity in tonnage (an int) Constructors o for all data provided o for only tonnage provided o any other as needed Appropriate setters and getters A toString method that overrides the according method in the superclass. It should additionally display the ship's cargo capacity. 1.4. Harbor The Harbor class is holding five anchor spaces for ships and one pier for loading & unloading. The anchor spaces The pier The anchor spaces The pier * You could use an array of type Ship to serve as the fixed size anchor spaces. If you use ArrayList, note that it's not fix size and the operations (i.e., ArrayList methods) like "add" or "remove" will cause the size to grow or shrink. The pier should also be of type Ship The Harbor class implements the following interfaces (found them on Blackboard with the assignment): public interface Anchoring { // Sails a ship into harbor. // Note that the anchor spaces could be all occupied then the anchoring should fail boolean anchorShipInHarbor (Ship ship); // Ship sails away from anchor spot. void sailShipFromHarbor(int anchorSpot); int ships InHarbor(); // How many ships are there? public interface LoadingPier { public boolean pierIsBusy(); // Whether or not a ship is using the pier. // Move a ship from anchor to the pier. // Note that if the pier is busy, no other ships can use it. // and before a ship is moved into the pier, it should be anchored out from the anchor spaces public boolean startPier(int anchorSpot); // Ship is done at the pier, put into an anchor spot. // Note that the ship must be anchored after they use the pier. public boolean donePier(); * Note that these two interfaces are give and you DON'T want to modify them. Just save them to the same place as the other source files, and implement them in the Harbor class by overriding & implementing all of those methods. IMPLEMENTING MEANS TO WRITE THE METHODS INCLUDING METHOD HEADER & BODY - this is how a method can actually function as required. The Harbor will also require a toString method that calls each ship's respective toString method (i.e. the Harbor's toString method lists all the ships currently anchored and the one that might be at the pier). 2. Scenario Simulation Implement scenario in Outer Main class and display corresponding output. Your scenario should involve the following jobs at possible situation: creating new ship(s) anchoring ships into harbor (anchor spots may be full) adding ships into pier (pier may be occupied) removing ship from pier (the ship may return to anchor spot or ...) sailing ship (move it out from the harbor / pier) etc. 3. Example Outputs 3.1. Scenario #1: Created new ship: Unknown, built in Unknown Ships anchored: 1 [O]Unknown, built in Unknown Created new ship: Cargo Ship SS Regulus, built in 1981, tonnage: 55355 Ships anchored: 2 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2] Created new ship: Cruise Ship Santa Maria, built in 1460, passengers: 75 Ships anchored: 3 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3] [4] Created new ship: Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 Ships anchored: 4 rolunknown built in Unknown Created new ship: Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 Ships anchored: 4 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4] Created new ship: U-boat, built in Unknown Ships anchored: 5 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Created new ship: Lusitania, built in 1915 Ships anchored: 5 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Adding Oto pier: true Ships anchored: 4 [O] [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Ship at pier: Unknown, built in Unknown Adding 1 to pier: false Ships anchored: 4 [0] Adding 1 to pier: false Ships anchored: 4 [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Ship at pier: Unknown, built in Unknown Sailing 1 from harbor. Ships anchored: 3 [0] [1] [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Ship at pier: Unknown, built in Unknown Sailing 2 from harbor. Ships anchored: 2 [0] [2] [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Ship at pier: Unknown, built in Unknown Ship is done at pier. Returned to a harbor anchor spot Ships anchored: 3 [OJUnknown, built in Unknown [2] [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Adding 3 to pier: true Ships anchored: 2 [OJUnknown, built in Unknown [2] [3] [4]U-boat, built in Unknown Ship at pier: Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 2.2 Scenario #2 Due Friday, Feb 21h on Blackboard Submission: Zip the whole project folder and submit to BB A Harbor has enough anchor space for 5 ships and one pier for loading/unloading. A Ship comes as either a CruiseShip or a CargoShip. We will need to create classes for Ship, Cruise Ship, CargoShip, Harbor, and an OuterMain to simulate scenarios in a busy harbor. 1. Class Description 1.1. Ship Design a Ship class that has the following members: A field for the name of the ship (a string) A field for the year that the ship was built (an int) Constructors o for all fields provided o for no built year provided (set the built year to -1) o for no name provided (set name to "") o for neither provided Appropriate getters and setters A toString method that displays the ship's name and the year it was built. If the ship has no name or a negative year of build, "unknown" should be used instead. 1.2. Cruise Ship Design a Cruise Ship class that is derived from the Ship class. The Cruise Ship class should have the following members: A field for the maximum number of passengers (an int) Constructors o for all data provided o for only passengers provided o any other as needed Appropriate setters and getters A toString method that overrides the according method in the superclass. It should additionally display the maximum number of passengers. 1.3. CargoShip Design a CargoShip class that is derived from the Ship class. The CargoShip class should have the following members: A field for the cargo capacity in tonnage (an int) Constructors o for all data provided o for only tonnage provided o any other as needed Appropriate setters and getters A toString method that overrides the according method in the superclass. It should additionally display the ship's cargo capacity. 1.4. Harbor The Harbor class is holding five anchor spaces for ships and one pier for loading & unloading. The anchor spaces The pier The anchor spaces The pier * You could use an array of type Ship to serve as the fixed size anchor spaces. If you use ArrayList, note that it's not fix size and the operations (i.e., ArrayList methods) like "add" or "remove" will cause the size to grow or shrink. The pier should also be of type Ship The Harbor class implements the following interfaces (found them on Blackboard with the assignment): public interface Anchoring { // Sails a ship into harbor. // Note that the anchor spaces could be all occupied then the anchoring should fail boolean anchorShipInHarbor (Ship ship); // Ship sails away from anchor spot. void sailShipFromHarbor(int anchorSpot); int ships InHarbor(); // How many ships are there? public interface LoadingPier { public boolean pierIsBusy(); // Whether or not a ship is using the pier. // Move a ship from anchor to the pier. // Note that if the pier is busy, no other ships can use it. // and before a ship is moved into the pier, it should be anchored out from the anchor spaces public boolean startPier(int anchorSpot); // Ship is done at the pier, put into an anchor spot. // Note that the ship must be anchored after they use the pier. public boolean donePier(); * Note that these two interfaces are give and you DON'T want to modify them. Just save them to the same place as the other source files, and implement them in the Harbor class by overriding & implementing all of those methods. IMPLEMENTING MEANS TO WRITE THE METHODS INCLUDING METHOD HEADER & BODY - this is how a method can actually function as required. The Harbor will also require a toString method that calls each ship's respective toString method (i.e. the Harbor's toString method lists all the ships currently anchored and the one that might be at the pier). 2. Scenario Simulation Implement scenario in Outer Main class and display corresponding output. Your scenario should involve the following jobs at possible situation: creating new ship(s) anchoring ships into harbor (anchor spots may be full) adding ships into pier (pier may be occupied) removing ship from pier (the ship may return to anchor spot or ...) sailing ship (move it out from the harbor / pier) etc. 3. Example Outputs 3.1. Scenario #1: Created new ship: Unknown, built in Unknown Ships anchored: 1 [O]Unknown, built in Unknown Created new ship: Cargo Ship SS Regulus, built in 1981, tonnage: 55355 Ships anchored: 2 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2] Created new ship: Cruise Ship Santa Maria, built in 1460, passengers: 75 Ships anchored: 3 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3] [4] Created new ship: Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 Ships anchored: 4 rolunknown built in Unknown Created new ship: Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 Ships anchored: 4 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4] Created new ship: U-boat, built in Unknown Ships anchored: 5 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Created new ship: Lusitania, built in 1915 Ships anchored: 5 [O]Unknown, built in Unknown [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Adding Oto pier: true Ships anchored: 4 [O] [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Ship at pier: Unknown, built in Unknown Adding 1 to pier: false Ships anchored: 4 [0] Adding 1 to pier: false Ships anchored: 4 [1]Cargo Ship SS Regulus, built in 1981, tonnage: 55355 [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Ship at pier: Unknown, built in Unknown Sailing 1 from harbor. Ships anchored: 3 [0] [1] [2]Cruise Ship Santa Maria, built in 1460, passengers: 75 [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Ship at pier: Unknown, built in Unknown Sailing 2 from harbor. Ships anchored: 2 [0] [2] [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Ship at pier: Unknown, built in Unknown Ship is done at pier. Returned to a harbor anchor spot Ships anchored: 3 [OJUnknown, built in Unknown [2] [3]Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 [4]U-boat, built in Unknown Adding 3 to pier: true Ships anchored: 2 [OJUnknown, built in Unknown [2] [3] [4]U-boat, built in Unknown Ship at pier: Cruise Ship Carnival Triumph, built in 1999, passengers: 1100 2.2 Scenario #2