Question
Objectives: 1)To learn to utilize data structures, including linked lists. 2)To sharpen your skills using decision statements, iterative control statements, arrays, pointers, structures and functions.
Objectives:
1)To learn to utilize data structures, including linked lists.
2)To sharpen your skills using decision statements, iterative control statements, arrays, pointers, structures and functions.
3)To learn to use GNU make to automate building your software.
Tasks:
1)You will create a program that manages employee records.
2) Create a header file (lastname_employeerec.h) that defines an employee data structure (sEMPLOYEE) that can be linked onto a linked list. The data structure should have the following fields:
a --First Name (firstName)
b--Last Name (lastName)
c--Employee ID (id)
d--Start Year (startYear)
e-- Starting Salary (startSalary)
f-- Current Salary (currentSalary)
g --next
3) Create a library of functions that operate on this data structure. The source code for the functions should be in lastname_employeerec.c and the function prototypes should be included in lastname_employeerec.h. The following functions should be in the library:
a-- sEMPLOYEE *create_employee_record() allocates memory for a new employee record, prompts user, through the console, to enter the data for the employee, returns a pointer to the newly created employee record
b-- sEMPLOYEE *add_employee_record(sEMPLOYEE *employeeListHead, sEMPLOYEE *employee) adds the employee record to a linked list; returns the new list head (it might have changed)
c-- sEMPLOYEE *delete_employee_record(sEMPLOYEE
*employeeListHead, unsigned int id) deletes the employee record with the specified employee ID (can linear search to find record); returns the new list head (it might have changed)
d -- void print_employee_record(sEMPLOYEE *employee) prints the data in the employee record
e-- sEMPLOYEE *sort_employee_records(sEMPLOYEE
*employeeListHead) sorts the list of employee records according to entire last name (must use bubble sort); returns the new list head (it might have changed)
f --int write_employee_records(char *filename, sEMPLOYEE
*employeeListHead) writes the list of employee records to a file; returns 0 on SUCCESS, 1 on FAILURE
sEMPLOYEE *read_employee_records(char *filename, sEMPLOYEE
*employeeListHead) reads employee records from a file and adds them to the specified linked list; the new list head (it might have changed)
4) Create an application with source code in lastname_main.c that is an employee record management system like the student record management system we developed in class. The application should give users a menu of choices:
1 add a new employee record 2 delete an employee record
3 sort employee records by last name 4 print all employee records
5 write all employee records to a file 6 read employee records from a file 7 exit
5) Create a Makefile to build two versions of your application: a normal version (employee) and a debug version (employee_dbg) that is ready for use in GDB. Your Makefile should be designed so that a source file is compiled only if it has been modified.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
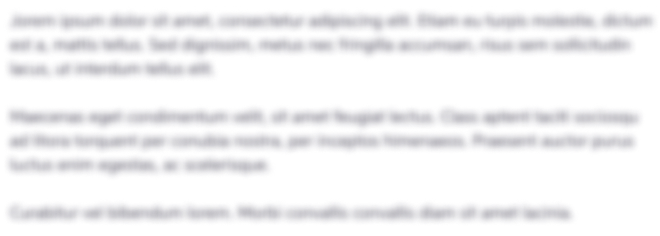
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started