Question
Need to create a Generic Set class that can handle sets of any generic type in java and a complementary SetUtilities Class that take inputs
Need to create a Generic Set class that can handle sets of any generic type in java and a complementary SetUtilities Class that take inputs of my Generic Class objects and either combine the sets objects into a new set object as a union of sets or an intersection of sets using two my class objects. Please respond with a java code with comments.
This class should implement the Comparable interface.
Note: a set is not allowed to contain duplicate values.
You can not use any methods related to Sets from the Java library.
You can not use ArrayList or any methods from the ArrayList class.
You can not use any built-in Java methods to implement your solution except the following:
Arrays.sort();
System.arraycopy();
Your class shall have a private integer data field called size.
Constructors:
class shall have a default constructor which creates an empty set with an initial capacity of 12.
Second Constructor with input of one capacity variable:
- Your class shall have a constructor which accepts one integer parameter.
- This parameter is the initial capacity of the set and should be used to initialize the size of the internal array.
Third Constructor will accept a comma separated list of generic values as a parameter.
Fourth Constructor should be a copy constructor that will make a deep copy.
Public Methods:
exists(value):
- This method shall accept a single value as a parameter.
- This method shall return true or false depending on whether or not the given value already exists within your set
add(value):
- This method shall accept a single value as a parameter.
- This method shall add the value to the set, provided that the value does not already exist
addAll(Element... values):
- This method shall accept a comma separate list of generic values as a parameter (see variable-length parameter lists).
- This method shall add all of the given values from the set, provided that the value to be added is not a duplicate.
remove(value):
- This method shall accept a single value as a parameter.
- This method shall remove the given value from the set provided that the value currently exists in the set. (Note: This is not the index to remove, but the actual value you want to remove.)
get(index):
- This method shall accept an integer parameter which is an index value.
- This method will return the value stored at the given index in the set.
size():
- This method shall take no parameters and return the size (number of elements) in the set.
- This method should be implemented with O(1) runtime.
equals(Object):
- This method shall override the equals method from the object class.
- This method shall take an object of this class as a parameter and return true if the two sets are equal, false if they are not.
toString():
- This method shall return a String representation of your set.
- If the set is empty, you may print out an empty pair of {}.
Private Methods:
resize():
- This method shall be responsible for resizing the capacity of the set when the set becomes full.
- This method shall resize the set array by creating a new array double the current capacity of the set array.
Should also create a complement class
MyClassSetUtils Class:
This class shall only contain only public static methods.
This class shall have no data fields.
This class shall have an empty no-arg (default) constructor which should have private visibility.
Public Methods:
union(MyClassObj1, MyClassObj2):
- This method shall take two myClass instances, containing elements of any data type, as parameters, and return a myClass object (not an array) which is the union of the two sets.
intersection(myClassObj1, myClassObj2):
- This method shall take two myClass instances, containing elements of any data type, as parameters, and return a myClass object (not an array)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
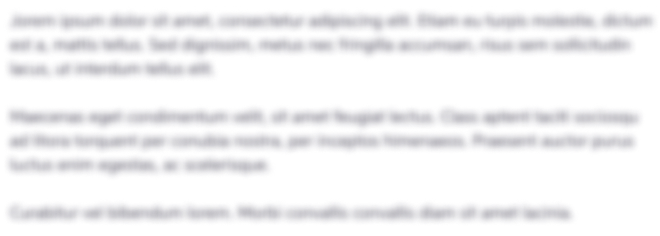
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started