Question
Need to add reveal all trolls when gameover with comments for each line of code. public class TreasureGameGUI { public static void main(String[] args) {
Need to add reveal all trolls when gameover with comments for each line of code.
public class TreasureGameGUI { public static void main(String[] args) { new TreasureGame().play(); //calling play method in treasure game class } }
import java.awt.BorderLayout; //border layout package for borders import java.awt.Dimension; //dimension package for dimension of board import java.awt.event.ActionEvent; //action event class for actions done import java.awt.event.ActionListener; //action listener for listening to action event import javax.swing.JFrame; //for j frames import javax.swing.JLabel; //for j labels on frames import javax.swing.SwingConstants; //for swing constants used for positioning public class TreasureGame extends JFrame { protected static final int NUM_TRIES = 50; //variable for max number of tries protected static final int NUM_TREASURES = 20; //variable for max number of treasures private static final String lastMove = "Last move: %s"; //variable to store last move private JLabel lastMoveLbl; //object named lastmoveLbl private StatsPanel statsPanel; //object named stats panel private TreasureBoardPanel boardPanel; //object named board panel private boolean gameOver; //game over variable for true or false public TreasureGame() { super("Treasure Hunt Game"); //sending string to super class setLayout(new BorderLayout()); //setting layout by calling object for borderlayout class setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //method used to specify one of several options for close button setVisible(true); //method to set the visibility } public void play() { gameOver = false; //setting gameover variable to false initially addComponents(); //calling add components method pack(); //calling pack method } private void addComponents() { JLabel headerLbl = new JLabel("Treasure Hunt"); //headerlbl object sending treasure hunt string to constructor headerLbl.setPreferredSize(new Dimension(60, 40)); //sets sizes of screen headerLbl.setHorizontalAlignment(SwingConstants.CENTER); //display area for a short string or image add(headerLbl, BorderLayout.NORTH); //add method to add theo object headerlbl onto the north side of the screen statsPanel = new StatsPanel(); /ew stats panel object add(statsPanel, BorderLayout.WEST); //add stats panel to the west side boardPanel = new TreasureBoardPanel(this); /ew treasure board panel object add(boardPanel, BorderLayout.CENTER); //adds board panel to the center lastMoveLbl = new JLabel(String.format(lastMove, "")); //getting the last move into lastmovelbl object lastMoveLbl.setPreferredSize(new Dimension(60, 40)); //setting lastmovelbl dimensions lastMoveLbl.setHorizontalAlignment(SwingConstants.CENTER); //sets alignment of lastmovelbl add(lastMoveLbl, BorderLayout.SOUTH); //places lastmovelbl to south side } public void updateTreasureFound(boolean treasureFound) { statsPanel.updateTreasureFound(treasureFound); //update the boolean variable to statspanel object String move = ""; //declaring string move empty if (statsPanel.getTreasuresLeft() == 0) //if statspanel is at 0 { move = String.format(lastMove, "Game over - You win"); //display you win gameOver = true; //and game is over } else if (statsPanel.getTriesLeft() == 0) //if tries left is at 0 { move = String.format(lastMove, "Game over - You lose");//diplay you lost gameOver = true; // and game is over } else { if (treasureFound) move = String.format(lastMove, "Treasure"); //updates lastmove variable with treasure found else move = String.format(lastMove, "Miss"); //updates lastmove variable with miss } lastMoveLbl.setText(move); //setting lastmove variable if (gameOver && statsPanel.getTreasuresLeft() != 0) //if game is over and still have treasures left boardPanel.displayAllTreasures(); //display all treasures } public boolean isGameOver() { return gameOver; //gets if game is over } } class ButtonListener implements ActionListener { private TreasureGame game; //object for treausre game class public ButtonListener(TreasureGame game) { this.game = game; //calls game } @Override public void actionPerformed(ActionEvent ae) { if (!game.isGameOver()) //checks if game is over or not { BoardButton btn = (BoardButton) ae.getSource(); //object for board button btn.setText(btn.getDisplayText()); //call settext method if (btn instanceof TreasureButton) //is button is pressed and treasure if found game.updateTreasureFound(true); //pass true else game.updateTreasureFound(false); //or else pass false btn.setEnabled(false); //set button enabling to false } } }
import java.awt.Dimension; //dimension package import javax.swing.Box; //box package import javax.swing.BoxLayout; //boxlayout package import javax.swing.JLabel; // for jlabels import javax.swing.JPanel; //for jpanels
public class StatsPanel extends JPanel { private static final String treasuresLeftStr = "Treasures left: %d"; //string for displaying treasures left private static final String treasuresFoundStr = "Treasures found: %d"; //string for displaying treasures found private static final String triesLeftStr = "Tries left: %d"; //string for tries left private int treasuresLeft; //variable for treasures left private int treasuresFound; //variable for treasures found private int triesLeft; //variable for tries left private JLabel treasuresLeftLbl; //object named treasure left lbl private JLabel treasuresFoundLbl; //object named treasures found lbl private JLabel triesLeftLbl; //object named tries left public StatsPanel() { super(); //calls super class setPreferredSize(new Dimension(200, 100)); //sets preferred dimensions setLayout(new BoxLayout(this, BoxLayout.Y_AXIS)); // treasuresLeft = TreasureGame.NUM_TREASURES; //calls num num treasures to find amount of treasures left treasuresFound = 0; //initial treasures found triesLeft = TreasureGame.NUM_TRIES; //calls num tries to find amount treasuresLeftLbl = new JLabel(String.format(treasuresLeftStr, treasuresLeft)); /ew display for treasures left treasuresFoundLbl = new JLabel(String.format(treasuresFoundStr, treasuresFound)); /ew display for treasures found triesLeftLbl = new JLabel(String.format(triesLeftStr, triesLeft)); /ew display for tries left add(Box.createVerticalGlue()); //specify excess space above statspanel add(treasuresLeftLbl); //add treasure left display add(treasuresFoundLbl); //add treasure found display add(triesLeftLbl); //add tries left display add(Box.createVerticalGlue()); //specift excess space below stats panel } public void updateTreasureFound(boolean treasureFound) { if (treasureFound) { treasuresFound += 1; //if treasure found + 1 to treasures found treasuresFoundLbl.setText(String.format(treasuresFoundStr, treasuresFound)); //updates treasures found treasuresLeft -= 1; //if treasure found -1 to treasures left treasuresLeftLbl.setText(String.format(treasuresLeftStr, treasuresLeft)); //updates treausres left } updateTriesLeft(); //updates how many tries left } private void updateTriesLeft() { triesLeft -= 1; //1 less try each time triesLeftLbl.setText(String.format(triesLeftStr, triesLeft)); //updates tries left } public int getTreasuresLeft() { return treasuresLeft; //gets treasure left } public int getTriesLeft() { return triesLeft; //gets tries left } }
import java.awt.GridLayout; //for grid layout import java.util.Random; //for random class import javax.swing.JPanel; //for j panel package
public class TreasureBoardPanel extends JPanel { private static final int WIDTH = 10; //variable for width of panel private static final int HEIGHT = 10; //variable for height of panel private BoardButton[] buttons; //variable array for buttons private TreasureGame game; //object for treasure game public TreasureBoardPanel(TreasureGame game) { super(); //calls super class this.game = game; //sets game setLayout(new GridLayout(WIDTH, HEIGHT)); //sets layout addButtons(); //adds button method calling } private void addButtons() { this.buttons = new BoardButton[WIDTH * HEIGHT]; //sets boardbutton to specified dimensions Random r = new Random(); /ew random object for (int i = 0; i
import java.awt.Dimension; //for setting dimensions import javax.swing.JButton; //for setting buttons import javax.swing.SwingConstants; //for swing constants
public class BoardButton extends JButton { private String displayText; //string variable for display text public BoardButton() { this("O"); //setting string to O } public BoardButton(String text) { super(); //sends string to super class this.displayText = text; //display text passed setHorizontalAlignment(SwingConstants.CENTER); //place constants on the center of button setPreferredSize(new Dimension(60, 40)); //setting dimensions for buttons } public String getDisplayText() { return displayText; //gets display text } }
public class TreasureButton extends BoardButton { public TreasureButton() { super("$$$"); //sets button to $$$ } }
Expert Answer
Tejaswi answered this
Was this answer helpful?
0
0
1,560 answers
// --------- TreasureGameGUI.java - No change --------------
public class TreasureGameGUI {
public static void main(String[] args) {
new TreasureGame().play(); // calling play method in treasure game class
}
}
// ---------- TrollButton.java - New class ----------------
public class TrollButton extends BoardButton {
public TrollButton() {
super(" TROLL "); // sets button to $$$
}
}
//------------ TreasureBoardPanel.java ---------------------------
import java.awt.GridLayout;
import java.util.Random;
import javax.swing.JPanel;
public class TreasureBoardPanel extends JPanel {
private static final int WIDTH = 10; // variable for width of panel
private static final int HEIGHT = 10; // variable for height of panel
private BoardButton[] buttons; // variable array for buttons
private TreasureGame game; // object for treasure game
public TreasureBoardPanel(TreasureGame game) {
super(); // calls super class
this.game = game; // sets game
setLayout(new GridLayout(WIDTH, HEIGHT)); // sets layout
addButtons(); // adds button method calling
}
private void addButtons() {
this.buttons = new BoardButton[WIDTH * HEIGHT]; // sets boardbutton to specified dimensions
Random r = new Random(); // new random object
for (int i = 0; i
int index = r.nextInt(this.buttons.length); // variable index for random treasures
while (this.buttons[index] != null)
index = r.nextInt(this.buttons.length); // random treasure objects
this.buttons[index] = new TreasureButton(); // new treasure button objects
// the troll is always one position in away from a treasure in the horizontal and vertical directions.
if(index+1
this.buttons[index+1] = new TrollButton();
} else if (index+WIDTH
this.buttons[index+WIDTH] = new TrollButton();
}
}
for (int i = 0; i
if (this.buttons[i] == null)
this.buttons[i] = new BoardButton(); // new button object
}
for (int i = 0; i
this.buttons[i].addActionListener(new ButtonListener(game)); // action listener for button(boxes)
add(this.buttons[i]); // adds buttons(boxes)
}
}
public void displayAllTreasures() {
for (int i = 0; i
if (this.buttons[i] instanceof TreasureButton)
this.buttons[i].setText(this.buttons[i].getDisplayText()); // displays all treasures at the end of game.
}
}
}
//------------- StatsPanel.java ----------------
import java.awt.Dimension;
import javax.swing.Box;
import javax.swing.BoxLayout;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class StatsPanel extends JPanel {
// string for displaying treasures left
private static final String treasuresLeftStr = "Treasures left: %d";
// string for displaying treasures found
private static final String treasuresFoundStr = "Treasures found: %d";
// string for displaying trolls encountered
private static final String trollsEncounteredStr = "Trolls encountered: %d";
// string for tries left
private static final String triesLeftStr = "Tries left: %d";
private int treasuresLeft; // variable for treasures left
private int treasuresFound; // variable for treasures found
private int trollsEncountered; // variable for trolls encountered
private int triesLeft; // variable for tries left
private JLabel treasuresLeftLbl; // object named treasure left lbl
private JLabel treasuresFoundLbl; // object named treasures found lbl
private JLabel trollsEncounteredLbl; // object named treasures found lbl
private JLabel triesLeftLbl; // object named tries left
public StatsPanel() {
super(); // calls super class
setPreferredSize(new Dimension(200, 100)); // sets preferred dimensions
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS)); //
treasuresLeft = TreasureGame.NUM_TREASURES; // calls num num treasures to find amount of treasures left
treasuresFound = 0; // initial treasures found
trollsEncountered = 0; // initial trolls encountered
triesLeft = TreasureGame.NUM_TRIES; // calls num tries to find amount
treasuresLeftLbl = new JLabel(String.format(treasuresLeftStr, treasuresLeft)); // new display for treasures left
treasuresFoundLbl = new JLabel(String.format(treasuresFoundStr, treasuresFound)); // new display for treasures found
trollsEncounteredLbl = new JLabel(String.format(trollsEncounteredStr, trollsEncountered)); // new display for trolls encountered
triesLeftLbl = new JLabel(String.format(triesLeftStr, triesLeft)); // new display for tries left
add(Box.createVerticalGlue()); // specify excess space above statspanel
add(treasuresLeftLbl); // add treasure left display
add(treasuresFoundLbl); // add treasure found display
add(trollsEncounteredLbl); // add trolls encountered display
add(triesLeftLbl); // add tries left display
add(Box.createVerticalGlue()); // specift excess space below stats panel
}
public void updateTreasureFound(boolean treasureFound) {
if (treasureFound) {
treasuresFound += 1; // if treasure found + 1 to treasures found
treasuresFoundLbl.setText(String.format(treasuresFoundStr, treasuresFound)); // updates treasures found
treasuresLeft -= 1; // if treasure found -1 to treasures left
treasuresLeftLbl.setText(String.format(treasuresLeftStr, treasuresLeft)); // updates treausres left
}
updateTriesLeft(); // updates how many tries left
}
public void updateTrollEncountered(boolean trollEncountered) {
if (trollEncountered) {
trollsEncountered += 1;
trollsEncounteredLbl.setText(String.format(trollsEncounteredStr, trollsEncountered));
treasuresFound = 0; // lose all treasure if troll encountered
treasuresFoundLbl.setText(String.format(treasuresFoundStr, treasuresFound)); // updates treasures found
}
updateTriesLeft(); // updates how many tries left
}
private void updateTriesLeft() {
triesLeft -= 1; // 1 less try each time
triesLeftLbl.setText(String.format(triesLeftStr, triesLeft)); // updates
// tries
// left
}
public int getTreasuresLeft() {
return treasuresLeft; // gets treasure left
}
public int getTriesLeft() {
return triesLeft; // gets tries left
}
}
// ------------- TreasureGame.java ----------------------
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.SwingConstants;
public class TreasureGame extends JFrame {
protected static final int NUM_TRIES = 50; // variable for max number of tries
protected static final int NUM_TREASURES = 20; // variable for max number of treasures
private static final String lastMove = "Last move: %s"; // variable to store last move
private JLabel lastMoveLbl; // object named lastmoveLbl
private StatsPanel statsPanel; // object named stats panel
private TreasureBoardPanel boardPanel; // object named board panel
private boolean gameOver; // game over variable for true or false
public TreasureGame() {
super("Treasure Hunt Game"); // sending string to super class
setLayout(new BorderLayout()); // setting layout by calling object for borderlayout class
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // method used to specify one of several options for close button
setVisible(true); // method to set the visibility
}
public void play() {
gameOver = false; // setting gameover variable to false initially
addComponents(); // calling add components method
pack(); // calling pack method
}
private void addComponents() {
JLabel headerLbl = new JLabel("Treasure Hunt"); // headerlbl object sending treasure hunt string to constructor
headerLbl.setPreferredSize(new Dimension(60, 40)); // sets sizes of screen
headerLbl.setHorizontalAlignment(SwingConstants.CENTER); // display area for a short string or image
add(headerLbl, BorderLayout.NORTH); // add method to add theo object headerlbl onto the north side of the screen
statsPanel = new StatsPanel(); // new stats panel object
add(statsPanel, BorderLayout.WEST); // add stats panel to the west side
boardPanel = new TreasureBoardPanel(this); // new treasure board panel object
add(boardPanel, BorderLayout.CENTER); // adds board panel to the center
lastMoveLbl = new JLabel(String.format(lastMove, "")); // getting the last move into lastmovelbl object
lastMoveLbl.setPreferredSize(new Dimension(60, 40)); // setting lastmovelbl dimensions
lastMoveLbl.setHorizontalAlignment(SwingConstants.CENTER); // sets alignment of lastmovelbl
add(lastMoveLbl, BorderLayout.SOUTH); // places lastmovelbl to south side
}
public void updateTreasureFound(boolean treasureFound) {
statsPanel.updateTreasureFound(treasureFound); // update the boolean variable tostatspanel object
String move = ""; // declaring string move empty
if (statsPanel.getTreasuresLeft() == 0) // if statspanel is at 0
{
move = String.format(lastMove, "Game over - You win"); // display
// you win
gameOver = true; // and game is over
} else if (statsPanel.getTriesLeft() == 0) // if tries left is at 0
{
move = String.format(lastMove, "Game over - You lose");// diplay you
// lost
gameOver = true; // and game is over
} else {
if (treasureFound) {
move = String.format(lastMove, "Treasure"); // updates lastmove variable with treasure found
} else {
move = String.format(lastMove, "Miss"); // updates lastmove variable with miss
}
}
lastMoveLbl.setText(move); // setting lastmove variable
if (gameOver && statsPanel.getTreasuresLeft() != 0) // if game is over and still have treasures left
{
boardPanel.displayAllTreasures(); // display all treasures
}
}
public void updateTrollEncountered(boolean trollEncountered) {
statsPanel.updateTrollEncountered(trollEncountered); // update the boolean variable tostatspanel object
String move = ""; // declaring string move empty
if (statsPanel.getTreasuresLeft() == 0) // if statspanel is at 0
{
move = String.format(lastMove, "Game over - You win"); // display you win
gameOver = true; // and game is over
} else if (statsPanel.getTriesLeft() == 0) // if tries left is at 0
{
move = String.format(lastMove, "Game over - You lose");// display you lost
gameOver = true; // and game is over
} else {
if (trollEncountered) {
move = String.format(lastMove, "Troll"); // updates lastmove variable with treasure found
} else {
move = String.format(lastMove, "Miss"); // updates lastmove variable with miss
}
}
lastMoveLbl.setText(move); // setting lastmove variable
if (gameOver && statsPanel.getTreasuresLeft() != 0) // if game is over and still have treasures left
{
boardPanel.displayAllTreasures(); // display all treasures
}
}
public boolean isGameOver() {
return gameOver; // gets if game is over
}
}
class ButtonListener implements ActionListener {
private TreasureGame game; // object for treausre game class
public ButtonListener(TreasureGame game) {
this.game = game; // calls game
}
@Override
public void actionPerformed(ActionEvent ae) {
if (!game.isGameOver()) // checks if game is over or not
{
BoardButton btn = (BoardButton) ae.getSource(); // object for board button
btn.setText(btn.getDisplayText()); // call settext method
if(btn instanceof TrollButton) // is button is pressed and troll encountered
{
game.updateTrollEncountered(true);
}
if (btn instanceof TreasureButton) // is button is pressed and treasure if found
{
game.updateTreasureFound(true); // pass true
}
else {
game.updateTreasureFound(false); // or else pass false
}
btn.setEnabled(false); // set button enabling to false
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
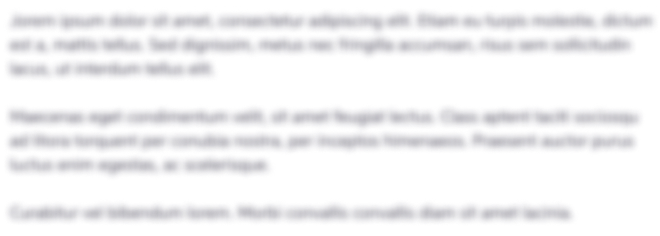
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started