Question
NEED TO FINISH THE CODE IN C++ FOR PROGRAM TO RUN (TEXT FILE IS BELOW) using namespace std; void get_input(int& month, int& day, int& year);
NEED TO FINISH THE CODE IN C++ FOR PROGRAM TO RUN
(TEXT FILE IS BELOW) using namespace std;
void get_input(int& month, int& day, int& year); // postcondition: get the input of a date. If the date is NOT valid, will prompt the user to reenter a valid date
void get_input(int& month, int& year); // postcondition: get the input of a month. If the month is NOT valid, will prompt the user to reenter a valid month
void get_input(int& year); // postcondition: get the input of a year. If the date is NOT valid, will prompt the user to reenter a valid year. // the year must be later than 1582 inorder to be valid
bool isValid(int month, int day, int year); // postcondition: return true if the specified date is valid, false otherwise
bool isValid(int month, int year); // postcondition: return if the specified month is valid, false othewise
bool isValid(int year); // postcondition: return true if the given year is valid. false otherwise // the given year is valid if it is later than year 1582
void print_calendar(int month, int day, int year); // precondition: the date is a valid date // postcondition: print out the day of week for specified date. For example, print_calendar(10, 15, 2014) will print out // October 15, 2014 is Wednesday
void print_calendar(int month, int year); // precondition: the month is a valid month, and the year is a valid year // postcondition: print out the calendar of specified month
void print_calendar(int year); // precondition: the year is a valid year // postcondition: print out the calendar of specified year
string monthName(int month); // precondition: the month value is 1, 2, .., 12 // postcondition: return a string that represents the name of the month. If month is 1, the name is "January" and so on
int daysInMonth(int month, int year); // precondition: the month and year are valid // postcondition: return the number of days in the given month in given year
void menu(); // postcondition: the menu is displayed for choose
bool isLeapYear(int year); // precondition: the year is valid // postcondition: return true if the year is leap year; false otherwise
int getCenturyValue(int year); // precondition: the year is valid // postcondition: return the century value of the given year.
int getYearValue(int year); // precondition: the year is valide // postcondition: return the year value of the given year.
int getMonthValue(int month, int year); // precondition: the month and year are valide // postcondition: return the month value of the given month in given year.
int dayOfWeek(int month, int day, int year); // precondition: the date is valid // postcondition: return 0 if the date is Sunday, 1 if the date is Monday and so on
string dayOfWeek(int day); // preconditon: day has integer value 0, 1, 2, 3, 4, 5, or 6 // postcondition: the name of the day of week is returned as a string. // If day has value 0, then SUNDAY is returned; 1, then MONDAY is returned // and so on
//************************************************************************ // You may have implemented all functions above for lab five/project five // Implementation all functions above are given in this file // They are the solution for Project Five and more. // You must implement the functions below. Also, you must change the menu // option accordingly and add one more case in main function // ***********************************************************************
bool print_calendar(int year, string fileName); // precondition: year is a leagal year, and fileName is the name of output file // postcondition: if the calendar of the given year is successfully output to the file // with name fileName, return true; false otherwise
void print_calendar(int month, int year, ofstream& output); // precondition: month is a legal month in a given legal year and output is an ofstream // that connected to a successfully opened file // postcondition: the calendar of the given month of given year is output to a file // through the output.
int main() { char choice; int day, month, year; do { system("CLS"); menu();// display menu by calling menu function cout>choice;// get user's choice switch(choice) { case '1': // will query a date. get_input(month, day, year); print_calendar(month, day, year);// print out the day of week of specified date break; case '2': // print out the calendar of a given month. get_input(month, year); print_calendar(month, year);//print out the calendar of specified month break; case '3': // print out the calendar of a given year. get_input(year); print_calendar(year);// print out the calendar of specified year break; case '4': // print out the calendar of specified year to yourname.txt file // you implement this ****************** break; case '5': // quit the program. cout
// you implement all function stubs here void menu() { cout
void get_input(int& month, int& day, int& year) { cout > month >> day >> year; while(!isValid(month, day, year)) // as long as the date is NOT valid, keep asking to reenter the date { cout > month >> day >> year; } }
void get_input(int& month, int& year) { cout > month >> year; while(!isValid(month, year)) // as long as the month is NOT valid, keep asking to reenter the month { cout > month >> year; } }
void get_input(int& year) { cout > year; while(!isValid(year)) // as long as the year is NOT valid, keep asking to reenter the year { cout > year; } }
bool isValid(int month, int day, int year) { // notice that this implementation is different from the one I talked in class // Questions 1: Please answer (YES/NO) to indicate if you understand this implementation // by adding one line of comments at beginning of the file. This worth 1 point
bool result = true; // temporary assume that the result is true switch(month) // update result based on day { case 1: case 3: case 5: case 7: case 8: case 10: case 12: result = day >= 1 && day = 1 && day = 1 && day = 1 && day
bool isValid(int month, int year) { return month >= 1 && month 1582; // month is between 1 and 12 and year is later than 1582 }
bool isValid(int year) { return year > 1582; // year is later than 1582 }
bool isLeapYear(int year) { return (year % 400 == 0 || year % 4 == 0 && year % 100 != 0); // book's instruction }
int getCenturyValue(int year) { return 2 * ( 3 - (year / 100) % 4 ) ; // book's instruction }
int getYearValue(int year) { return year % 100 + year % 100 / 4; // book's instruction }
int getMonthValue(int month, int year) // book's instruction { int result; switch(month) { case 1: if(isLeapYear(year)) result = 6; else result = 0; break; case 2: if(isLeapYear(year)) result = 2; else result = 3; break; case 3: case 11: result = 3; break; case 4: case 7: result = 6; break; case 5: result = 1; break; case 6: result = 4; break; case 8: result = 2; break; case 9:case 12: result = 5; break; default: // case 10 result = 0; break; } return result; }
int dayOfWeek(int month, int day, int year) // book's instruction { if(!isValid(month, day, year)) return -1; return (getCenturyValue(year) + getMonthValue(month, year) + getYearValue(year)+ day)%7; }
void print_calendar(int month, int day, int year) { // Questions 2: Add one line of comments at the beginning of the file to indicate // if you understand what the following if statement does? (YES/NO) // This worth 1 point
if(!isValid(month, day, year)){ // if the date is NOT valid, print out error message and return cout
void print_calendar(int month, int year) { if(!isValid(month, year)){ // if the month is NOT valid, print out error message and return cout
// print out the month and year cout
void print_calendar(int year) { if(!isValid(year)){ // if the year is NOT valid, print out error message and return cout
for(int i = 1; i
string monthName(int month) { string result; switch(month) { case 1: result = "January"; break; case 2: result = "Febraury"; break; case 3: result = "March"; break; case 4: result = "April"; break; case 5: result = "May"; break; case 6: result = "June"; break; case 7: result = "July"; break; case 8: result = "August"; break; case 9: result = "September"; break; case 10: result = "October"; break; case 11: result = "November"; break; case 12: result = "December"; break; default: result = "Not valid month"; break; } return result; }
string dayOfWeek(int day) { string result; switch(day) { case 0: result = "Sunday"; break; case 1: result = "Monday"; break; case 2: result = "Tuesday"; break; case 3: result = "Wednesday"; break; case 4: result = "Thursday"; break; case 5: result = "Friday"; break; case 6: result = "Saturday"; break; default: result = "Invalid date"; break; } return result; }
int daysInMonth(int month, int year) {
int result; switch(month) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: result = 31; break; case 4: case 6: case 9: case 11: result = 30; break; case 2: if(isLeapYear(year)) result = 29; else result = 28; break; default: result = -1; break; } return result; }
bool print_calendar(int year, string fileName) { // if year is not valid, print out error message and return false
// declare a locat ofstream variable and open file to write // if file open failed, print out error message and return false // use for loop to print out 12 months to the file
// close file
// return true }
void print_calendar(int month, int year, ofstream& output) { // if the month is NOT valid, print out error message and return // print out the month and year
// print out day names // calculate spaces before the first day of the month
// print out spaces // calculate the days in the month // print out all days, using a for loop // two more new lines
// Note this function is very similar to the one print to screen }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
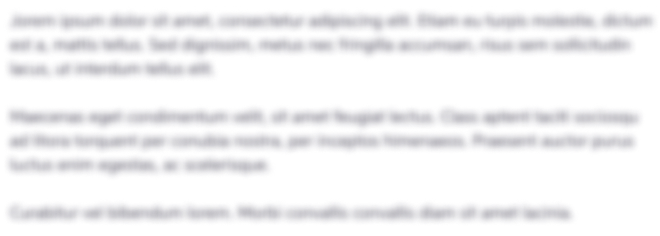
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started