Question
need to implement these methods to exisitng code import java.awt.*; import java.awt.event.*; import java.awt.image.*; import javax.imageio.*; import javax.swing.*; import javax.swing.event.*; public class DrawingPanel implements ActionListener
need to implement these methods to exisitng code
import java.awt.*; import java.awt.event.*; import java.awt.image.*; import javax.imageio.*; import javax.swing.*; import javax.swing.event.*; public class DrawingPanel implements ActionListener { public static final int DELAY = 50; // delay between repaints in millis private static final String DUMP_IMAGE_PROPERTY_NAME = "drawingpanel.save"; private static String TARGET_IMAGE_FILE_NAME = null; private static final boolean PRETTY = true; // true to anti-alias private static boolean DUMP_IMAGE = true; // true to write DrawingPanel to file private int width, height; // dimensions of window frame private JFrame frame; // overall window frame private JPanel panel; // overall drawing surface private BufferedImage image; // remembers drawing commands private Graphics2D g2; // graphics context for painting private JLabel statusBar; // status bar showing mouse position private long createTime; static { TARGET_IMAGE_FILE_NAME = System.getProperty(DUMP_IMAGE_PROPERTY_NAME); DUMP_IMAGE = (TARGET_IMAGE_FILE_NAME != null); } // construct a drawing panel of given width and height enclosed in a window public DrawingPanel(int width, int height) { this.width = width; this.height = height; this.image = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB); this.statusBar = new JLabel(" "); this.statusBar.setBorder(BorderFactory.createLineBorder(Color.BLACK)); this.panel = new JPanel(new FlowLayout(FlowLayout.CENTER, 0, 0)); this.panel.setBackground(Color.WHITE); this.panel.setPreferredSize(new Dimension(width, height)); this.panel.add(new JLabel(new ImageIcon(image))); // listen to mouse movement MouseInputAdapter listener = new MouseInputAdapter() { public void mouseMoved(MouseEvent e) { DrawingPanel.this.statusBar.setText("(" + e.getX() + ", " + e.getY() + ")"); } public void mouseExited(MouseEvent e) { DrawingPanel.this.statusBar.setText(" "); } }; this.panel.addMouseListener(listener); this.panel.addMouseMotionListener(listener); this.g2 = (Graphics2D)image.getGraphics(); this.g2.setColor(Color.BLACK); if (PRETTY) { this.g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); this.g2.setStroke(new BasicStroke(1.1f)); } this.frame = new JFrame("Building Java Programs - Drawing Panel"); this.frame.setResizable(false); this.frame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent e) { if (DUMP_IMAGE) { DrawingPanel.this.save(TARGET_IMAGE_FILE_NAME); } System.exit(0); } }); this.frame.getContentPane().add(panel); this.frame.getContentPane().add(statusBar, "South"); this.frame.pack(); this.frame.setVisible(true); if (DUMP_IMAGE) { createTime = System.currentTimeMillis(); this.frame.toBack(); } else { this.toFront(); } // repaint timer so that the screen will update new Timer(DELAY, this).start(); } // used for an internal timer that keeps repainting public void actionPerformed(ActionEvent e) { this.panel.repaint(); if (DUMP_IMAGE && System.currentTimeMillis() > createTime + 4 * DELAY) { this.frame.setVisible(false); this.frame.dispose(); this.save(TARGET_IMAGE_FILE_NAME); System.exit(0); } } // obtain the Graphics object to draw on the panel public Graphics2D getGraphics() { return this.g2; } // set the background color of the drawing panel public void setBackground(Color c) { this.panel.setBackground(c); } // show or hide the drawing panel on the screen public void setVisible(boolean visible) { this.frame.setVisible(visible); } // makes the program pause for the given amount of time, // allowing for animation public void sleep(int millis) { try { Thread.sleep(millis); } catch (InterruptedException e) {} } // take the current contents of the panel and write them to a file public void save(String filename) { String extension = filename.substring(filename.lastIndexOf(".") + 1); // create second image so we get the background color BufferedImage image2 = new BufferedImage(this.width, this.height, BufferedImage.TYPE_INT_RGB); Graphics g = image2.getGraphics(); g.setColor(panel.getBackground()); g.fillRect(0, 0, this.width, this.height); g.drawImage(this.image, 0, 0, panel); // write file try { ImageIO.write(image2, extension, new java.io.File(filename)); } catch (java.io.IOException e) { System.err.println("Unable to save image: " + e); } } // makes drawing panel become the frontmost window on the screen public void toFront() { this.frame.toFront(); }
import java.awt.*; public class Facebook{ private String name; private String content; DrawingPanel panel; private Graphics g; public Facebook(String nm){ content = "undefined"; name = nm; // Create the drawing panel panel = new DrawingPanel(200, 150); g = panel.getGraphics(); // display name g.drawString(name+"'s mood is undefined.", 20, 75); } public void setContent(String newContent){ content = newContent; if(content.equals("happy")){ g.setColor(Color.red); g.fillRect(0, 0, 200, 150); g.setColor(Color.black); // display mood g.drawString(name+"'s mood is:"+ "happy", 20, 75); } else{ g.setColor(Color.white); g.fillRect(0, 0, 200, 150); g.setColor(Color.black); g.drawString(name+"'s mood is:"+ content, 20, 75); } } public String getContent(){ return content; } }
public class FacebookPerson{ private String myName; protected String myMood; protected Facebook myfacebook; public FacebookPerson(String name){ myName = name; myfacebook = new Facebook(myName); //System.out.println("FacebookPerson's constructor"); } public FacebookPerson(){ } public String getName(){ return myName; } public void setMood(String newMood){ myMood = newMood; myfacebook.setContent(myMood); } public String getMood(){ return myMood; } }
import java.util.*; public class testFacebook{ public static void main (String[] args){ // Prompt user to enter the number of facebookpresons Scanner userInput = new Scanner(System.in); System.out.println("Enter the number of facebookpresons to be created: "); int numP=0; while(true){ try{ String inputString = userInput.nextLine(); numP = Integer.parseInt(inputString); if(numP>0 && numP
please bold the areas in where you implemented the method and it would if could explain it
Your HW5 is to modify the Facebook example so that instead of each person has its own Facebook window, all persons are displayed in the same Facebook window. Different persons are shown at different regions of the window as illustrated by the figure at the end of this document. The size of the Facebook window is 700 550 and the size of each person's region is 200 150. The program will ask the user to enter the number of facebookpresons to be created. The maximum number of person that can be created is 9 (i.e. maximum three rows in the window). If the entered number is greater than 9 or smaller than 1, t new number until the entered number is between 1 and 9 (inclusive). Then the program will ask the user to enter the person names and create their corresponding regions in the window with their default mood displayed (see the illustrative figure at the end of this document). The user can then select a person (the program will prompt an "unrecognized name!" message if the typed name is not recognizable). After the person is selected, the user can do the following two things: 1) type in the mood for that person; or 2) type "SS$" to delete the person. In the first case (i.e., the user's input is not "$S$"), the selected person's region is updated in the following way: he program will ask the user to enter a When the mood is happy, the region has a red When the mood is sad, the region has a green background and the mood is displayed Otherwise, the region has a white background and the mood is displayed. background and the mood is displayed O In the second case (i.e., the user's input is "SS$"), the person is deleted and the person's region (including the black boundary of the region) in the window is erased. An illustration of how the program runs and the corresponding window display are given at the end of this document. Your HW5 is to modify the Facebook example so that instead of each person has its own Facebook window, all persons are displayed in the same Facebook window. Different persons are shown at different regions of the window as illustrated by the figure at the end of this document. The size of the Facebook window is 700 550 and the size of each person's region is 200 150. The program will ask the user to enter the number of facebookpresons to be created. The maximum number of person that can be created is 9 (i.e. maximum three rows in the window). If the entered number is greater than 9 or smaller than 1, t new number until the entered number is between 1 and 9 (inclusive). Then the program will ask the user to enter the person names and create their corresponding regions in the window with their default mood displayed (see the illustrative figure at the end of this document). The user can then select a person (the program will prompt an "unrecognized name!" message if the typed name is not recognizable). After the person is selected, the user can do the following two things: 1) type in the mood for that person; or 2) type "SS$" to delete the person. In the first case (i.e., the user's input is not "$S$"), the selected person's region is updated in the following way: he program will ask the user to enter a When the mood is happy, the region has a red When the mood is sad, the region has a green background and the mood is displayed Otherwise, the region has a white background and the mood is displayed. background and the mood is displayed O In the second case (i.e., the user's input is "SS$"), the person is deleted and the person's region (including the black boundary of the region) in the window is erased. An illustration of how the program runs and the corresponding window display are given at the end of this documentStep by Step Solution
There are 3 Steps involved in it
Step: 1
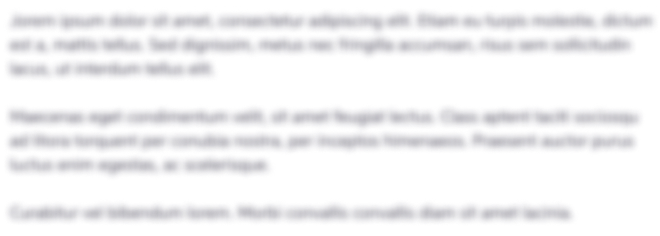
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started