Question
Override the toString() method and equals() method inside your class you created from Assignment 5. [2 points] Create a Copy Constructor in your class from
Override the toString() method and equals() method inside your class you created from Assignment 5. [2 points]
Create a Copy Constructor in your class from Assignment 5. [1 point]
Create a static field in your class called counter. It should increment everytime an object is created. [1 point]
Create a static method that displays the counter value. Also call this method at the end of your main method. [1 point]
Use the "this" reference in all your setter (mutator) methods and also use the "this" reference in your default constructor to call the parameterized constructor. [1 point]
Demonstrate that the copy Constructor, toString, and equals methods work in your main method [1 point]
import java.awt.Color;
public class Car
{
private Color color;
private int numDoors;
private double topSpeed;
// Default constructor
Car()
{
this(Color.BLACK, 4, 150.0);
}
// Parameterized constructor
Car(Color color, int numDoors, double topSpeed)
{
this.color = color;
this.numDoors = numDoors;
this.topSpeed = (topSpeed);
}
// Copy constructor
public Color getColor()
{
return color;
}
public void setColor(Color color)
{
this.color = color;
}
public int getnumDoors()
{
return numDoors;
}
public void setnumDoors(int newNumber)
{
if (newNumber < 0)
{
System.out.println("Invalid!");
return;
}
numDoors = newNumber;
}
public double gettopSpeed()
{
return topSpeed;
}
public void settopSpeed(double newtopSpeed)
{
if (newtopSpeed < 0)
{
System.out.println("Invalid!");
return;
}
topSpeed = newtopSpeed;
}
public void display()
{
System.out.println("Color: " + color);
System.out.println("Number of Doors: " + numDoors);
System.out.println("Top Speed: " + topSpeed);
}
@Override
public String toString()
{
return ("Color: " + color + " numDoors: " + numDoors + " topSpeed: " + topSpeed);
}
public boolean equals(Car other)
{
return (this.color == other.color) && (this.numDoors == other.numDoors) && (this.topSpeed == other.topSpeed) ;
}
}
--------------------------------------------------------------------------------------------------------------------
import java.awt.Color;
public class Demo {
public static void main(String[] args)
{
Car car1 = new Car();
Car car2 = new Car(Color.RED, 4, 100);
// car1.setColor("White");
// car1.setnumDoors(4);
// car1.settopSpeed(140.0);
// car1.display();
//
// Car car2 = new Car(
// "blue", 3, 126.0);
System.out.println(car1.equals(car2));
return;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
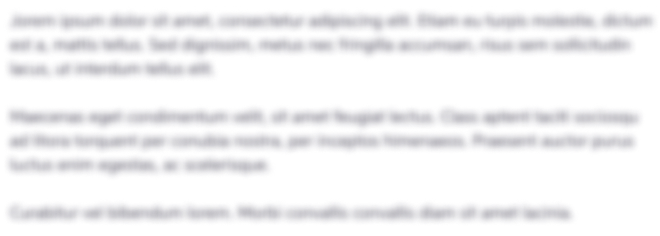
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started