Question
Need Help With Client Please !!! (Stack&Queue Package ) LinkedQueue.java package stackAndQueuePackage; public class LinkedQueue implements QueueInterface { private Node firstNode; // references node at
Need Help With Client Please !!!
(Stack&Queue Package)
LinkedQueue.java
package stackAndQueuePackage; public class LinkedQueue
lastNode = newNode; } // end enqueue
public T getFront() { T front = null; if (!isEmpty()) front = firstNode.getData(); return front; } // end getFront
public T dequeue() { T front = null; if (!isEmpty()) { front = firstNode.getData(); firstNode = firstNode.getNextNode(); if (firstNode == null) lastNode = null; } // end if return front; } // end dequeue public boolean isEmpty() { return (firstNode == null) && (lastNode == null); } // end isEmpty public void clear() { firstNode = null; lastNode = null; } // end clear
private class Node { private T data; // entry in queue private Node next; // link to next node
private Node(T dataPortion) { data = dataPortion; next = null; } // end constructor private Node(T dataPortion, Node linkPortion) { data = dataPortion; next = linkPortion; } // end constructor
private T getData() { return data; } // end getData
private void setData(T newData) { data = newData; } // end setData
private Node getNextNode() { return next; } // end getNextNode private void setNextNode(Node nextNode) { next = nextNode; } // end setNextNode } // end Node } // end Linkedqueue
LinkedStack.java
package stackAndQueuePackage; public class LinkedStack
public T peek() { T top = null; if (topNode != null) top = topNode.getData(); return top; } // end peek
public T pop() { T top = null; if (topNode != null) { top = topNode.getData(); topNode = topNode.getPreviousNode(); } // end if return top; } // end pop
public boolean isEmpty() { return topNode == null; } // end isEmpty public void clear() { topNode = null; } // end clear
private class Node { private T data; // entry in stack private Node previous; // link to previous node
private Node(T dataPortion) { data = dataPortion; previous = null; } // end constructor
private Node(T dataPortion, Node linkPortion) { data = dataPortion; previous = linkPortion; } // end constructor
private T getData() { return data; } // end getData
private void setData(T newData) { data = newData; } // end setData
private Node getPreviousNode() { return previous; } // end getPreviousNode
private void setPreviousNode(Node previousNode) { previous = previousNode; } // end setPreviousNode } // end Node } // end LinkedStack
QueueInterface.java
package stackAndQueuePackage; /** An interface for the ADT queue. @author Frank M. Carrano @version 3.0 */ public interface QueueInterface
/** Removes and returns the entry at the front of this queue. @return either the object at the front of the queue or, if the queue is empty before the operation, null */ public T dequeue();
/** Retrieves the entry at the front of this queue. @return either the object at the front of the queue or, if the queue is empty, null */ public T getFront();
/** Detects whether this queue is empty. @return true if the queue is empty, or false otherwise */ public boolean isEmpty();
/** Removes all entries from this queue. */ public void clear(); } // end QueueInterface
StackInterface.java
package stackAndQueuePackage; /** An interface for the ADT stack. @author Frank M. Carrano @version 3.0 */ public interface StackInterface
Tree Package
BinaryNode.java
package treePackage; /** A class that represents nodes in a binary tree. @author Frank M. Carrano @version 3.0 */ class BinaryNode
public BinaryNode() { this(null); // call next constructor } // end default constructor
public BinaryNode(T dataPortion) { this(dataPortion, null, null); // call next constructor } // end constructor
public BinaryNode(T dataPortion, BinaryNode
public T getData() { return data; } // end getData
public void setData(T newData) { data = newData; } // end setData
public BinaryNodeInterface
public BinaryNodeInterface
public void setLeftChild(BinaryNodeInterface
public void setRightChild(BinaryNodeInterface
public boolean hasLeftChild() { return left != null; } // end hasLeftChild
public boolean hasRightChild() { return right != null; } // end hasRightChild
public boolean isLeaf() { return (left == null) && (right == null); } // end isLeaf
public BinaryNodeInterface
if (left != null) newRoot.left = (BinaryNode
public int getHeight() { return getHeight(this); // call private getHeight } // end getHeight
private int getHeight(BinaryNode
if (node != null) height = 1 + Math.max(getHeight(node.left), getHeight(node.right)); return height; } // end getHeight
public int getNumberOfNodes() { int leftNumber = 0; int rightNumber = 0;
if (left != null) leftNumber = left.getNumberOfNodes(); if (right != null) rightNumber = right.getNumberOfNodes(); return 1 + leftNumber + rightNumber; } // end getNumberOfNodes } // end BinaryNode
BinaryNodeInterface.java
package treePackage; /** An interface for a node in a binary tree. @author Frank M. Carrano @version 3.0 */ interface BinaryNodeInterface
/** Sets the data portion of this node. @param newData the data object */ public void setData(T newData);
/** Retrieves the left child of this node. @return the node that is this nodes left child */ public BinaryNodeInterface
/** Retrieves the right child of this node. @return the node that is this nodes right child */ public BinaryNodeInterface
/** Sets this nodes left child to a given node. @param leftChild a node that will be the left child */ public void setLeftChild(BinaryNodeInterface
/** Sets this nodes right child to a given node. @param rightChild a node that will be the right child */ public void setRightChild(BinaryNodeInterface
/** Detects whether this node has a left child. @return true if the node has a left child */ public boolean hasLeftChild();
/** Detects whether this node has a right child. @return true if the node has a right child */ public boolean hasRightChild();
/** Detects whether this node is a leaf. @return true if the node is a leaf */ public boolean isLeaf();
/** Counts the nodes in the subtree rooted at this node. @return the number of nodes in the subtree rooted at this node */ public int getNumberOfNodes();
/** Computes the height of the subtree rooted at this node. @return the height of the subtree rooted at this node */ public int getHeight();
/** Copies the subtree rooted at this node. @return the root of a copy of the subtree rooted at this node */ public BinaryNodeInterface
BinaryTree.java
package treePackage; import java.util.Iterator; import java.util.NoSuchElementException; import stackAndQueuePackage.*;
/** A class that implements the ADT binary tree. @author Frank M. Carrano @version 3.0 */ public class BinaryTree
public BinaryTree() { root = null; } // end default constructor
public BinaryTree(T rootData) { root = new BinaryNode
public BinaryTree(T rootData, BinaryTree
public void setTree(T rootData) { root = new BinaryNode
public void setTree(T rootData, BinaryTreeInterface
private void privateSetTree(T rootData, BinaryTree
if ((leftTree != null) && !leftTree.isEmpty()) root.setLeftChild(leftTree.root); if ((rightTree != null) && !rightTree.isEmpty()) { if (rightTree != leftTree) root.setRightChild(rightTree.root); else root.setRightChild(rightTree.root.copy()); } // end if
if ((leftTree != null) && (leftTree != this)) leftTree.clear(); if ((rightTree != null) && (rightTree != this)) rightTree.clear(); } // end privateSetTree
private BinaryNode
public T getRootData() { T rootData = null;
if (root != null) rootData = root.getData(); return rootData; } // end getRootData
public boolean isEmpty() { return root == null; } // end isEmpty
public void clear() { root = null; } // end clear
protected void setRootData(T rootData) { root.setData(rootData); } // end setRootData
protected void setRootNode(BinaryNodeInterface
protected BinaryNodeInterface
public int getHeight() { return root.getHeight(); } // end getHeight
public int getNumberOfNodes() { return root.getNumberOfNodes(); } // end getNumberOfNodes
public Iterator
public Iterator
public Iterator
private class PreorderIterator implements Iterator
private class InorderIterator implements Iterator
public InorderIterator() { nodeStack = new LinkedStack
public boolean hasNext() { return !nodeStack.isEmpty() || (currentNode != null); } // end hasNext
public T next() { BinaryNodeInterface
// find leftmost node with no left child while (currentNode != null) { nodeStack.push(currentNode); currentNode = currentNode.getLeftChild(); } // end while
// get leftmost node, then move to its right subtree if (!nodeStack.isEmpty()) { nextNode = nodeStack.pop(); assert nextNode != null; // since nodeStack was not empty // before the pop currentNode = nextNode.getRightChild(); } else throw new NoSuchElementException();
return nextNode.getData(); } // end next
public void remove() { throw new UnsupportedOperationException(); } // end remove } // end InorderIterator
private class PostorderIterator implements Iterator
BinaryNodeInterface
public void remove() { throw new UnsupportedOperationException(); } // end remove } // end PostorderIterator private class LevelOrderIterator implements Iterator
if (rightChild != null) nodeQueue.enqueue(rightChild); } else { throw new NoSuchElementException(); } return nextNode.getData(); } // end next public void remove() { throw new UnsupportedOperationException(); } // end remove } // end LevelOrderIterator } // end BinaryTree
BinaryTreeInterface.java
package treePackage; /** An interface for the ADT binary tree. @author Frank M. Carrano @version 3.0 */ public interface BinaryTreeInterface
/** Sets an existing binary tree to a new binary tree. @param rootData an object that is the data in the new trees root @param leftTree the left subtree of the new tree @param rightTree the right subtree of the new tree */ public void setTree(T rootData, BinaryTreeInterface
TreeInterface.java
package treePackage; /** An interface for the ADT tree. @author Frank M. Carrano @version 3.0 */ public interface TreeInterface
TreeIteratorInterface.java
package treePackage; import java.util.Iterator; /** An interface of iterators for the ADT tree. @author Frank M. Carrano @version 3.0 */ public interface TreeIteratorInterface
ClientBinaryTree.java
import java.util.Iterator;
import treePackage.*;
public class ClientBinaryTree {
public static void createTree(BinaryTree
// Create the nodes at level 3
// Create the nodes at level 4
// Set values to the leaves at level 4 level4Node1.setTree("F", null, null); // no children // Set values to the nodes at level 3
// Set values to the nodes at level 2 level2Node1.setTree("B", level3Node1, level3Node2);
// Set values to the root at level 1
} public static void traverseTree(BinaryTree
System.out.println(" Preorder traversal:"); System.out.println("Expected: A B D E F G C H"); System.out.print("Actual: "); iter = rootNode.getPreorderIterator(); while (iter.hasNext()) { System.out.print(iter.next() + " "); } System.out.println();
System.out.println(" Postorder traversal:"); System.out.println("Expected: D F G E B H C A "); System.out.print("Actual: "); iter = rootNode.getPostorderIterator(); while (iter.hasNext()) { System.out.print(iter.next() + " "); } System.out.println();
System.out.println(" Level order traversal:"); System.out.println("Expected: A B C D E H F G "); System.out.print("Actual: "); iter = rootNode.getLevelOrderIterator(); while (iter.hasNext()) { System.out.print(iter.next() + " "); } System.out.println(); }
public static void main(String[] args) {
BinaryTree
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
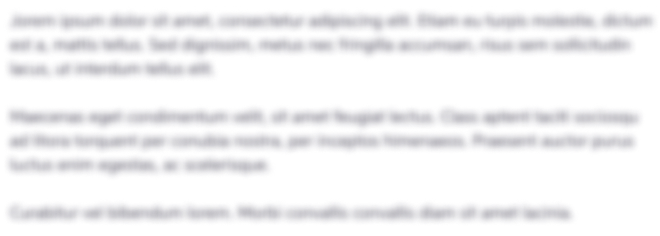
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started