Question
Objectives In this programming project, students will learn: - How to create multi-form windows form application - How to define visual inheritance - How to
Objectives In this programming project, students will learn: - How to create multi-form windows form application - How to define visual inheritance - How to define classes and inheritance - How to define and use object array - How to perform object-oriented programming by encapsulating data and methods in objects Goals In this lab assignment, students will demonstrate the abilities to: - Create multi-form windows form application - Define visual inheritance - Define classes and inheritance - Define and use object array - Perform object-oriented programming by encapsulating data and methods in objects Grading rubric Category High Proficiency Medium Proficiency Low Proficiency Define GUIs for multiple windows forms [20 pts] Successfully define all window forms with the appropriate controls. [20pts] Most window forms are defined correctly, some forms or controls are not defined appropriately. [10-19 pts] Most window forms are missing, or most controls are not defined appropriately. [0-9 pts] Define classes and inheritance [30 pts] Successfully define all classes, inheritance relationships are defined correctly. [30ts] Most classed are defined correctly, applied inheritance, however, some codes are not correct. [10-29 pts] Most classes are not defined correctly, or the inheritance is not defined correctly. [0-9 pts] Define event handler methods for "Login" form (5 pts) Successfully define the login functionality. [5 pts] Minor issues are found for login functionality [3-5 pts] Can not implement login functionality. [0-2pts] Define event handler methods for "Search" form (10 pts) Successfully implement "search" functionality and define the button-click methods. [10 pts] Minor issues are found for "search" functionality, or the button-click methods. [5-9 pts] Major issues are found for "search" functionality, or the button-click methods. [0-4 pts] Define event handler methods for "View" form [10 pts] Successfully implement "view" functionality and define the button-click methods. [10 pts] Minor issues are found for "view" functionality, or the button-click methods. [5-9 pts] Major issues are found for "view" functionality, or the button-click methods. [0-4 pts] Define event handler methods for "Shopping Cart" form [10 pts] Successfully implement "shopping cart" functionality and define the button-click methods. [10 pts] Minor issues are found for "shopping cart" functionality, or the button-click methods. [5-9 pts] Major issues are found for "shopping cart" functionality, or the button-click methods. [0-4 pts] Define event handler methods for "Check Out" form [10 pts] Successfully implement "check out" functionality and define the button-click methods. [10 pts] Minor issues are found for "check out" functionality, or the button-click methods. [5-9 pts] Major issues are found for "check out" functionality, or the button-click methods. [0-4 pts] Define event handler methods for "Confirmation" form [5 pts] Successfully implement "confirmation" functionality and define the button-click methods. [5 pts] Minor issues are found for "confirmation" functionality, or the button-click methods. [3-5 pts] Major issues are found for "confirmation" functionality, or the button-click methods. [0-2 pts] In this project, you will design and develop a Bookstore Multi-form Windows Form Application and perform Object Oriented Programming. This project covers Chapter 9, 10 and 14. Application Descriptions When executes your project, a Login form should be displayed first, the password TextBox's PasswordChar property should be set as "*": In order to make testing easier, please use "csc239" as user name, and "password" as password value. After user logs in successfully, user could search for the publication by titles from the following Search form. Do not need to display many publications; 4-6 items should be fine. For example, only let user search for publication from 2 books and 2 magazines: User then could view the information for the selected publication on the View form: If the user wants to buy the viewed publication, he/she could add it in the shopping cart, then view the shopping cart or continue shopping. If the user does not plan to buy the viewed publication, he/she could go back to select and view other publications. When the user chooses to view shopping cart, the ShoppingCart form should display the publication title, item's price and let user select quantity to buy: Then user may choose "Check out" or "Continue shopping". The Checkout form should display the following information and let user input billing and shipping address, provide gift information and select deliver date. After checking out, user may choose to display Confirmation form or go back to modify the shopping cart information (For example - Quantity of publication). This is a sample confirmation form which displays amount due and shipping information. User may leave this bookstore from this form by clicking "Thanks for shopping" button. Programming Technical Specifications Object-oriented programming need to be performed. All data must be encapsulated in objects (declared as instance variables in class definition). Inheritance and polymorphism should be executed if appropriate. Database is not needed; you may save the publication objects in an array. So do not add too many publications in your book store, 4 to 6 items should be fine. Try to add controls in the shopping cart form during execution time if necessary (writing code to add controls dynamically, see examples below). Exceptions handling is encouraged but not required. Use debugger or testing technologies to test your application. The software testing documentations will be posted on Black Board. This is an open project, there is no standard solution. Good luck! Development Steps o Design and build GUI forms o Design and define classes and modules. o Complete GUI_behind code files by defining event handler methods and other methods. ------------------------------------------------------------------------------------------------------ In order to help you to manage your programming project, we split the project into two parts. Please complete tasks for each part and submit for credits by the due dates specified below. Part 1 1. Design and Build GUI Forms It must be a multi-form Windows Form Application. Build as many GUI forms as needed. You may design any GUI as you like. The GUI should be convenient and efficient to perform the required tasks specified above. In order to achieve visual consistency across your apps, you may define a base form which contains the common controls and let all other forms inherit from the base form visually. Should let user perform the following tasks through GUI forms o Log in the application o Search for publication o View the information of the selected publication (Only select and view ONE publication at one time) o Display the selected publications in shopping cart form and let user choose quantities of ordered items. o Allow user input billing or shipping information in the checkout form. o Display confirmation on the confirmation form. Set up the properties for each control after you build the forms. 2. Design and Define Classes Define as many classes as needed by adding class files in your project. Each class should be defined in its own file. Encapsulate all data in classes Define inheritance between the related classes For example: Derived class Derived class o This class represents an order item object. 3. Define Modules If one or more variables or objects will be shared by more than one Classes or Forms, declare them as public variables or objects in a Module. This Module is only a group of declarations; it will not be executed, so you do not need to initialize these objects. Right-click the project name Add Module type name Declare public variables For example: Module BookStroe Public myBookStore As BookStoreDatabase Public myCart As ShoppingCart Public myCustomer As Customer End Module 4. Compress the whole project folder into a zip file and submit for grading. After you complete all tasks of part 1, compress the whole project folder into a zip file and submit for grading. The due date for part 1 is: 11:59pm on 02/15/2017. ------------------------------------------------------------------------------------------------------ Part 2 1. Complete GUI_behind code files by defining event handler methods and other methods 2. Test and debug the functionalities of each form. 3. Compress the whole project folder into a zip file and submit for grading. 4. The due date for part 1 is: 11:59pm on 03/22/2017. References should be set to a new object before they could be used. You need to initialize myBookStore, myCart and myCustomer objects after user log in successfully from the Login. After this, myBookStore,myCart and myCustomer can be used in any Form's code. Private Sub btnLogin_Click(......) Handles btnLogin.Click ............... myBookStore = New BookStoreDatabase() myCart = New ShoppingCart() myCustomer = New Customer() ............... End Sub When you open a new Form from the current Form, you may create an object of the new Form, let this object show and hide the current Form. The following code demonstrates how to open Search Form from Login Form when user presses "Login" button: Public Class frmLogin Private Sub btnLogin_Click(.........) Handles btnLogin.Click Dim f As New frmSearch() f.Show() Me.Hide() End Sub End Class When you open a new Form and do something in the new Form, you may choose one of the following ways. For example, if user wants to view publication's information when clicking "View" button in "Search" Form, a new "View" Form will be shown and displays publication's info: Public Class frmSearch Private Sub btnView_Click(.........) Handles btnView.Click ......... Dim viewForm As New frmView() 'let viewForm display publication's info here viewForm.Label.Text = ......... Me.Hide() viewForm.Show() ......... End Sub End Class Or Public Class frmSearch Private Sub btnView_Click(.........) Handles btnView.Click ......... Dim viewForm As New frmView() ' do not display publication's info here ' display publication's info in the form_load ' method of View Form, see code bellow Me.Hide() viewForm.Show() ......... End Sub End Class Public Class frmView Private Sub frmView_Load(......) Handles Me.Load ' display publication's info here Label.Text = ..... End Sub End Class In shopping cart form, you may use one of the following two ways to add controls and display items in the cart: 1) declare array of controls, the size of array should be determined by number of items in the shopping cart, then you could use a loop to add the controls in the shopping cart Form dynamically: (myCart.itemNumber refers to number of ordered items in myCart) Public Class frmCart Dim labels(myCart.itemNumber - 1) As Label Dim textBoxes(myCart.itemNumber - 1) As TextBox Dim numUpDowns(myCart.itemNumber - 1) As NumericUpDown .......... Private Sub frmCart_Load(......) Handles MyBase.Load Dim xLabel As Integer = 50 Dim xTextBox As Integer = 240 Dim xNumUpDown As Integer = 370 Dim y As Integer = 138 Dim width As Integer = 55 Dim height As Integer = 20 If myCart.itemNumber > 0 Then For i As Integer = 0 To myCart.itemNumber - 1 labels(i) = New Label() labels(i).Location = New Point(xLabel, y) labels(i).Size = New Size(width, height) labels(i).Text = myCart.cart(i).name Me.Controls.Add(labels(i)) ' Add other controls here y += 40 Next Else ............ End If End Sub ............ End Class 2) Because there are only 4 publications in the bookstore, so you may add 4 sets of controls on the Form in the design time and make them invisible. In the frmCart_Load()event handler method, check number of items in the cart (myCart.itemNumber),modify the corresponding properties of the controls and make them visible.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
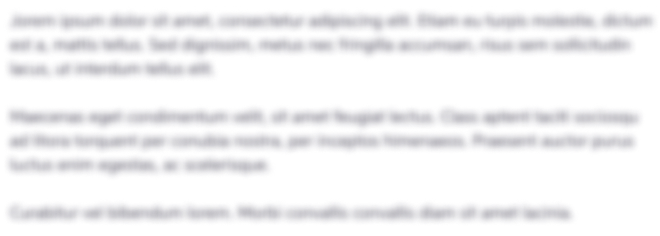
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started