Question
Need help with debugging code! Int Array Play In this lab, we review and practice arrays and functions. You will practice: How to use array
Need help with debugging code!
Int Array Play
In this lab, we review and practice arrays and functions. You will practice:
- How to use array to store a collection of values:
- partially filled array (using a int variable to keep track of the number of elements)
- searching for a value in the array,
- inserting an element into the array,
- deleting an element from the array,
- How to clearly declare a function by writing concise function comments, and specifying properly name, parameters and return type for the function. This step address the question of what the function is supposed to do?
- Passing array as parameter to function: both the array and the number of elements need to be passed to the function
- Call-by-value and call-by-reference
- return type: for functions that perform some kind of checking, it should return bool type.
- How to implement a function given its declaration. This step takes care of How the function works? When implementing a function, all you need to know is the function's declaration.
Example Output:
Enter a list of up to 20 integers or -1 to end the list 3 2 4 8 12 5 6 9 0 11 10 13 -1 3 2 4 8 12 5 6 9 0 11 10 13 Enter a value and a position to insert: 18 5 3 2 4 8 12 18 5 6 9 0 11 10 13 Enter a value to delete from the array: 18 3 2 4 8 12 5 6 9 0 11 10 13 Enter a value to append: 15 3 2 4 8 12 5 6 9 0 11 10 13 15
Please fill in the code in all places where there have a ToDo comment.
Hints
- Start with the simiplest function, e.g., the one to search the content of an array.
- The suggested order to write the function is:
- Function to search for a value in the array
- Function to fill an array with positive integers
- Function to delete an element from the array: after deletion, the array should have no holes.
- Function to insert an element into the array
- Write one function, test it to make sure it really works, and then move on to next function.
- Write comments to 1) document your algorithms and design, 2) make your code readable, 3) debug code.
Here is the code;
#include
using namespace std;
const int CAPACITY = 20;
// [Already implemented]
// displayArray - display the array on a single line separated by blanks.
// @param: int array[] is an unordered array of integers
// @param: int numberOfElements
// [Already implemented]
void displayArray(int array[], int numElements);
// ToDo: Declare a function fillArray that fills an int array with values
// entered
// by the user. Stop reading when the user inputs -1 or you reach CAPACITY.
// fillArray - reads a list of positive integers ending with -1 or until
// CAPACITY.
// @param: int array[] is an unordered array of integers when leaving this
// function
// @param: int& numberElements is the number of Elements in the array after
// function
// @returns void.
void fillArray(int array[], int &numberElements);
// ToDo: Delcare a function that removes (i.e., deletes) the element
// removeElement - removes the element of the given index from the given array.
// @param: int array[] is an unordered array of integers
// @param: int& numberElements
// @param: int position of element to delete
// @returns: true if delete was successful, false otherwise
bool removeElement(int array[], int &numberElements, int position);
// ToDo: Delcare a function that inserts the element in the given position
// insertElement - removes the element of the given index from the given array.
// @param: int array[] is an unordered array of integers
// @param: int& numberElements
// @param: int position to insert into
// @param: int target to insert.
// @returns: true if insert was successful, false otherwise
bool insertElement(int array[], int &numberElements, int position, int target);
// ToDo: Declare a funcxtion that searches for an element in the given array
// searchElement - searches for the element in the given array.
// @param int array[] is an unordered array of integers
// @param int numberOfElements
// @param int element
// @returns index of element or -1 if not found.
int searchElement(int array[], int numberOfElements, int element);
// ToDo: Declare a function that reerses the array given an array and it's size.
// @param int array[] is an unordered array of integers
// @param int numberOfElements
// @returns void, but array is reversed from the original order.
// MUST USE a version of swap that you've implemented.
void reverse(int array[], int numberOfElements);
int main() {
// The NumArray can be partially filled, we use variable NumArrayElems to keep
// track of how many numbers
// have been stored in the array.
int NumArray[CAPACITY]; // an int array with a given CAPACITY
int NumArrayElems =
0; // the array is initially empty, i.e., contains 0 elements
// 1. ToDo: Call your fillArray function to read in a sequence of integer
// values, separated by space, and ending with -1. Store the values in the
// NumArray array and the number of elements in NumArrayElems.
// Display the contents of the array afterwards
fillArray(NumArray, NumArrayElems);
displayArray(NumArray, NumArrayElems);
// 2. ToDo: Read in a value and position from the user. Call your
// insertElement function
// to insert the given value into the given position of the array
// Display the contents of the array afterwards
int position, value;
cout
cin >> value >> position;
insertElement(NumArray, NumArrayElems, position, value);
displayArray(NumArray, NumArrayElems);
// 3. ToDo: Read in a value and call your searchElement function.
cout
cin >> value;
position = searchElement(NumArray, NumArrayElems, value);
// 4. if the value is found, delete it from the array using your
// deleteElement function
// if the value not found, print "Value not found!"
// Display the contents of the array afterwards
if (position != -1)
removeElement(NumArray, NumArrayElems, position);
else
cout
displayArray(NumArray, NumArrayElems);
// 5. TODO: Read in a value and call your insertElement function to append
// a value to the end of the array
// Display the contents of the array afterwards
cout
cin >> value;
insertElement(NumArray, NumArrayElems, NumArrayElems, value);
displayArray(NumArray, NumArrayElems);
// 6. TODO: Call your reverse function to reverse the values in the array.
// Display the contents of the array.
reverse(NumArray, NumArrayElems);
//displayArray(NumArray, NumArrayElems);
return 0;
}
void displayArray(int array[], int numElements) {
for(int i=0; i
cout
}
cout
}
void fillArray(int array[], int &numberElements) {
cout
int value;
int i=0;
cin>>value;
while(value!=-1 && i
array[i] = value;
cin>>value;
i++;
}
numberElements = i;
}
bool removeElement(int array[], int &numberElements, int position) {
for(int i=position; i
array[i] = array[i+1];
}
return numberElements--;
}
bool insertElement(int array[], int &numberElements, int position, int target) {
for(int i=numberElements-1; i>=position;i--){
array[i+1] = array[i];
}
array[position] = target;
return numberElements++;
}
int searchElement(int array[], int numberOfElements, int element) {
for(int i =0;i
if(array[i]==element){
return i;
}
}
return -1;
}
void swap(int *el1, int *el2){
int temp = *el1;
*el1 = *el2;
*el2 = temp;
}
void reverse(int array[], int numberOfElements) {
int i=0;
while(i
swap(&array[i], &array[numberOfElements-i-1]);
i++;
}
} Here is the DEBUG INFO;
COMPILER STACK TRACE None PROGRAM EXECUTION STACK TRACE None INPUT OF THE TEST CASE 1 8 9 7 6 5 4 3 2 1 0 -1 2 11 -2 10 4 -1 5 YOUR CODE'S OUTPUT 1 Enter a list of up to 20 integers or -1 to end the list 2 8 9 7 6 5 4 3 2 1'0 3 Enter a value and a position to insert: 11 8 9 7 6 5 4 3 2 1 0 Enter a value to delete from the array: Value not found! 11 8 9 7 6 5 4 3 2 1 0 6 Enter a value to append: 11 8 9 7 6 5 4 3 2 1 0 -1 7 -1 0 1 2 3 4 5 6 7 9 8 11 8 THE CORRECT OUTPUT OF THE TEST CASE 1 Enter a list of up to 20 integers or -1 to end the list 2 8 9 7 6 5 4 3 2 1 0 3 Enter a value and a position to insert: 8 9 7 6 5 4 3 2 1 0 4 Enter a value to delete from the array: Value not found! 5 Enter a value to append: 8 9 7 6 5 4 3 2 1 0 -1 UNIX DIFF OF CORRECT OUTPUT AND YOUR OUTPUT 3c3 Enter a value to append: 8 9 7 6 5 4 3 2 1 0 -1 PRETTY DIFF This diff is colored to make it clear what parts of the output are wrong. Green indicates things in the correct output that you are missing, red indicates things in your output that shouldn't be there. The character refers to newlines, so the green character refers a newline you are missing in your output and the red refers to a newline you need to remove from your output. 1 Enter a list of up to 20 integers or -1 to end the list- 2 8 9 7 6 5 4 3 2 1' 0 - Enter a value and a position to insert: 11 8 9 7 6 5 4 3 2 1 0 - Enter a value to delete from the array: Value not found !-- 11 8 9 7 6 5 4 3 2 1 0 - Enter a value to append: 11 8 9 7 6 5 4 3 2 1 0 -1 - 7 -1 0 1 2 3 4 5 6 7 9 8 11 8 DEBUG INFO Your instructor has chosen to give you the following information to help you debug your code and improve your submission COMPILER STACK TRACE None PROGRAM EXECUTION STACK TRACE None INPUT OF THE TEST CASE 1 36 21 39 4 8 17 22 65 43 -1 12 12 36 4 57 5 YOUR CODE'S OUTPUT 1 Enter a list of up to 20 integers or -1 to end the list 2 36 21 39 4 8 17 22 65 43 3 Enter a value and a position to insert: 36 21 39 4 8 17 22 65 430 Enter a value to delete from the array: 21 39 4 8 17 22 65 43 0 5 Enter a value to append: 21 39 4 8 17' 22 65 43 0 57 6 57 0 43 65 22 17 8 4 39 21 THE CORRECT OUTPUT OF THE TEST CASE 1 Enter a list of up to 20 integers or -1 to end the list 2 36 21 39 4 8 17 22 65 43 3 Enter a value and a position to insert: 36 21 39 4 8 17 22 65 43 4 Enter a value to delete from the array: 2139 4 8 17 22 65 43 5 Enter a value to append: 21 39 4 8 17' 22 65 43 57 6 UNIX DIFF OF CORRECT OUTPUT AND YOUR OUTPUT 3,603,5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
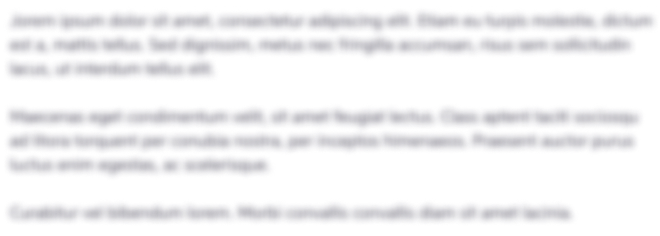
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started