Answered step by step
Verified Expert Solution
Question
1 Approved Answer
// Node.h #ifndef NODE_H #define NODE_H class Node { private: int m_entry; Node *m_next; public: Node(); Node(int entry); int getEntry() const; void setEntry(int entry); Node
// Node.h
#ifndef NODE_H #define NODE_H class Node { private: int m_entry; Node *m_next; public: Node(); Node(int entry); int getEntry() const; void setEntry(int entry); Node *getNext(); void setNext(Node *next); }; #endif
// Node.cpp
#include "Node.h" Node::Node() { m_entry = 0; m_next = nullptr; } Node::Node(int entry) { m_entry = entry; } int Node::getEntry() const { return m_entry; } void Node::setEntry(int entry) { m_entry = entry; } Node *Node::getNext() { return m_next; } void Node::setNext(Node *next) { m_next = next; }
// LinkedList.h
#ifndef LINKED_LIST_H #define LINKED_LIST_H #include "Node.h" //Gone over in class #include#include //For runtime_error using namespace std; class LinkedList { private: Node *m_front; int m_length; public: // creates an empty list LinkedList(); // creates a deep copy LinkedList(const LinkedList &original); //deletes all nodes ~LinkedList(); LinkedList &operator=(const LinkedList &original); // returns true if emtpy, false otherwise bool isEmpty() const; // Returns length int getLength() const; // adds a node containing the entry at that position // positions range from 1 to length + 1 // throws exception if out of range void insert(int position, int entry); // deletes the node at that position // positions range from 1 to length // if position out of range, throw exception void remove(int position); // deletes all nodes in the list void clear(); // Returns the entry at a given position // positions range from 1 to length // if position out of range, throw exception int getEntry(int position) const; // replaces the entry at a given position // The number of nodes is unchanged // positions range from 1 to length // position out of range, throw exception void replace(int position, int newEntry); }; #endif
// LinkedList.cpp
#include "LinkedList.h" #includeLinkedList::LinkedList() { m_front = nullptr; m_length = 0; } LinkedList::LinkedList(const LinkedList &original) { } LinkedList::~LinkedList() { clear(); } LinkedList& LinkedList::operator=(const LinkedList &original) { } bool LinkedList::isEmpty() const { return m_front == nullptr; } int LinkedList::getLength() const { return m_length; } void LinkedList::insert(int position, int entry) { } void LinkedList::remove(int position) { Node* temp = m_front; if (position > 0 && position <= m_length) { for (int i = 1; i < position; i++) { if (i == position - 1) { m_front = temp; } temp = temp -> getNext(); } m_front -> setNext(temp -> getNext()); delete(temp); m_length--; } else throw (domain_error("Position out of range!")); } void LinkedList::clear() { if (!isEmpty()) { while(m_front != nullptr) { Node* temp = m_front; m_front = m_front -> getNext(); delete(temp); m_length = 0; } } } int LinkedList::getEntry(int position) const { Node* temp = m_front; for (int i = 1; i < position; i++) { if(position > m_length) { throw (domain_error("Position out of range!")); } temp = temp -> getNext(); } return temp -> getEntry(); } void LinkedList::replace(int position, int newEntry) { }
Complete the LinkedList.cpp
Step by Step Solution
There are 3 Steps involved in it
Step: 1
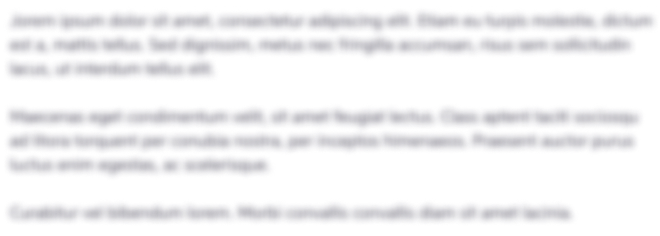
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started