Question
Need help creating and implementing a linked list to a program. C++ Linked List Implementation Our last program was to maintain a list of books
Need help creating and implementing a linked list to a program.
C++ Linked List Implementation
Our last program was to maintain a list of books using a vector class created by you. In this assignment, you will be writing the linked list. Implement class LinkedList consisting of Nodes of a generic type.
Nodes
The Node class must be represented as a template.
The Node class will require these private fields.
- T data: The data to hold in the Node
- Node* next: A pointer to the next Node (if any) in the chain of Nodes.
- Node* prev: A pointer to the previous Node (if any) in the chain of Nodes.
Linked List
This class will require the following fields:
- Node* mHead: Points the first Node in the chain
- Node* mTail: Points the last Node in the chain
- int mSize: Keeps a count of the number of Nodes in the chain
Your LinkedList class must also support the following public member functions.
- LinkedList(): A default constructor sets both pointers to null and sets the size to 0.
- LinkedList(const LinkedList&): A copy constructor that takes a LinkedList object and performs a deep copy of the passed list into this list.
- ~LinkedList(): A destructor that performs pop_front on the list while the list is not empty.
- operator=(const LinkedList&): LinkedList& An overloaded assignment operator that deletes the current array and replaces it with deep copy of the passed list.
- size() const: int: Returns the size of the LinkedList.
- push_back(const T): void: Creates a new Node and assigns it to the end of the list of Nodes while updating the size by 1.
- push_front(const T): void: Creates a new Node and assigns it to the front of the list of Nodes while updating the size by 1.
- pop_back(): T: Deletes the Node at the end of the list (or throws an error if this is not possible). Decreases size by 1. Returns the deleted element.
- pop_front(): T: Deletes the Node at the front of the list (or throws an error if this is not possible). Decreases size by 1. Returns the deleted element.
- at(int) const: T: Returns the element stored at the specified index. If the index is out-of-bounds (either for being negative or greater than/equal the size of the list), throw invalid\_argument("Index out of bounds.");. This will require you to include the stdexcept library. This method should operate at O(1) for accessing the first or last element in a list and at O(n) for any element other than the first or the last.
- front() const: T: Returns the first element.
- back() const: T: Returns the last element.
Return to the Books object.
Return to your Books code. Find Books.h and remove the import statements to vector.hpp and replace those with the local version of LinkedList.hpp that you made.
- Add a menu option to add to the beginning of the list.
- Add a menu option to remove the first Book in the list.
- Add a menu option to remove the last Book in the list.
- Add a menu option to remove every Book in the list.
Provided Node Header File:
#ifndef Node_HPP #define Node_HPP #include
template
Provided LinkedList Header File:
#ifndef LinkedList_HPP #define LinkedList_HPP #include
PREVIOUS FILES:
vector.hpp
#pragma once #ifndef VECTOR_H #define VECTOR_H
#include
template
template
template
template
template
template
mData[mSize] = val; mSize++; }
template
template
#endif VECTOR_H
Book.hpp
#pragma once #ifndef BOOK_HPP #define BOOK_HPP
#include
class Book { string name; string author; int publishYear; int pages;
public: Book(); Book(string, string, int, int); string getName(); string getAuthor(); int getPublishYear(); int getPages(); };
#endif
Book.cpp
#include
Book::Book() {} Book::Book(string n, string a, int y, int p) { if (n == "") { throw "Name of Book Cannot Be Empty"; } if (a == "") { throw "Name of Author Cannot Be Empty"; } if (y <= 1990) { throw "The Publish Year Cannot Be Empty"; } if (p <= 0) { throw "The Pages Cannot Be Less Than Zero"; }
name = n; author = a; publishYear = y; pages = p; }
string Book::getName() { return name; }
string Book::getAuthor() { return author; }
int Book::getPublishYear() { return publishYear; }
int Book::getPages() { return pages; }
Books.hpp
#pragma once #ifndef BOOKS_HPP #define BOOKS_HPP
#include "vector.hpp" #include "Book.hpp" using namespace std;
class Books { private: vector
#endif
Books.cpp
#include "vector.hpp" #include "Books.hpp" using namespace std;
void Books::addBook(Book b) { books.push_back(b); }
int Books::getCount() { return books.size(); }
Book Books::getBookWithHighestPages() { int mostPages = INT_MIN; int mostPagesBook = -1; for (int i = 0; i < books.size(); i++) { if (books[i].getPages() > mostPages) { mostPages = books[i].getPages(); mostPagesBook = i; } } return books[mostPagesBook]; }
Book Books::get(int i) { return books[i]; }
int Books::getNumberOfBooksHavePagesMoreThan(int i) { int numOfBooks = 0; for (int j = 0; j < books.size(); j++) { if (books[j].getPages() > i) { numOfBooks++; } } return numOfBooks; }
main.cpp
#include
using namespace std;
Book newBook() {
string name; string author; int publishYear; int pages;
cout << "Enter the Name of the Book: "; cin >> name; cout << "Enter the Author of the Book: "; cin >> author; cout << "Enter the Year the Book was Published: "; cin >> publishYear; cout << "Enter the Number of Pages: "; cin >> pages; cout << endl;
Book b(name, author, publishYear, pages); return b; }
int main() { int choice; bool runProgram = true; Book temp; Books b;
while (runProgram) { cout << "================ Books Menu ================" << endl; cout << "1. Add Book" << endl; cout << "2. Get Book Count" << endl; cout << "3. Get Book With Most Pages" << endl; cout << "4. Get Number of Books With More Pages Than:" << endl; cout << "5. Exit" << endl; cout << "Enter Your Choice: "; cin >> choice; cout << endl;
switch (choice) { case 1: b.addBook(newBook()); break; case 2: cout << "Number of Books: " << b.getCount() << endl << endl; break; case 3: temp = b.getBookWithHighestPages(); cout << "The Book With The Most Pages Is: " << temp.getName() << ", with a total of " << temp.getPages() << " pages. It was written by " << temp.getAuthor() << " and was published in " << temp.getPublishYear() << "." << endl << endl; break; case 4: int numOfPages; cout << "Enter The Number of Pages: "; cin >> numOfPages; cout << endl << "Number of Books With More Pages Than " << numOfPages << ": " << b.getNumberOfBooksHavePagesMoreThan(numOfPages) << endl << endl; break; case 5: runProgram = false; break; default: cout << "That is not one of the options, please enter a number 1-5." << endl << endl; break; } } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
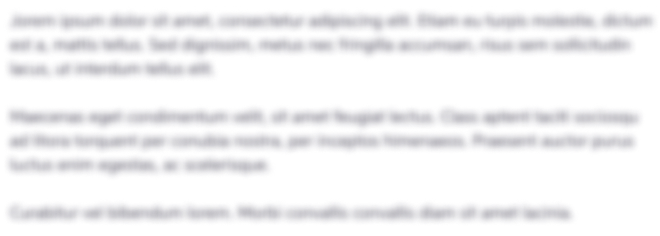
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started