Question
Need help creating Java JUnit test for the following code... package medical.com.medicalApplication.prompts; import java.util.Arrays; import java.util.List; import java.util.Scanner; import medical.com.medicalApplication.model.Allergey; import medical.com.medicalApplication.model.MedicalRecord; import medical.com.medicalApplication.model.Medication; import
Need help creating Java JUnit test for the following code...
package medical.com.medicalApplication.prompts;
import java.util.Arrays; import java.util.List; import java.util.Scanner;
import medical.com.medicalApplication.model.Allergey; import medical.com.medicalApplication.model.MedicalRecord; import medical.com.medicalApplication.model.Medication; import medical.com.medicalApplication.model.Patient; import medical.com.medicalApplication.model.Treatment; import medical.com.medicalApplication.services.MedicalRescordService; /** * * This class creates the prompts for the medical application * */ public class MedicalRecordPrompt { private static List
public void mainPrompt(Scanner scanner) { int input = -1; System.out.println("Enter Patient ID:"); String patientId = scanner.next(); Patient patient = MedicalRescordService.getReference().getPatient(patientId); if (patient != null) { while (input != 0) { System.out.println("Patient: " + patient.getName()); prompt.stream().forEach(System.out::println); input = scanner.nextInt();
switch (input) { case 1: addTreatment(scanner, patient.getId()); break; case 2: addMedication(scanner, patient.getId()); break; case 3: MedicalRescordService.getReference().getMedicalRecord(patientId).getHistory().getAllTreatments() .forEach(System.out::println); break; case 4: MedicalRescordService.getReference().getMedicalRecord(patientId).getHistory().getAllMedications() .forEach(System.out::println); break; case 5: addAllergy(scanner, patient.getId()); break; case 6: MedicalRescordService.getReference().getMedicalRecord(patientId).getHistory().getAlergies() .forEach(System.out::println); break; case 0: break; default: break; } } } else { System.out.println("Patient with that ID could not be found"); } }
private void addAllergy(Scanner scanner, String patientId) { int input = -1;
while (input != 0) { System.out.println("Enter Allergy:"); String allergyName = scanner.next();
Allergey allergy = new Allergey(allergyName); MedicalRecord medicalRecord = MedicalRescordService.getReference().getMedicalRecord(patientId);
if (medicalRecord != null) { medicalRecord.getHistory().addAllergy(allergy); } else { System.err.println("Error! Medical Record is null"); }
System.out.println( "Would you like to add another Allergy? 1 for Yes 0 To return to the Medical Record Menu"); input = scanner.nextInt(); } }
public void addTreatment(Scanner scanner, String patientId) { int input = -1;
while (input != 0) { System.out.println("Enter the treatment date:"); String treatmentDate = scanner.next();
System.out.println("Enter diagnose:"); String diagnose = scanner.next();
System.out.println("Enter description:"); String description = scanner.next();
Treatment treatment = new Treatment(treatmentDate, diagnose, description); MedicalRecord medicalRecord = MedicalRescordService.getReference().getMedicalRecord(patientId);
if (medicalRecord != null) { medicalRecord.getHistory().addTreatment(treatment); } else { System.err.println("Error! Medical Record is null"); }
System.out.println( "Would you like to add another Treatment? 1 for Yes 0 To return to the Medical Record Menu"); input = scanner.nextInt(); } }
public List
while (input != 0) { System.out.println("Enter medication name:"); String name = scanner.next(); System.out.println("Enter startDate:"); String startDate = scanner.next(); System.out.println("Enter endDate:"); String endDate = scanner.next(); System.out.println("Enter dose:"); String dose = scanner.next();
Medication medication = new Medication(name, startDate, endDate, dose); MedicalRecord medicalRecord = MedicalRescordService.getReference().getMedicalRecord(patientId);
if (medicalRecord != null) { medicalRecord.getHistory().addMedication(medication); } else { System.err.println("Error! Medical Record is null"); }
System.out.println( "Would you like to add another Medication? 1 for Yes 0 To return to the Medical Record Menu"); input = scanner.nextInt(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
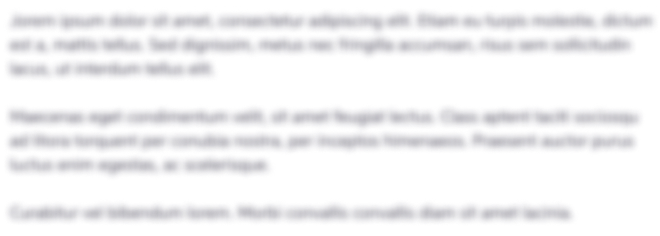
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started