Question
Need to add ainterger in List called Length (ive got that part done), this will keep track of the elements we have in the list.
Need to add ainterger in List called Length (ive got that part done), this will keep track of the elements we have in the list. Modify function printNodes, add a statement at the beginning telling how many elements we have in a list base on length. In list traverse the list and incrememnts of the value every node by 1, need to put a test for this case in main. Add a public method "Node searchValue(int val) "i have done this". In this it needs to traverse the list, search the nodes for value passed in its parament. If found should return reference to the node, if not should return null. Add a test in the main class TestList for this, if value returned by method is null, output a message saying it was not found, returned referenced is not null out put a message saying value is in the list. Add method boolean removeValue(Int val) in class List, should look through list for first node contaiing value, if node found, should remove it and return true, not found should return false. List contains multiple value should only remove first one.
THIS IS MY CODE SO FAR
List.Java public class List { public Node head; int length; //public constructor List() { head = null; length = 0; } // Inserts the value at the front of the list public void insertFront(int val){ Node n = new Node(val); n.next = head; head = n; } // Inserts a new value into a sorted list in ascending order public void insertOrder(int val) { if (head == null || val <= head.value) insertFront(val); else { Node temp = head; Node n = new Node(val); while (temp.next != null && temp.next.value < val) temp = temp.next; n.next = temp.next; temp.next = n; } } public Node searchValue(int val) { } // removes the front node of the list // returns false if the list was empty, true otherwise public boolean removeFront() { if (head == null) return false; else { head = head.next; return true; } } // removes the second node of the list // returns false if the list was empty, true otherwise public boolean removeSecond() { if (head == null || head.next == null) return false; else { Node temp = head; temp.next = temp.next.next; return true; } } // outputs all the nodes in the list public void printNodes() { Node temp = head; Node prev = null; while (temp != null) { if(temp == head) head = head.next; else prev.next = temp.next; System.out.println(temp.value); prev = prev.next = temp.next; temp = temp.next; } } // returns the count of the nodes in the list public int countNodes() { Node n = head; int count = 0; while (n != null) { ++count; n = n.next; } return count; } // returns a reference to the last node in the list public Node lastNode() { if (head == null) return null; else { Node last = head; while (last.next != null) last = last.next; return last; } } }
import java.util.Scanner;
public class TestList {
static boolean quit = false; static Scanner scan = new Scanner(System.in); public static void quickTest1(List aList) { aList.insertOrder(5); aList.insertOrder(1); aList.insertOrder(7); aList.insertOrder(4); aList.insertOrder(6); aList.printNodes(); } public static void quickTest(List aList) { aList.insertFront(5); aList.insertFront(4); aList.insertFront(7); aList.insertFront(1); aList.printNodes(); System.out.println("The list has " + aList.countNodes() + " nodes"); System.out.println("The last node in the list contains " + aList.lastNode().value); aList.removeFront(); aList.printNodes(); System.out.println("Removing the second node:"); aList.removeSecond(); aList.printNodes(); } //Interface options public static void printMenu() { System.out.println("Possible actions: "); System.out.println("0 = Quit the Program"); System.out.println("1 - Insert a value at the front"); System.out.println("2 - Insert a value in order"); System.out.println("3 - Remove the front node"); System.out.println("4 - Remove the second node"); System.out.println("5 - Count the nodes"); System.out.println("6 - Print all the nodes"); System.out.println("7 - Print the value in the last node"); } public static void performAction(List aList, int choice) { int val; switch(choice) { case 0: quit = true; break; case 1: System.out.println("Enter a value to insert in the list: "); val = scan.nextInt(); aList.insertFront(val); break; case 2: System.out.println("Enter a value to insert in the list: "); val = scan.nextInt(); aList.insertOrder(val); case 3: if(aList.removeFront()) System.out.println("The first node removed successfully!"); else System.out.println("Unable to perform the action"); break; case 4: if(aList.removeSecond()) System.out.println("The Second node removed successfully!"); else System.out.println("Unable to perform the action"); break; case 5: System.out.println("The list has " + aList.countNodes() + " nodes"); break; case 6: aList.printNodes(); break; case 7: System.out.println("The last node in the list contains " + aList.lastNode().value); break; default: System.out.println("Unknown option, try again."); } } public static void main(String[] args) { int choice; List myList = new List(); //quickTest1(myList); while(!quit) { printMenu(); System.out.println("Enter your choice"); choice = scan.nextInt(); performAction(myList, choice); } System.out.println("Thanks for playing, bye!"); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
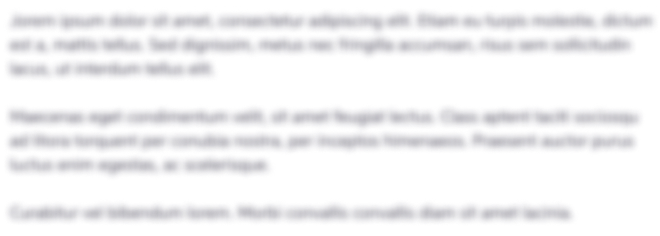
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started