Question
Overview This hands-on lab allows you to follow and experiment with the critical steps of developing a program including the program description, analysis, test plan,
Overview This hands-on lab allows you to follow and experiment with the critical steps of developing a program including the program description, analysis, test plan, design (using pseudocode), and implementation with C code. The example provided uses sequential and selection statements. Program Description This program will calculate the sum of 5 integers.
The program will ask the user to 5 integers. If the sum of the numbers is greater than 100, a message is printed stating the sum is over 100. The design step will include both pseudocode.
Analysis
I will use sequential, and selection programming statements.
I will define six integer numbers: value1, value2, value3, value4, value5 and sum. The value1, value2, value3, value4 and value5 variables will store the integers input by the user. The sum will store the sum of the 5 values.
The sum will be calculated by this formula: sum = value1 + value2 + value3 + value4 + value5
For example, if the first values entered were value 1=1, value 2=1, value 3=2,value 4=2 and value 5=3 respectively: sum = 1 + 1 + 2 + 2 + 3 = 9
The additional selection statement will be of this form:
If sum > 100 then
print "Sum is over 100"
End If
Test Plan To verify this program is working properly the input values could be used for testing:
Test Case Input Expected Output
1 value1=1 value2=1 value3=1 value4=0 Value5=2 Sum = 5
2 value=100 value=100 value=100 value=100 value=200 Sum = 600
Sum is over 100
3 value= -100 value= -100 value= -200 value = 0 value= 200 Sum = -200
Pseudocode // This program will calculate the sum of 5 integers.
// Declare variables Declare value1, value2, value3, value4, value5, sum as Integer
//Initialize Sum to 0
Set sum = 0
// Enter values
Print Enter an Integer for value1
Input value1
Print Enter an Integer for value2
Input value2
Print Enter an Integer for value3
Input value3
Print Enter an Integer for value4
Input value4
Print Enter an Integer for value5
Input value5
// Calculate sum
sum = value1 + value2 + value3 + value4 + value5
// Print results and messages
Print Sum is + sum
If (sum > 100)
Printf Sum is over 100
End if
C Code The following is the C Code that will compile in execute in the online compilers.
// C code
// This program will calculate the sum of 5 integers.
// Developer: Faculty CMIS102
// Date: Jan 31, XXXX
#include
int main ()
{
/* variable definition: */
int value1,value2,value3,value4,value5,sum;
/* Initialize sum */
sum = 0;
printf("Enter an Integer for value1 ");
scanf("%d", &value1);
printf("Enter an Integer for value2 ");
scanf("%d", &value2);
printf("Enter an Integer for value3 ");
scanf("%d", &value3);
printf("Enter an Integer for value4 ");
scanf("%d", &value4);
printf("Enter an Integer for value5 ");
scanf("%d", &value5);
sum = value1 + value2 + value3 + value4 + value5;
printf("Sum is %d " , sum );
if (sum >100)
printf("Sum is over 100 ");
return 0;
}
Setting up the code and the input parameters in ideone.com: Note the input integer values are 100, 100, 100, 200 and 100, for this test case. You can change these values to any valid integer values to match your test cases.
Learning Exercises for you to complete
1. Demonstrate you successfully followed the steps in this lab by preparing screen captures of you running the lab as specified in the Instructions above.
2. Change the C code to sum 10 integers as opposed to 5? (Hint: Please dont use arrays or Loops for this. We will be using those features in another week.) Support your experimentation with a screen capture of executing the new code
3. Modify the code to print an additional selection statement if the sum of the value is negative Support your experimentation with a screen capture of executing the new code.
4. Prepare a new test table with at least 3 distinct test cases listing input and expected output for the selection statement you created in the previous exercise.
5. Create your own C code implementation of one of the following mathematical formulas:
a. y = mx + b; (slope of a line) Assume the user will be prompted to input m, x and b and the program will calculate y. If the value of y is greater than 10, inform the user the value is greater than 10.
b. a = PI * r*r; (area of circle). Assume the user will be prompted to input the radius r. You can define PI as 3.1416. . If the value of a is greater than 10, inform the user the value is greater than 10.
c. v = 4/3 PI r*r*r; (volume of sphere) Assume the user will be prompted to input the radius r. You can define PI at 3.1416. If the value of v is greater than 10, inform the user the value is greater than 10.
Be sure you provide not only the C code but a test table with at least 3 distinct test cases listing input and expected output your mathematical formula.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
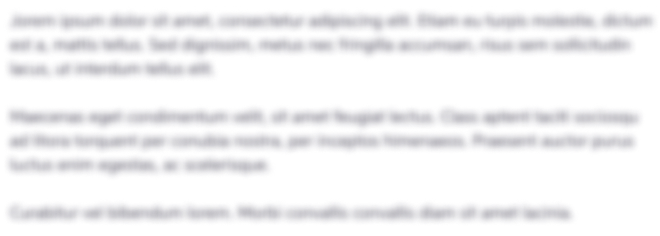
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started