Question
One ZIP file needs to submit to D2L with the following naming convention CPRG251_A1_Firstname_Lastname.zip using your first and last name. If working in a group
- One ZIP file needs to submit to D2L with the following naming convention CPRG251_A1_Firstname_Lastname.zip using your first and last name.
- If working in a group of two (2), only one team member needs to submit to D2L (both can if you so wish). Both members will receive the same feedback. The file should have the following namingconvention:CPRG251_A1_Lastname of member 1_Lastname of member 2.zip
- The ZIP file must contain the following: 1. The following directory structure:
- bin/ - Compiled Java files.
- src/ - Java source code files:
- sait/mms/application/
- sait/mms/managers/
- sait/mms/problemdomain/
- doc/ - Generated Javadoc files.
- Ensure the private option is checked and everything is included in the generated documentation.
- lib/ - Any third-party libraries. This folder can be empty.
- res/ - Any resource or data files.
- test/ - Unit test cases. This folder can be empty.
- A text file named Readme.txt in the root folder of the ZIP archive and contain:
- A project title.
- What the program does.
- The date.
- The author
- How to run the program.
- A runnable JAR file in the root folder of the ZIP archive.
- Use the naming convention: FirstInitialLastname1.jar (i.e.: JBlow1.jar).
- It is to be built using only Eclipse IDE and JDK 1.8x.
- Text files containing the output of each completed runner's main method.
- Use the name of the runner for the text file (i.e.: XYZRunner.txt)
Assignment Instructions
Import the provided Java code into your Eclipse. Go through the provided runner classes (in the order below) to implement the related class.
- MovieRunner
- MovieManagementSystemRunner
- MovieManagementSystemMenuRunner
Start at the top of the runner class then go down implementing each called method correctly. Once you have completed implementing the class, copy and paste the output from the runner's main method into a text file called XYZRunner.txt (replacing XYZRunner with the corresponding runner).
Use the provided JavaDocs, and output formatting to implement the classes.
Assignment Guidelines
- You will use only Eclipse IDE.
- The due date for this assignment is posted in D2L in the assignment submission area and in the provided calendar located in the Course Information area.Any assignment submitted after the due date will receive a mark of zero, but feedback maybe given.
- Submissions must be student's original work.Refer to the Academic Misconduct (AC.3.4) policies and procedures.
Problem
Write a movie management system using object-oriented design principles. The program will read from the supplied data file into a single array list. The data file (movies.txt) contains information about the movies. Each movie record has the following attributes:
- Duration (in minutes)
- Title
- Year of release
Each record in the movies.txt file is formatted as follows:
- Duration,Title,Year
- e.g.:91,Gravity,2013
Specifically, you must create an interactive menu driven application that gives the user the following options:
- Add a new movie.
- The user will be prompted to enter the duration in minutes, title of the new movie, and the year the movie was released. Before the movie is added, the inputs provided by the user should be validated:
- The duration and year of the movie should not be zero and the title of the movie should not be empty.
- The user will be prompted to enter the duration in minutes, title of the new movie, and the year the movie was released. Before the movie is added, the inputs provided by the user should be validated:
- Generate list of movies released in a year.
- The user will input a year and the program will display a list of all the movies released in that year along with the duration (in minutes) of all the movies.
- The list of movies does not have to be sorted.
- Generate list of random movies.
- The user will input the number of movies and the program will display a list containing the number of random movies along with the duration (in minutes) of all the movies.
- There is no minimum or maximum duration for the movies in the list.
- You can use Collections.shuffle in the java.utils package to randomize the movie list.
- Exit the program.
- Save the list of movies back into the data file "movies.txt" using the above format (Duration,Title,Year).
- The process terminates cleanly.
Notes:
To follow the object-oriented principles, your project should contain ONLY the following classes and methods in their respective package.
Package | Class | Methods |
sait.mms.application | AppDriver | main |
sait.mms.managers | MovieManagementSystem | Default constructor, displayMenu, addMovie, generateMoviesInYear, generateRandomMovieList, loadMovies, writeMoviesToFile |
sait.mms.problemdomain | Movie | Non-default constructor, Accessor methods, toString |
You cannot use parallel and/or nested arrays in this assignment (you can use ArrayList).
I'm not used to use the Eclipse. so I don't know how to start and where I need to start to write down my code. based on this documents, I would appreciate it if you could tell me how to start the code from this document.
import sait.mms.problemdomain.*;
/** * Runner class for implementing the Movie class. * DO NOT modify any of the System.out.* lines of code in this file. * @author Nick Hamnett * @version Dec 12, 2021 */ public class MovieRunner {
/** * @param args */ public static void main(String[] args) { System.out.println("Create Movie object:"); /* * Creates a Movie object using a user-defined constructor. */ int duration = 125; String title = "Tron: Legacy"; int year = 2010; //Movie movie = new Movie(duration, title, year); // System.out.println("==========================="); System.out.println(); System.out.println("Call movie.getDuration():"); // Expected output: 125 //System.out.println(movie.getDuration()); // System.out.println("==========================="); System.out.println(); System.out.println("Call movie.getTitle():"); // Expected output: Tron: Legacy //System.out.println(movie.getTitle()); // System.out.println("==========================="); System.out.println(); System.out.println("Call movie.getYear():"); // Expected output: 2010 //System.out.println(movie.getYear()); // System.out.println("==========================="); System.out.println(); System.out.println("Call movie.formatForFile():"); // Expected output: 125,Tron: Legacy,2010 //System.out.println(movie.formatForFile()); // System.out.println("==========================="); System.out.println(); System.out.println("Call movie.toString():"); // Expected output: 125 Tron: Legacy 2010 //System.out.println(movie); // System.out.println("==========================="); System.out.println(); }
}
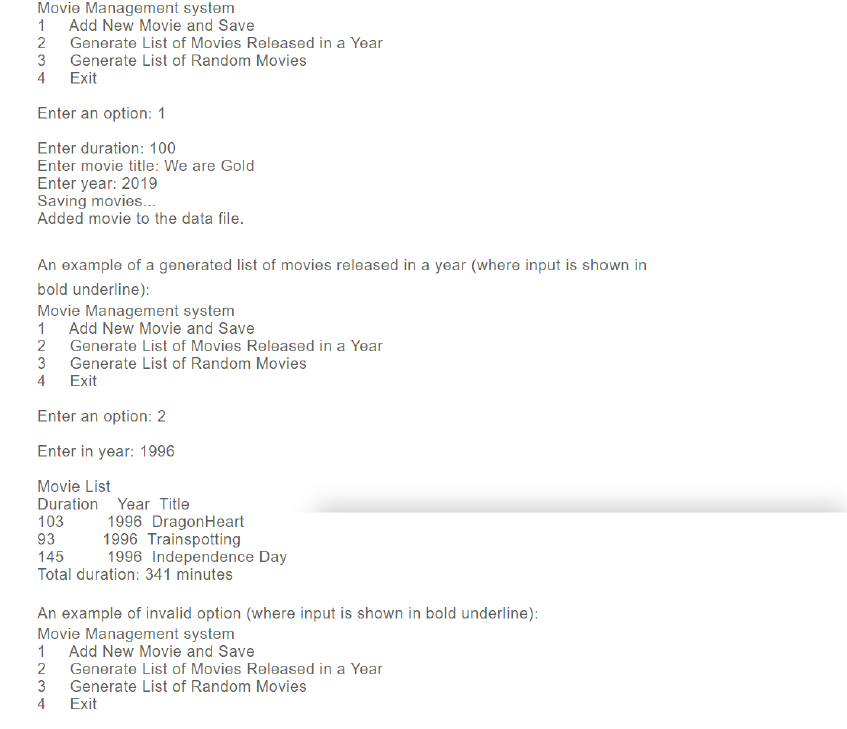
Movie Management system 1 2 Add New Movie and Save Generate List of Movies Released in a Year Generate List of Random Movies 3 4 Exit Enter an option: 1 Enter duration: 100 Enter movie title: We are Gold Enter year: 2019 Saving movies... Added movie to the data file. An example of a generated list of movies released in a year (where input is shown in bold underline): Movie Management system Add New Movie and Save Generate List of Movies Released in a Year 1 2 3 4 Exit Generate List of Random Movies Enter an option: 2 Enter in year: 1996 Movie List Year Title Duration 103 1996 DragonHeart 93 145 1996 Trainspotting 1996 Independence Day Total duration: 341 minutes An example of invalid option (where input is shown in bold underline): Movie Management system 1 2 Add New Movie and Save Generate List of Movies Released in a Year Generate List of Random Movies 3 4 Exit
Step by Step Solution
3.42 Rating (155 Votes )
There are 3 Steps involved in it
Step: 1
moviestxt 91Gravity2013 132Thin Red Line2002 130Terminator1994 120Titanic1996 120Saving Pirate Ryan1...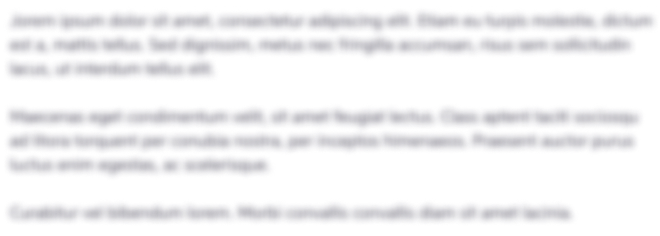
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started