Question
Need to design and implement a reliable block transfer protocol that implements the Stop-and-Wait protocol (SWAP). Some Background: The Stop-and Wait protocol uses both flow
Need to design and implement a reliable block transfer protocol that implements the Stop-and-Wait protocol (SWAP).
Some Background:
The Stop-and Wait protocol uses both flow control and error control. Both the sender and the receiver use a sliding window with a size of 1. The sender sends one packet at a time and waits for an acknowledgment before sending the next packet. To detect corrupted packets, we need to add a checksum to each data packet. To prevent duplicate packets, the protocol uses sequence numbers.
SWAP The SWAP APIs I'm using are:
1) int swap_open (unsigned int destination_address, unsigned short server_port): It is used by a SWAP client to open a reliable session to a SWAP server of the destination IP address destination_address in the network byte order with the port number.
2) int swap_wait (unsigned short server_port): It is used by a SWAP server to wait for a reliable session connection request from a SWAP client. The port number server_port is in the network byte order. It returns an identifier, called session descriptor, used for data exchange if there is no error, otherwise -1.
3) void swap_close (int socket_descriptor): It is used by both the client and the server to close the SWAP connection.
4) int swap_write (int socket_descriptor, unsigned char buffer, int buffer_length): NEED HELP TO IMPLEMENT THIS FUNCTION IN swap_client.c.
5) int swap_read(int socket_descriptor, unsigned char buffer): NEED HELP TO IMPLEMENT THIS FUNCTION IN swap_server.c.
The application I'm trying to develop also uses a protocol called Simple Datalink Protocol (SDP). I have already completed SDP. These are the APIs used in sdp.c (described below) which are used by SWAP.
SDP APIs are:
1) int sdp_receive (int sd, char *buf): It reads data and stores it in buf, from the session sd, which is returned from swap_open() or swap_wait(). The maximum size that it can receive is 256 bytes. And it returns the number of bytes read from the session sd if there is no error, -2 if the session is disconnected, and -1 for other general errors.
2) int sdp_receive_with_timer (int sd, char *buf, unsigned int expiration): It reads data and stores it in buf, from the session sd, which is returned from swap_open() or swap_wait(). expiration is used to set a timer and the unit is a millisecond. The maximum size that it can receive is 256 bytes. It returns the number of bytes read from the session sd if there is no error -3 if the timer expires, -2 if the session is disconnected, and -1 for other general errors.
3) int sdp_send (int sd, char *buf, int length): It writes data of length, which is stored in buf, to the session sd. sd is the value returned from swap_open() or swap_wait(). The maximum size that it can send is 256 bytes. It returns the number of bytes written to the session sd if there is no error, otherwise -1.
Note: The following three SWAP functions are included in the same file as the SDP functions. These SWAP functions are shared by both swap_client.c and swap_server.c.
4) int swap_connect (unsigned int IP_addrress , unsigned short server _ port ): Function to connect and send data on a socket.
5) int swap_disconnect (int socket_descriptor ): Called by swap_close(). Function to flush the contents of the receive buffer.
6) int swap_accept(unsigned int IP_addrress , unsigned short server _ port ): Function to accept data on a socket.
CHECKSUM Since SDP is a non-reliable block transfer protocol, I need to also use a checksum algorithm. (See the error checking algorithm for DATA frames as well as ACK frames for further info). I've already implemented a checksum function.
SWAP Client Implementation
In swap_client.c, there are three functions with some global variables:
1) int swap_open(unsigned int addr, unsigned short port);
2) int swap_write(int sd, char *buf, int length); // you need to design and implement
3) void swap_close(int sd);
(can use global variable S for the control variable on the SWAP client in swap_client.c)
SWAP Server Implementation
In swap_server.c, there are three functions with some global variables:
1) int swap_wait(unsigned short port);
2) int swap_read(int sd, char *buf); // you need to design and implement
3) void swap_close(int sd);
(can use the global variable R for the control variable on the SWAP server in swap_server.c)
What I Need Help With:
I've completed my source code but I need to design and implement the SWAP functions swap_read() and swap_write(). All the other SWAP functions are done (source file screenshot attached). SDP is already completely implemented in sdp.c, and a checksum function is provided in checksum.c.
My source code compiles and runs but the program functionality is incomplete. This is what I'm having trouble with:
- Completing the function swap_write() found in the file swap_client.c.
- Completing the function swap_read() found in the file swap_server.c.
- Implementing error checking for DATA frames as well as ACK frames to provide reliable communications. Need to use the checksum() function found in the file checksum.c for error checking.
- I also need to add print statements to your code to provide information about what the client and server programs are doing when they run.
The sender should send one frame at a time and wait for an acknowledgment before the next frame is sent.
Compile and Running the SWAP Client and Server Programs
I'm testing on two terminals; one terminal for the server and another terminal for the client. I'm specifying the port number and IP address as command line arguments.
#Compile the SWAP server program ---> $ gcc test_swap_server.c swap_server.c sdp.c checksum.c -o test_swap_server
# Run the SWAP server program from the command line; specify the port number ---> ./test_swap_server 8899
# Compile the SWAP client program ---> $ gcc test_swap_client.c swap_client.c sdp.c checksum.c -o test_swap_client
# Run the client program from the command line; specify the IP address and port number ---> ./test_swap_client 127.0.0.1 8899
The swap_server should print the message sent from swap_client.
SWAP CLIENT SOURCE CODE SO FAR:
#include
#include
#include
#include
#include
#include
#define MAXLINE 128 // maximum characters to receive and send at once
#define MAXFRAME 256
extern int swap_connect(unsigned int addr, unsigned short port);
extern int swap_disconnect(int sd);
extern int sdp_send(int sd, char *buf, int length);
extern int sdp_receive(int sd, char *buf);
extern int sdp_receive_with_timer(int sd, char *buf, unsigned int expiration);
int session_id = 0;
int S = 0; // frame number sent
int swap_open(unsigned int addr, unsigned short port)
{
int sockfd; // sockect descriptor
struct sockaddr_in servaddr; // server address
char buf[MAXLINE];
int len, n;
/*
* if the session is already open, then return error
*/
if (session_id != 0)
return -1;
/*
* connect to a server
*/
session_id = swap_connect(addr, port); // in sdp.o
/*
* return the seesion id
*/
return session_id;
}
int swap_write(int sd, char *buf, int length)
{
int n;
char frame[MAXFRAME];
if (session_id == 0 || sd != session_id)
return -1;
/*
* send a DATA frame
*/
// ...
/*
* read a frame with a timer
*/
// ...
/*
* several different cases including disconnection
*/
// ...
/*
* return the length sent
*/
// ...
}
void swap_close(int sd)
{
if (session_id == 0 || sd != session_id)
return;
else
session_id = 0;
swap_disconnect(sd); // in sdp.o
}
SWAP SERVER SOURCE CODE SO FAR:
#include
#include
#include
#include
#include
#include
#define MAXLINE 128 // maximum characters to receive and send at once
#define MAXFRAME 256
extern int swap_accept(unsigned short port);
extern int swap_disconnect(int sd);
extern int sdp_send(int sd, char *buf, int length);
extern int sdp_receive(int sd, char *buf);
extern int sdp_receive_with_timer(int sd, char *buf, unsigned int expiration);
int session_id = 0;
int R = 0; // frame number to receive
int swap_wait(unsigned short port)
{
/*
* if the session is already open, then return error
*/
if (session_id != 0)
return -1;
/*
* accept a connection
*/
session_id = swap_accept(port); // in sdp.o
/*
* return a ssession id
*/
return session_id;
}
int swap_read(int sd, char *buf)
{
int n;
char frame[MAXFRAME];
if (session_id == 0 || sd != session_id)
return -1;
/*
* receive a frame without a timer
*/
// ...
/*
* several cases
*/
// ...
/*
* copy the data field in the frame into buf, and return the length
*/
// ...
}
void swap_close(int sd)
{
if (session_id == 0 || sd != session_id)
return;
else
session_id = 0;
swap_disconnect(sd); // in sdp.o
}
TEST CLIENT FILE:
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#define MAX_FTA 128
// External functions
extern int swap_open(unsigned int addr, unsigned short port);
extern int swap_write(int sd, unsigned char buf[], int length);
extern void swap_close(int sd);
// These arguments must be passed to the program
// argv[1]: IP address of server, e.g., "127.0.0.1"
// argv[2]: port number of sever, e.g., "6789"
int main (int argc, char *argv[])
{
int sd;
char buf[MAX_FTA];
int n;
int i;
if (argc < 3) {
fprintf(stderr, "Usage: %s IP_address port_numbern", argv[0]);
exit(1);
}
// connect to the swap server
sd = swap_open(inet_addr(argv[1]), htons(atoi(argv[2])));
if (sd < 0)
{
fprintf(stderr, "%s cannot swap_openn", argv[0]);
exit(1);
}
else
fprintf(stdout, "connected to the swap servern");
// send messages to the swap server
buf[0] = '0';
buf[1] = '1';
buf[2] = '2';
buf[3] = '3';
buf[4] = '4';
buf[5] = '5';
buf[6] = '6';
buf[7] = '7';
buf[8] = '8';
buf[9] = '9';
n = 20;
for (i = 0; i < n; i++)
swap_write(sd, buf, 10);
// close the connection to the swap server
swap_close(sd);
}
TEST SERVER FILE:
#include
#include
#include
#include
#include
#include
#include
#include
#define MAX_FTA 128
// External functions
extern int swap_wait(unsigned short port);
extern int swap_read(int sd, unsigned char buf[]);
extern void swap_close(int sd);
// These arguments must be passed to the program
// argv[1]: port number, e.g., "6789"
int main (int argc, char *argv[])
{
int sd, bytes_read;
char buf[MAX_FTA];
if (argc < 2) {
fprintf(stderr, "Usage: %s port_numbern", argv[0]);
exit(1);
}
sd = swap_wait(htons(atoi(argv[1])));
if (sd < 0) {
fprintf(stderr, "%s cannot wait, %dn", argv[0], sd);
exit(1);
}
// read message from the client and store them into the above file
while((bytes_read = swap_read(sd, buf)) > 0) {
buf[bytes_read] = '';
printf("%sn", buf);
}
// close the file and the connection
swap_close(sd);
}
CHECKSUM SOURCE CODE:
#include
#include
// Internet Checksum - RFC-1071
unsigned short
checksum(unsigned char buf[], int length)
{
int i;
unsigned short word16;
unsigned int sum32;
if (length % 2) // if odd
return 999;
sum32 = 0;
for (i = 0; i < length; i = i + 2) {
word16 = (buf[i] << 8) + buf[i+1];
sum32 = sum32 + word16;
if (sum32 & 0x10000)
sum32 = (sum32 & 0xffff) + 1;
}
sum32 = ~sum32;
return (sum32 & 0xffff);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
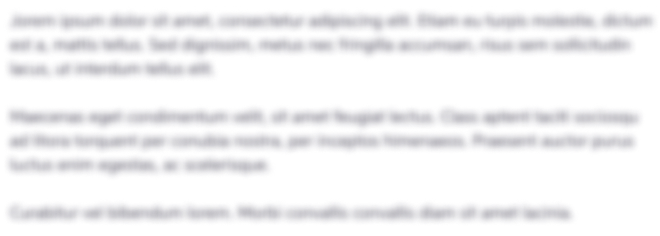
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started