Question
[Operating system] In the following code it uses pipes and file descriptors. It opens and close the pipes but I don't understand why and when
[Operating system]
In the following code it uses pipes and file descriptors. It opens and close the pipes but I don't understand why and when it needs to do such a thing. I know this program will read input and print, but not very clear about other things.
#include < stdio.h >
#include < stdlib.h >
#include < unistd.h >
#include < string.h >
int main()
{
char mapl[] = "qwertyuiopasdfghjklzxcvbnm"; /* for encoding letter */
char mapd[] = "1357924680"; /* for encoding digit */
int fd[2]; /* for the pipe */
char buf[80], buf2[80];
int i, j, n, returnpid;
if (pipe(fd) < 0) {
printf("Pipe creation error ");
exit(1);
}
returnpid = fork();
if (returnpid < 0) {
printf("Fork failed ");
exit(1);
} else if (returnpid == 0) { /* child */
/* close(fd[1]); child forgets to close out */
while ((n = read(fd[0],buf,80)) > 0) { /* read from pipe */
buf[n] = 0;
printf("
sleep(3); /* add this line to delay child */
for (i = 0, j = 0; i < n; i = i+2, j++) /* skip the odd characters */
buf2[j] = buf[i];
write(fd[1],buf2,j); /* accidentally echo back new string via fd[1] */
}
close(fd[0]);
printf("
} else { /* parent */
close(fd[0]); /* close parent in */
while (1) {
printf("
n = read(STDIN_FILENO,buf,80); /* read a line */
if (n <= 0) break; /* EOF or error */
buf[--n] = 0;
printf("
for (i = 0; i < n; i++) /* encrypt */
if (buf[i] >= 'a' && buf[i] <= 'z')
buf[i] = mapl[buf[i]-'a'];
else if (buf[i] >= 'A' && buf[i] <= 'Z')
buf[i] = mapl[buf[i]-'A']-('a'-'A');
else if (buf[i] >= '0' && buf[i] <= '9')
buf[i] = mapd[buf[i]-'0'];
printf("
write(fd[1],buf,n); /* send the encrypted string */
}
close(fd[1]);
wait(NULL);
printf("
}
exit(0);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
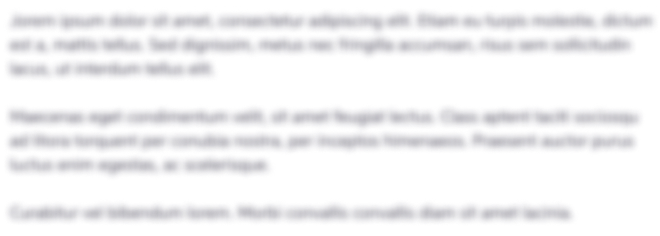
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started