Question
Need this lab for computer science done please! I've included all the java files below :) List.java: /** List ADT */ public interface List {
Need this lab for computer science done please! I've included all the java files below :)
List.java:
/** List ADT */ public interface List
Node.java
/** * Node class for Linked List */ public class Node{ private E element; // Value for this node private Node next; // reference to next node in list /** Constructor * @param item the element to be stored in Node * @param nextVal the next Node that this is pointing to */ public Node(E item, Node nextval) { element = item; next = nextval; } /** Constructor * @param item the element to be stored in Node */ public Node(E item){ element = item; next = null; } //other constructors public Node(){ element = null; next = null; } public Node(Node nextval) { next = nextval; } /** *@return the Node that is next to this */ public Node getNext() { return next; } /** * Sets this next to the given Node * @param nextNal the Node that is to be set to this Node's next */ public void setNext(Node nextval){ next = nextval; } /** * returns the element in the Node *@return element in the Node */ public E getElement() { return element; } /** * sets the element stored in Node to the element given *@param item the element to be stored in Node. */ public E setElement(E item) { return element = item; } }
MakeItASingle.java
import java.util.*; import java.lang.*; /**This is an ugly driver that is just checking methods*/ public class MakeItASingle{ public static void main(String[] args){ System.out.println("Single Linked"); playWithSingleString(); System.out.println("-----------------------------------"); } public static void playWithSingleString(){ SLListImplement a List Interface for a Single-Linked List. Download List.java, Node.java, and Make ItSingle.java Open the file List.java. This file contains an interface that describes the List ADT that you will implement. You will need to use this interface to implement a single-linked list. So you will need to implement the following class: public class SLListStringList = new SLList (); System.out.println("Empty list:"); System.out.println(StringList.toString()); StringList.add("A"); System.out.println("Added one thing"); System.out.println(StringList.toString()); System.out.println("The head of the list has: "+ StringList.getHead().getElement()); System.out.println("The tail of the list has: "+ StringList.getLast().getElement()); StringList.add("C"); System.out.println("Added another thing"); System.out.println(StringList.toString()); System.out.println("The head of the list has: "+ StringList.getHead().getElement()); System.out.println("The tail of the list has: "+ StringList.getLast().getElement()); StringList.insert(1, "B"); System.out.println("inserting something in index 1"); System.out.println(StringList.toString()); System.out.println("The head of the list has: "+ StringList.getHead().getElement()); System.out.println("The tail of the list has: "+ StringList.getLast().getElement()); StringList.insert(0, "This is the alphabet"); System.out.println("inserting something in index 0 - so a new head"); System.out.println(StringList.toString()); System.out.println("inserting something at the end - so a new tail"); StringList.insert(StringList.length(),"this is the end"); System.out.println(StringList.toString()); String whatIsThere = StringList.getValue(1); System.out.println("What is in index 1: " + whatIsThere); String whatCameBefore = StringList.prev(1); System.out.println("What came before index 1: "+ whatCameBefore); String whatCameAfter = StringList.next(1); System.out.println("What came after index 1: "+ whatCameAfter); System.out.println("The length of your list is: "+ StringList.length()); StringList.reverse(); System.out.println("reversed: "+StringList.toString()); System.out.println("the length of the list is: "+ StringList.length()); SLList StringList2 = new SLList (); StringList2.add("*"); StringList2.add("*"); System.out.println("The list to insert looks like: "+StringList2.toString()); System.out.println("the length of the inserted list is: "+ StringList2.length()); StringList.insertList(StringList2, 1); System.out.println("inserted another list at after index 1"); System.out.println(StringList.toString()); System.out.println("the length of the list is: "+ StringList.length()); System.out.println("The head of the list has: "+ StringList.getHead().getElement()); System.out.println("The tail of the list has: "+ StringList.getLast().getElement()); SLList StringList3 = new SLList (); System.out.println("the length of the inserted list is: "+ StringList3.length()); StringList.insertList(StringList3, 1); System.out.println("List after trying to insert an empty list: "+StringList.toString()); System.out.println("Removing the thing at index 3"); StringList.remove(3); System.out.println(StringList.toString()); StringList3.add("Q"); StringList3.add("U"); System.out.println("The list to insert looks like: "+StringList3.toString()); StringList.insertList(StringList3, StringList.length()-1); System.out.println("Adding a list to the end"); System.out.println(StringList.toString()); System.out.println("The head of the list has: "+ StringList.getHead().getElement()); System.out.println("The tail of the list has: "+ StringList.getLast().getElement()); StringList.clear(); System.out.println("clear the list and print"); System.out.println(StringList.toString()); System.out.println("The length of your list is: "+ StringList.length()); StringList.add("Z"); StringList.add("X"); System.out.println("Added two things"); System.out.println(StringList.toString()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
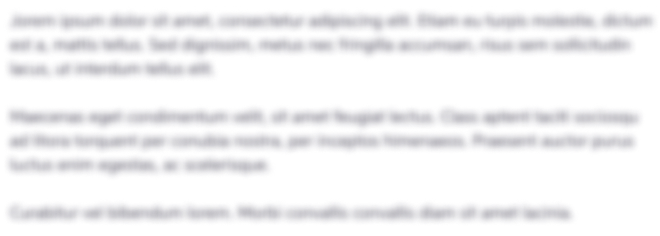
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started