Question
Object.py #!/usr/bin/env python3 from objects import Card, Hand, Deck def main(): print(Cards - Tester) print() #test sorting of the cards testcardsList = [Card(Ace,Spades), Card(Queen,Hearts), Card(10,Clubs),
Object.py
#!/usr/bin/env python3 from objects import Card, Hand, Deck
def main(): print("Cards - Tester") print()
#test sorting of the cards testcardsList = [Card("Ace","Spades"), Card("Queen","Hearts"), Card("10","Clubs"), Card("3","Diamonds"), Card("Jack","Hearts"), Card("7","Spades")] testcardsList.sort() print("TEST CARDS LIST AFTER SORTING.") for c in testcardsList: print(c) print()
# test deck print("DECK") deck = Deck() print("Deck created.") deck.shuffle() print("Deck shuffled.") print("Deck count:", len(deck)) print()
# test hand hand = Hand() for i in range(10): hand.addCard(deck.dealCard())
print("SORTED HAND") for c in sorted(hand): print(c)
print() print("Hand points:", hand.points) print("Hand count:", len(hand)) print("Deck count:", len(deck))
if __name__ == "__main__": main()
Blackjack.py:
#!/usr/bin/env python3
from objects import Card, Deck, Hand
class Blackjack: def __init__(self, startingBalance): self.money = startingBalance self.deck = Deck() self.playerHand = Hand() self.dealerHand = Hand() self.bet = 0
def displayCards(self,hand, title): ''' Print the title and display the cards in the given hand in a sorted order''' print(title.upper()) for c in sorted(hand): print(c) print()
def handle_winner(self, winner, message): ''' print the player's hand's points print the message Update self.money according to the winner ''' pass #To be implemented in Phase 2 def getBet(self): ''' Method to update self.bet by prompting the user for the bet amount, making sure bet is less than self.money. ''' pass #To be implemented in Phase 2 def setupRound(self): ''' Setup the round by doing these steps: Call getBet to initialize self.bet, initialize self.deck to a new Deck object and shuffle it initialize self.dealerHand and self.playerHand to new Hand objects deal two cards to the playerHand, and one card to the dealerHand finally, print dealerHand and playerHand using displayCards method ''' pass # To be implemented in Phase 2
def play_playerHand(self): ''' Method to implement player playing his hand by 1. Prompting the user to indicate Hit (h) or Stand (s) 2. If user picks stand, end the player play by returning 3. If user picks hit, deal a card to the playerHand. check if with the latest addition, the hand busts (has > 21 points), if so return otherwise, prompt the player again whether to hit or stand. 4. Print playerHand points ''' pass # To be implemented in Phase 2 def play_dealerHand(self): ''' Method to play the dealer's hand. Continue to deal cards till the points of the dealerHand are less than 17. Print the dealer's hand before returning''' pass # To be implemented in Phase 3 def playOneRound(self): ''' Method implements playing one round of the game 1. Checks if playerHand is a Blackjack, if so handles that case 2. Lets player play his hand if it busts, declares player loser 3. Else lets dealer play his hand. 4. If dealer busts, declares the player to be the winner 5. Otherwise declares the winner based on who has higher points: if Player > dealer, player is the winner else if player
def main(): print("BLACKJACK!") print("Blackjack payout is 3:2")
# initialize starting money money = 100 print("Starting Balance:", money)
blackjack = Blackjack(money) # start loop again = 'y' while again.lower() == 'y':
print("Setting up a round...") blackjack.setupRound()
print("Playing Player Hand...") blackjack.play_playerHand()
print() again = input("Play again? (y): ").lower() print() if again != "y": break
print("Bye!")
# if started as the main module, call the main function if __name__ == "__main__": main()
phase 2 instructions:
this project is in python code, thanks!
#!/usr/bin/env python3 from objects import Card, Hand, Deck def main(): print("Cards print() Tester") #test sorting of the cards testcardsList = [Card("Ace", "Spades"), Card("Queen", "Hearts"), Card("10", "Clubs"), Card("3", "Diamonds"), Card("Jack", "Hearts"), Card("7", "Spades")] testcardsList.sort() print("TEST CARDS LIST AFTER SORTING.") for cin testcardsList: print(c) print() # test deck print("DECK") deck = Deck print("Deck created.") deck.shuffle print("Deck shuffled.") print("Deck count:", len(deck)) print) # test hand hand = Hand for i in range (10): hand.addCard(deck.dealCard) print("SORTED HAND") for c in sorted (hand): print(c) print) print("Hand points:", hand.points) print("Hand count:", len(hand)) print("Deck count:", Len(deck)) if name main main #!/usr/bin/env python3 from objects import Card, Deck, Hand class Blackjack: def init__(self, startingBa lance): self.money = startingBalance self.deck = Deck() self.playerHand = Hand self.dealerHand = Hand self.bet = 0 order! def displayCards(self, hand, title): Print the title and display the cards in the given hand in a sorted print(title.upper) for c in sorted (hand): print(c) print() def handle_winner (self, winner, message): print the player's hand s points print the message Update self.money according to the winner pass =To be implemented in Phase 2 def getBet (self): Method to update self.bet by prompting the user for the bet amount, making sure bet is less than self.money. pass =To be implemented in Phase 2 def setupRound(self): Setup the round by doing these steps: Call get Bet to initialize self.bet, initialize self.deck to a new Deck object and shuffle it initialize self.dealerHand and self.player Hand to new Hand objects deal two cards to the player Hand, and one card to the dealer Hand finally, print dealer Hand and player Hand using displayCards method pass = To be implemented in Phase 2 def play_player Hand (self): Method to implement player playing his hand by 1. Prompting the user to indicate Hit (h) or Stand (s) 2. If user picks stand, end the player play by returning 3. If user picks hit, deal a card to the playerHand. check if with the latest addition, the hand busts (has > 21 points), if so return otherwise, prompt the player again whether to hit or stand. 4. Print player Hand points pass # To be implemented in Phase 2 def play_dealerHand(self): Method to play the dealer's hand. Continue to deal cards till the points of the dealer Hand are Less than 17. Print the dealer's hand before returning' pass # To be implemented in Phase 3 def playOneRound(self): In Method implements playing one round of the game 1. Checks if player Hand is a Blackjack, if so handles that case 2. Lets player play his hand if it busts, declares player loser 3. Else lets dealer play his hand. 4. If dealer busts, declares the player to be the winner 5. Otherwise declares the winner based on who has higher points: if Player > dealer, player is the winner else if player Hearts > Diamonds > Clubs. (Luckily, these are alphabetically ordered as well!) The ranks follow this order: Ace > King > Queen > Jack > 10 > 9 >8 >7>6 >5 > 4>3 > 2. There are many ways to implement this. b. You will make the card class printable. You should replace the display Card method with one of the special methods. (Which one?) 2. Deck and Hand Classes: a. Make the Deck and Hand classes iterable, by adding a Generator function as we discussed in the class. One possible way is to have a dictionary, say RANKORDER defined whose keys are the possible ranks ("Ace", "King", ... "4", "3", "2"), and values are some integers that match the desired ordering of the ranks. E.g. RANKORDER = { "2":2,"3": 3, .... "10":10,"Jack":11,"Queen":12, "King":13,"Ace": 14} Clearly RANKORDER["2"] >> Ln: 162 Col: 0 The main function has a list called testcardsList, comprising of test cards. When the sort method is called on this list and the result printed, it should show them in sorted order as seen in the sample output. Also the cards displayed in the sorted hand towards the end of the main function should appear is correct sort order. This is the second phase of the course project which we have already started. In this phase, we will improve our program from phase 1. You will do this in two stages: first, you will update the classes in objects.py and second, you will add implementation to some of the methods of the partially implemented Blackjack class in the file blackjack.py. The rules of the game we are implementing are described at the end in the Appendix. Please read those to get a better idea of the game. Don't be discouraged by the length of this document or the rules of the game listed in the Appendix. Just follow the instructions and you will have no problem getting the game to work. Step 1: Update classes in objects.py: You will update the classes in the objects.py in the following way: 1. Card Class: a. You will make the Card class comparable so that when a hand is displayed, the cards in the hand are shown in a sorted order (what set of special methods will you need to add?). Remember that when comparing two cards, you want to compare their suit attributes first and then the rank attributes. Note that the suits follow this order: Spades > Hearts > Diamonds > Clubs. (Luckily, these are alphabetically ordered as well!) The ranks follow this order: Ace > King > Queen > Jack > 10 > 9 >8 >7>6 >5 > 4>3 > 2. There are many ways to implement this. b. You will make the card class printable. You should replace the display Card method with one of the special methods. (Which one?) 2. Deck and Hand Classes: a. Make the Deck and Hand classes iterable, by adding a Generator function as we discussed in the class. One possible way is to have a dictionary, say RANKORDER defined whose keys are the possible ranks ("Ace", "King", ... "4", "3", "2"), and values are some integers that match the desired ordering of the ranks. E.g. RANKORDER = { "2":2,"3": 3, .... "10":10,"Jack":11,"Queen":12, "King":13,"Ace": 14} Clearly RANKORDER["2"] 21 points), if so return otherwise, prompt the player again whether to hit or stand. 4. Print player Hand points pass # To be implemented in Phase 2 def play_dealerHand(self): Method to play the dealer's hand. Continue to deal cards till the points of the dealer Hand are Less than 17. Print the dealer's hand before returning' pass # To be implemented in Phase 3 def playOneRound(self): In Method implements playing one round of the game 1. Checks if player Hand is a Blackjack, if so handles that case 2. Lets player play his hand if it busts, declares player loser 3. Else lets dealer play his hand. 4. If dealer busts, declares the player to be the winner 5. Otherwise declares the winner based on who has higher points: if Player > dealer, player is the winner else if player Hearts > Diamonds > Clubs. (Luckily, these are alphabetically ordered as well!) The ranks follow this order: Ace > King > Queen > Jack > 10 > 9 >8 >7>6 >5 > 4>3 > 2. There are many ways to implement this. b. You will make the card class printable. You should replace the display Card method with one of the special methods. (Which one?) 2. Deck and Hand Classes: a. Make the Deck and Hand classes iterable, by adding a Generator function as we discussed in the class. One possible way is to have a dictionary, say RANKORDER defined whose keys are the possible ranks ("Ace", "King", ... "4", "3", "2"), and values are some integers that match the desired ordering of the ranks. E.g. RANKORDER = { "2":2,"3": 3, .... "10":10,"Jack":11,"Queen":12, "King":13,"Ace": 14} Clearly RANKORDER["2"] >> Ln: 162 Col: 0 The main function has a list called testcardsList, comprising of test cards. When the sort method is called on this list and the result printed, it should show them in sorted order as seen in the sample output. Also the cards displayed in the sorted hand towards the end of the main function should appear is correct sort order. This is the second phase of the course project which we have already started. In this phase, we will improve our program from phase 1. You will do this in two stages: first, you will update the classes in objects.py and second, you will add implementation to some of the methods of the partially implemented Blackjack class in the file blackjack.py. The rules of the game we are implementing are described at the end in the Appendix. Please read those to get a better idea of the game. Don't be discouraged by the length of this document or the rules of the game listed in the Appendix. Just follow the instructions and you will have no problem getting the game to work. Step 1: Update classes in objects.py: You will update the classes in the objects.py in the following way: 1. Card Class: a. You will make the Card class comparable so that when a hand is displayed, the cards in the hand are shown in a sorted order (what set of special methods will you need to add?). Remember that when comparing two cards, you want to compare their suit attributes first and then the rank attributes. Note that the suits follow this order: Spades > Hearts > Diamonds > Clubs. (Luckily, these are alphabetically ordered as well!) The ranks follow this order: Ace > King > Queen > Jack > 10 > 9 >8 >7>6 >5 > 4>3 > 2. There are many ways to implement this. b. You will make the card class printable. You should replace the display Card method with one of the special methods. (Which one?) 2. Deck and Hand Classes: a. Make the Deck and Hand classes iterable, by adding a Generator function as we discussed in the class. One possible way is to have a dictionary, say RANKORDER defined whose keys are the possible ranks ("Ace", "King", ... "4", "3", "2"), and values are some integers that match the desired ordering of the ranks. E.g. RANKORDER = { "2":2,"3": 3, .... "10":10,"Jack":11,"Queen":12, "King":13,"Ace": 14} Clearly RANKORDER["2"]Step by Step Solution
There are 3 Steps involved in it
Step: 1
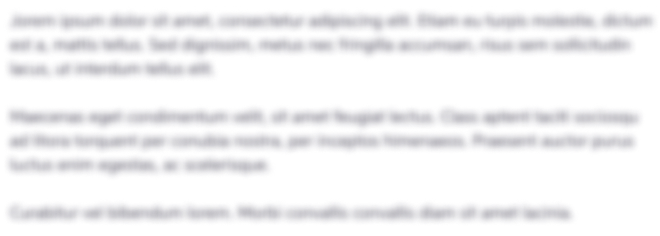
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started