Question
On this game, write a spec sheet stating what is required and what to do to accomplish that requirement. Question 1. import random
On this game, write a spec sheet stating what is required and what to do to accomplish that requirement.
Question 1.
import random
from os import system, name
#
def Roll(times,sides):
ans = 0
for i in range(int(times)):
num = random.randint(1,int(sides))
ans += num
return ans
#
def TargetRoller(times,sides,target):
ans = 0
for i in range(int(times)):
num = random.randint(1,int(sides))
if num >= int(target):
ans += 1
return ans
#
def Clear():
if name == 'nt':
system('cls')
else:
system('clear')
Question 2.
import random
import my_utilities
def GenerateName():
syl1 = "Mil Kat Jon Kit Bob Nor Car Tat Dem Min Lav Cat Chri Tho Jod Mar Fun Rif Crum Arm Apr Jag Kub".split()
syl2 = "der mar tre smel lon wal tea dog dan nic jack swea qui lon det cat jud faus mill brie sin cor reg".split()
return syl1[random.randint(0,len(syl1)-1)] + syl2[random.randint(0,len(syl2)-1)]
def GenerateHistory():
nouns = "virus dinner orange instance method scene worker development thing village newspaper sample trainer session winner construction".split()
verbs = "succeed prefer suppress qualify feel reserve resolve fine recommend weaken stare pour borrow preserve result cause negotiate line tie agree".split()
adjectives = "sufficient difficult anxious sexual traditional sorry exciting actual wonderful able several existing used distinct expensive latter".split()
places = "Miramichi Goondiwindi Miami Nephi Ojai Philippi Hanalei Biloxi Cincinnati Manti Bemidji Lodi Oraibi Lehi Walpi Corpus Christi Ypsilanti".split()
trivia = ["A [noun] is often one [noun] above you.",
"You have a lot of [adjective] [noun]s.",
"You'd rather be a [noun] than a [noun].",
"You did not [verb] the [noun], for it was not the right thing to do.",
"You love [verb]ing [adjective] [noun]."]
origins = ["You are from [place].",
"You were born in [place].",
"You recently moved away from [place].",
"Your friend has been telling you about [place].",
"You went to school in [place].",
"Some people call you [place]."]
pasts = ["You once [verb]ed a [noun]",
"You hadn't [verb]ed for a few months.",
"The [noun]s [verb]ed you last summer.",
"Your [noun]s didn't [verb].",
"You recently [verb]ed."]
history = []
history.append(origins[random.randint(0,len(origins)-1)])
history.append(trivia[random.randint(0,len(trivia)-1)])
history.append(pasts[random.randint(0,len(pasts)-1)])
newhistory = []
for sentence in history:
sentence = sentence.replace("[noun]", nouns[random.randint(0,len(nouns)-1)],1)
sentence = sentence.replace("[noun]", nouns[random.randint(0,len(nouns)-1)],1)
sentence = sentence.replace("[verb]", verbs[random.randint(0,len(verbs)-1)])
sentence = sentence.replace("[adjective]", adjectives[random.randint(0,len(adjectives)-1)])
sentence = sentence.replace("[place]", places[random.randint(0,len(adjectives)-1)])
newhistory.append(sentence)
return newhistory
def GenPlayer(level):
player = {}
#
player["name"] = GenerateName()
player["history"] = GenerateHistory()
player['treasures'] = []
#
player["level"] = level
player["attack"] = my_utilities.Roll(3,6) + my_utilities.Roll(level,4)
player["defense"] = my_utilities.Roll(3,6) + my_utilities.Roll(level,4)
player["health"] = my_utilities.Roll(5,6) + my_utilities.Roll(level,4)
#
player["row"] = 0
player["col"] = 0
return player
Question 3
import random
import my_utilities
def GenTreasure(board):
#
treasure = {}
treasure['row'] = random.randint(0,len(board)-1)
treasure['col'] = random.randint(0,len(board[0])-1)
#
x = random.randint(1,9)
if x == 1:
treasure['name'] = "Rare Set of Enchanted Toiletries"
treasure['points'] = random.randint(1,4) * 10
elif x == 2:
treasure['name'] = "Dwarven Shotglass"
treasure['points'] = random.randint(3,5) * 10
elif x == 3:
treasure['name'] = "Beer of Destiny"
treasure['points'] = random.randint(6,8) * 10
elif x == 4:
treasure['name'] = "The Faster Master Blaster of Disaster"
treasure['points'] = random.randint(7,10) * 10
elif x == 5:
treasure['name'] = "Flux Capacitor"
treasure['points'] = random.randint(6,9) * 10
elif x == 6:
treasure['name'] = "Cage containing a Bloodthirsty Furby"
treasure['points'] = random.randint(1,2) * 10
elif x == 7:
treasure['name'] = "Full body statue of Nicolas Cage"
treasure['points'] = random.randint(8,10) * 10
elif x == 8:
treasure['name'] = "Potion of Sarcasm. THE BEST POTION EVER!"
treasure['points'] = random.randint(1,5) * 5
elif x == 9:
treasure['name'] = "The Chill-axe. Everyone better simmer down RIGHT NOW."
treasure['points'] = random.randint(6,9) * 10
return treasure
def GenMinorTreasure(player):
#
verb = ['meet','find','encounter','stumble upon']
adjective = ['secluded ','friendly ','wandering ','reluctant ', 'timid ','battle-tested ']
noun = ['monk','adventurer','alchemist','priest','caravan leader','merchant','barkeep','bard']
enders = [" who helps you hone your skills.",
" who imparts some of his knowledge to you.",
" who sells you an experimental potion.",
" who relates a tale of their adventures."]
encounterString = "You "+verb[random.randint(0,len(verb)-1)]+" a "+adjective[random.randint(0,len(adjective)-1)]+noun[random.randint(0,len(noun)-1)]
encounterString += enders[random.randint(0,len(enders)-1)]
print (encounterString)
#
increase = random.randint(1,4)
stat = random.randint(1,4)
if stat == 1:
stat = "attack"
elif stat == 2:
stat = "defense"
else:
increase *= 2
stat = "health"
print ("Gain "+str(increase)+" "+stat)
player[stat] += increase
return player
def CheckTreasure(treasure, player):
#
if [player['row'],player['col']] == [treasure['row'],treasure['col']]:
return True
Step by Step Solution
There are 3 Steps involved in it
Step: 1
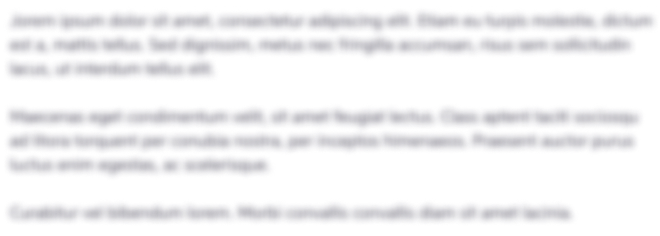
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started