Question
Overview For this assignment, write the functions that can be used to manipulate and test an integer number. The main routine has been provided for
Overview
For this assignment, write the functions that can be used to manipulate and test an integer number.
The main routine has been provided for you (it can be downloaded from http://faculty.cs.niu.edu/~byrnes/csci240/pgms/assign6.cpp). All that is needed for this assignment is to add the protoypes and implementations for the various required functions to the CPP file that is provided.
MIAN ROUTINE PROVIDED:
#include#include #include #include using namespace std; const int NUM_VALS = 10; //the maximum number of values to use /********* Put the function prototypes below this line *********/ int main() { int number, //holds the random number that is manipulated and tested loopCnt; //controls the loop //set the seed value for the random number generator //Note: a value of 1 will generate the same sequence of "random" numbers every // time the program is executed srand(31); //Generate 10 random numbers to be manipulated and tested for( loopCnt = 1; loopCnt <= NUM_VALS; loopCnt++ ) { //Get a random number number = rand(); //Display the sum of adding up the digits in the random number, the reversed //random number, and whether or not the number is palindromic or a prime number cout << "The number is " << number << endl << "----------------------------------------" << endl << "Adding the digits result" << setw(16) << sumDigits( number ) << endl << "Reversing the digits result" << setw(13) << reverse(number) << endl << "Is the number a palindrome?" << setw(13) << (isPalindrome(number)? "Yes" : "No") << endl << "Is the number prime?" << setw(20) << (isPrime(number)? "Yes" : "No") << endl << endl << endl; } /** If the extra credit is being attempted, call the extra function below this line **/ return 0; } /********* Code the functions below this line *********/
The Functions
The following functions are required for this assignment:
int sumDigits( int num )
This function will calculate and return a sum by adding the individual digits in an integer number. For example, if num contains 641, the function should return 11 (the sum of 6 + 4 + 1).
The function takes one integer argument: the number to use in calculating the sum. It returns an integer value that represents the sum of the digits.
To isolate each of the digits in an integer number, use modulus division by 10. For example, 641 % 10 = 1. To remove a digit from an integer number, use integer division by 10. For example, 641 / 10 = 64.
Therefore, to calculate the sum of the digits in an integer number, the logic that is described above can be put inside of a loop that executes until there are no longer any digits to remove from the integer number (ie. when the quotient is 0). Each iteration of the loop should take each isolated number and add it to a running total.
int reverse( int num )
This function will reverse and return the digits in an integer number. For example, if num contains 641, the function should return 146.
The function takes one integer argument: the number to use. It returns an integer value that represents the reversed number.
To reverse the digits in a number, take advantage of the two properties that were described in the description of the sumDigit function. Namely, that modulus division by 10 can be used to isolate a number and integer division by 10 can be used to remove a number. The reversed number can then be calculated by using simple addition.
To start the process, initialize a variable (say reverseNum) to 0. This will be the variable that holds the reversed number. Inside of a loop that executes as long as the integer number (the one in the argument - num) has digits to be removed:
multiply reverseNum by 10
isolate a digit from num
add the isolated digit to reverseNum
remove a digit from num
For example, assuming num contains 641, following the process described above results in:
Iteration #1:
0 * 10 = 0 (remember that reverseNum initially contains 0)
isolate the 1 from 641
0 + 1 = 1
remove the 1 from 641 so num contains 64
Iteration #2:
1 * 10 = 10
isolate the 4 from 64
10 + 4 = 14
remove the 4 from 64 so num contains 6
Iteration #3:
14 * 10 = 140
isolate the 6 from 6
140 + 6 = 146
remove the 6 from 6 so num contains 0
Now the digits in num have been reversed and the result is 146 in reverseNum.
bool isPalindrome( int num )
This function will test to see if an integer number is a palindrome. For an integer to be a palindrome, it must "read" the same forward and backward. So 464 is a palindrome, while 15 is not a palindrome.
The function takes one integer argument: the number to test. It returns a boolean value that represents whether the integer argument is or is not a palindrome.
To determine if an integer number is a palindrome, call the reverse() function that was previously written and compare the value that is returned from the function with the integer argument. If the returned value is equal to the integer argument, the number is a palindrome and true should be returned. If they're not equal, the number is not a palindrome and false should be returned.
bool isPrime( int num )
This function will test to see if an integer number is a prime number. For an integer to be a prime number, it must be divisible by only 1 and itself. So 11 is a prime number, while 15 is not a prime number.
The function takes one integer argument: the number to test. It returns a boolean value that represents whether the integer argument is or is not a prime number.
There are a number of different ways to determine if an integer number is a prime number, the following is just one of the ways. Write a loop that is controlled by an integer counter. The counter should start with a value of 2 and the loop should continue one value at a time as long as the counter is less than or equal to half of the integer number that is being tested. Inside of the loop, if the integer number is divisible by the counter, the integer number is not prime and false should be returned.
If the loop is able to complete execution, the integer number is prime and true should be returned.
Program Requirements
Each function must have a documentation box explaining:
/*************************************************************** Function: bool isPrime( int ) Use: This function determines if a number is a prime number Arguments: an integer, the number to be tested Returns: a boolean value that indicates if the number is or is not prime ***************************************************************/
See the documentation standards on the course webpage for more examples or if further clarification is needed.
As with the previous assignment and the assignments until the end of the semester, complete program documentation is required. For this assignment, that means that line documentation AND function documentation boxes are needed. In regards to line documentation, there is no need to document every single line, but logical "chunks" of code should be preceded by a line or two that describe what the "chunk" of code does.
its name
its use or function: that is, what does it do? What service does it provide to the code that calls it?
a list of its arguments briefly describing the meaning and use of each, and in particular whether the function modifies each one
the value returned (if any) or none
notes on any unusual features, assumptions, techniques, etc.
The main routine that has been provided may not be altered in the source code that is graded. However, as the program is being developed, you may comment out some of the function calls so that the functions can be coded one at a time. You may also want to think about making the loop execute fewer times as you're developing the functions.
Hand in a copy of the source code (CPP file) using Blackboard.
Output
The number is 139 ---------------------------------------- Adding the digits result 13 Reversing the digits result 931 Is the number a palindrome? No Is the number prime? Yes The number is 13240 ---------------------------------------- Adding the digits result 10 Reversing the digits result 4231 Is the number a palindrome? No Is the number prime? No The number is 17971 ---------------------------------------- Adding the digits result 25 Reversing the digits result 17971 Is the number a palindrome? Yes Is the number prime? Yes The number is 27503 ---------------------------------------- Adding the digits result 17 Reversing the digits result 30572 Is the number a palindrome? No Is the number prime? No The number is 927 ---------------------------------------- Adding the digits result 18 Reversing the digits result 729 Is the number a palindrome? No Is the number prime? No The number is 2453 ---------------------------------------- Adding the digits result 14 Reversing the digits result 3542 Is the number a palindrome? No Is the number prime? No The number is 6547 ---------------------------------------- Adding the digits result 22 Reversing the digits result 7456 Is the number a palindrome? No Is the number prime? Yes The number is 7076 ---------------------------------------- Adding the digits result 20 Reversing the digits result 6707 Is the number a palindrome? No Is the number prime? No The number is 30789 ---------------------------------------- Adding the digits result 27 Reversing the digits result 98703 Is the number a palindrome? No Is the number prime? No The number is 3862 ---------------------------------------- Adding the digits result 19 Reversing the digits result 2683 Is the number a palindrome? No Is the number prime? No
Step by Step Solution
There are 3 Steps involved in it
Step: 1
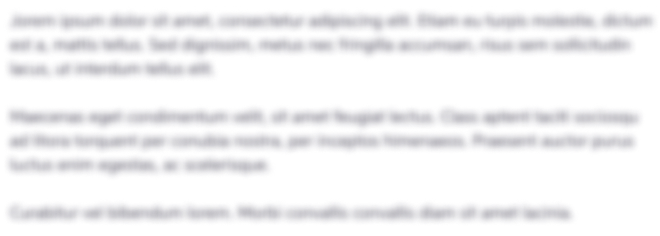
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started