Answered step by step
Verified Expert Solution
Question
1 Approved Answer
NOTE: use c programming language in Linux. Objectives: Warm up with C programming. Practice with data structures. Practice with dynamic memory allocation and pointers. Learn
NOTE: use c programming language in Linux.
Objectives: Warm up with C programming. Practice with data structures. Practice with dynamic memory allocation and pointers. Learn some system calls and practice using them. Exercise reading the man pages in Unix. Trace the systems calls that a program executes. Discipline your programs for black-box testing where the expected output will have a rigid format. 1 Project Description In this project, you will write a system program called dirtree, which takes the absolute path of a direc- tory name (such as /homealtipar/Downloads/final-src) as a command line argument and prints levelorder, and absolute path of all the files and sub-directories residing in the given directory, level by level. Your program should traverse the disk structure starting from the given directory and build a tree data structure. The root of the tree will be the given directory as a command-line argument and its children will be the files and sub-directories residing in the given directory. If a child node is a file, then it will be a leaf node; if a child node is a sub-directory, then it can have zero or more children. Since a node in the tree may have any number of children, children of a node should be represented as a linked list. You can build the tree in a recursive manner. After building the tree, it should be printed out level by level. First the root node (level one), then the nodes in level two, then the nodes in level three, and so on. In order to print the tree level by level, you will need to use a queue data structure. For each node, the following information in the specified format should be printed in a separate line: level:order:path For example, assume the given directory path is /homealtipar/Downloads/final-src and the content of this directory is: /homealtipar/Downloads/final-sre README.txt chap3 Simulator dup.c win32-pipe-parent.c chap4 thrd-posix.c Driver.java thrd-win32.c In the example above, the given directory (final-src) has two sub-directories (chap3, chap 4) and a file (README.txt). chap3 has a sub-directory (Simulator) and a file (win32-pipe-parent.c). Simulator has a file (dup.c). chap4 has three files (thrd-posix.c, Driver.java, and thrd-win32.c). For the given directory path /homealtipar/Downloads/final-src, your program will be in- voked as: ../dirtree /homealtipar/Downloads/final-src and it should print the following output to the screen: 1:1:/homealtipar/Downloads/final-src 2:1:/homealtipar/Downloads/final-src/README.txt 2:2:/homealtipar/Downloads/final-src/chap 3 2:3:/homealtipar/Downloads/final-src/chap4 3:1:/homealtipar/Downloads/final-src/chap3/Simulator 3:2:/homealtipar/Downloads/final-src/chap3/win32-pipe-parent.c 3:3:/homealtipar/Downloads/final-src/chap4/thrd-posix.c 3:4:/homealtipar/Downloads/final-src/chap4/Driver.java 3:5:/homealtipar/Downloads/final-src/chap4/thrd-win32.c 4:1:/homealtipar/Downloads/final-src/chap3/Simulator/dup.c Although you have to print the content level by level, there is no specific order to print within the individual levels. A good exercise would be trying to print each level alphabetically by the path name; however, this is not mandatory and it will not be enforced in our tests. Please check the readdir() function and the dirent structure to read the content of a directory, and the stat() function to differentiate files and directories. 2 Development It is the requirement of this assignment that you have to use the specified data structures (tree, linked list, queue), and dynamic memory allocation. You cannot assume maximum number of levels, elements, or characters, they can be any size and the memory for them should be allocated dynamically. This will allow you to practice with malloc, pointers and structures. You will develop your program in a Unix environment using the programming language. You can develop your program using a text editor (emacs, vi, gedit etc.) or an Integrated Development Environment available in Linux. gec must be used as the compiler. You will be provided a Makefile and your program should compile without any errors/warnings using this Makefile. Black-box testing will be applied and your program's output will be compared to the correct output (we will sort your output before matching). A sample directory structure is provided for you to test your program. A more complicated test (possibly more then one test) might be applied to grade your program. Submissions not following the specified rules here will be penalized. 3 Tracing the System Calls After finishing your program, you will trace the execution of your code to find out all the system calls that your program makes. To do this, you will use the strace command available in Linux. See man strace for more details on strace. In a separate README file, you will write only the names of the system calls that your program made in ascending sorted order by eliminating duplicates if any. 4 Checking the Memory Leaks You will need to make dynamic memory allocation. If you do not deallocate the memory that you allocated previously using free (), it means that your program has memory leaks. To receive full credit, your program should be memory-leak free. You can use valgrind to check the memory-leaks in your program. valgrind will output: *All heap blocks were freed - no leaks are possible" if your program is memory-leak free. Check man valgrind for more details on valgrind. 5 Submission Submission will be done through Blackboard strictly following the instructions below. Your work will be penalized 5 points out of 100 if the submission instructions are not followed. Memory leaks and compilation warnings will also be penalized with 5 points each, if any. You can check the compilation warnings using the -Wall flag of gcc. 5.1 What to Submit 1. README.txt: It should include your name, ID, and the list of system calls that your program made. Only the unique system call names (not their parameters, etc.) should be written in ascending order line by line, where each line has a single system call name. No other information should be provided. 2. dirtree.c: Source code of your program. 3. Makefile: Makefile used to compile your program. 5.2 How to Submit 1. Create a directory and name it as your UofL ID number. For example, if a student's ID is 1234567, then the name of the directory will be 1234567. 2. Put all the files to be submitted (only the ones asked in the What to Submit section above) into this directory 3. Zip the directory. As a result, you will have the file 1234567.zip. 4. Upload the file 1234567.zip to Blackboard using the "Attach File" option. You do not need to write any- thing to the "Submission" and "Comments" sections. NO LATE SUBMISSIONS WILL BE GRADED! 5. You are allowed to make multiple attempts of submission but only the latest attempt submitted before the deadline will be graded. 6 Grading Grading of your program will be done by an automated process. Your programs will also be passed through a copy-checker program which will examine the source codes if they are original or copied. We will also examine your source file(s) manually to check if you followed the specified implementation rules. 7 Changes No changes. Objectives: Warm up with C programming. Practice with data structures. Practice with dynamic memory allocation and pointers. Learn some system calls and practice using them. Exercise reading the man pages in Unix. Trace the systems calls that a program executes. Discipline your programs for black-box testing where the expected output will have a rigid format. 1 Project Description In this project, you will write a system program called dirtree, which takes the absolute path of a direc- tory name (such as /homealtipar/Downloads/final-src) as a command line argument and prints levelorder, and absolute path of all the files and sub-directories residing in the given directory, level by level. Your program should traverse the disk structure starting from the given directory and build a tree data structure. The root of the tree will be the given directory as a command-line argument and its children will be the files and sub-directories residing in the given directory. If a child node is a file, then it will be a leaf node; if a child node is a sub-directory, then it can have zero or more children. Since a node in the tree may have any number of children, children of a node should be represented as a linked list. You can build the tree in a recursive manner. After building the tree, it should be printed out level by level. First the root node (level one), then the nodes in level two, then the nodes in level three, and so on. In order to print the tree level by level, you will need to use a queue data structure. For each node, the following information in the specified format should be printed in a separate line: level:order:path For example, assume the given directory path is /homealtipar/Downloads/final-src and the content of this directory is: /homealtipar/Downloads/final-sre README.txt chap3 Simulator dup.c win32-pipe-parent.c chap4 thrd-posix.c Driver.java thrd-win32.c In the example above, the given directory (final-src) has two sub-directories (chap3, chap 4) and a file (README.txt). chap3 has a sub-directory (Simulator) and a file (win32-pipe-parent.c). Simulator has a file (dup.c). chap4 has three files (thrd-posix.c, Driver.java, and thrd-win32.c). For the given directory path /homealtipar/Downloads/final-src, your program will be in- voked as: ../dirtree /homealtipar/Downloads/final-src and it should print the following output to the screen: 1:1:/homealtipar/Downloads/final-src 2:1:/homealtipar/Downloads/final-src/README.txt 2:2:/homealtipar/Downloads/final-src/chap 3 2:3:/homealtipar/Downloads/final-src/chap4 3:1:/homealtipar/Downloads/final-src/chap3/Simulator 3:2:/homealtipar/Downloads/final-src/chap3/win32-pipe-parent.c 3:3:/homealtipar/Downloads/final-src/chap4/thrd-posix.c 3:4:/homealtipar/Downloads/final-src/chap4/Driver.java 3:5:/homealtipar/Downloads/final-src/chap4/thrd-win32.c 4:1:/homealtipar/Downloads/final-src/chap3/Simulator/dup.c Although you have to print the content level by level, there is no specific order to print within the individual levels. A good exercise would be trying to print each level alphabetically by the path name; however, this is not mandatory and it will not be enforced in our tests. Please check the readdir() function and the dirent structure to read the content of a directory, and the stat() function to differentiate files and directories. 2 Development It is the requirement of this assignment that you have to use the specified data structures (tree, linked list, queue), and dynamic memory allocation. You cannot assume maximum number of levels, elements, or characters, they can be any size and the memory for them should be allocated dynamically. This will allow you to practice with malloc, pointers and structures. You will develop your program in a Unix environment using the programming language. You can develop your program using a text editor (emacs, vi, gedit etc.) or an Integrated Development Environment available in Linux. gec must be used as the compiler. You will be provided a Makefile and your program should compile without any errors/warnings using this Makefile. Black-box testing will be applied and your program's output will be compared to the correct output (we will sort your output before matching). A sample directory structure is provided for you to test your program. A more complicated test (possibly more then one test) might be applied to grade your program. Submissions not following the specified rules here will be penalized. 3 Tracing the System Calls After finishing your program, you will trace the execution of your code to find out all the system calls that your program makes. To do this, you will use the strace command available in Linux. See man strace for more details on strace. In a separate README file, you will write only the names of the system calls that your program made in ascending sorted order by eliminating duplicates if any. 4 Checking the Memory Leaks You will need to make dynamic memory allocation. If you do not deallocate the memory that you allocated previously using free (), it means that your program has memory leaks. To receive full credit, your program should be memory-leak free. You can use valgrind to check the memory-leaks in your program. valgrind will output: *All heap blocks were freed - no leaks are possible" if your program is memory-leak free. Check man valgrind for more details on valgrind. 5 Submission Submission will be done through Blackboard strictly following the instructions below. Your work will be penalized 5 points out of 100 if the submission instructions are not followed. Memory leaks and compilation warnings will also be penalized with 5 points each, if any. You can check the compilation warnings using the -Wall flag of gcc. 5.1 What to Submit 1. README.txt: It should include your name, ID, and the list of system calls that your program made. Only the unique system call names (not their parameters, etc.) should be written in ascending order line by line, where each line has a single system call name. No other information should be provided. 2. dirtree.c: Source code of your program. 3. Makefile: Makefile used to compile your program. 5.2 How to Submit 1. Create a directory and name it as your UofL ID number. For example, if a student's ID is 1234567, then the name of the directory will be 1234567. 2. Put all the files to be submitted (only the ones asked in the What to Submit section above) into this directory 3. Zip the directory. As a result, you will have the file 1234567.zip. 4. Upload the file 1234567.zip to Blackboard using the "Attach File" option. You do not need to write any- thing to the "Submission" and "Comments" sections. NO LATE SUBMISSIONS WILL BE GRADED! 5. You are allowed to make multiple attempts of submission but only the latest attempt submitted before the deadline will be graded. 6 Grading Grading of your program will be done by an automated process. Your programs will also be passed through a copy-checker program which will examine the source codes if they are original or copied. We will also examine your source file(s) manually to check if you followed the specified implementation rules. 7 Changes No changesStep by Step Solution
There are 3 Steps involved in it
Step: 1
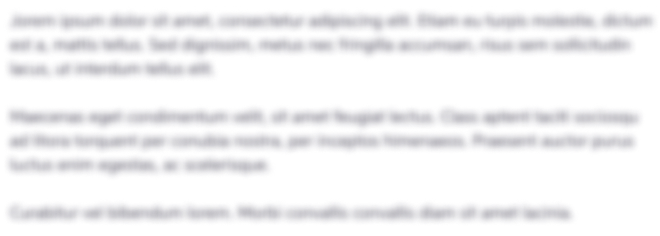
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started