Question
package algs34; import java.util.*; import stdlib.*; /* * Complete the methods of MyFB so that it works. * Each method must work in the worst
package algs34;
import java.util.*; import stdlib.*;
/* * Complete the methods of MyFB so that it works. * Each method must work in the worst case time given below (assuming uniform hashing and ignoring string lengths): * * addPerson must take constant time * * addFriendship must take constant time * * queryFriendship must take constant time * * removeFriendship should take constant time * * lookupFriends should take time proportional to the number of * friends that the named person has (or less) * * Your solution should use a single field of type * * HashMap
// a person "exists" after they are added using addPerson // addPerson does nothing if p already exists public void addPerson (Person p) { if (DEBUG) { StdOut.format ("P %s ", p); } // TODO } // addFriendship does nothing if p1 and p2 are already friends or if one does not exist public void addFriendship (Person p1, Person p2) { if (DEBUG) { StdOut.format ("F %s %s ", p1, p2); } // TODO } // removeFriendship does nothing if p1 and p2 are not friends or if one does not exist public void removeFriendship (Person p1, Person p2) { if (DEBUG) { StdOut.format ("U %s %s ", p1, p2); } // TODO } // queryFriendship returns false if p1 and p2 are not friends or if one does not exist public boolean queryFriendship (Person p1, Person p2) { if (DEBUG) { StdOut.format ("Q %s %s ", p1, p2); } // TODO return false; } // lookupFriends returns null or empty iterable if p does not exists public Iterable lookupFriends (Person p) { if (DEBUG) { StdOut.format ("L %s ", p); } // TODO return null; }
public static void main (String[] args) { Trace.showBuiltInObjects (true); //Trace.run (); /* * The first line below causes "in" to read from the console. You can * change this to read from a file, by using something like the line * below, where "input.txt" is a file you create in your eclipse * workspace. The file should be in the same folder that contains "src" * "bin" and "lib": */
//In[] inputFiles = new In[] { new In () }; // from console //In[] inputFiles = new In[] { new In ("input.txt") }; // from file In[] inputFiles = new In[] { new In ("input.txt"), new In () }; // from file, then console MyFB fb = new MyFB (); StdOut.println ("Enter one of the following:"); StdOut.println (" P name age -- add person"); StdOut.println (" F name1 age1 name2 age2 -- add friendship"); StdOut.println (" U name1 age1 name2 age2 -- remove friendship"); StdOut.println (" Q name1 age1 name2 age2 -- query friendship"); StdOut.println (" L name age -- lookup friends"); StdOut.println (" R -- restart"); StdOut.println (" X -- exit"); for (In in : inputFiles) { while (in.hasNextLine ()) { //StdOut.println (fb. ...); // while debugging, print debugging info here! You can print maps, sets, lists. String action; try { action = in.readString (); } catch (NoSuchElementException e) { continue; } switch (action) { case "P": case "p": { Person p = makePerson (in); fb.addPerson (p); break; } case "F": case "f": { Person p1 = makePerson (in); Person p2 = makePerson (in); fb.addFriendship (p1, p2); break; } case "U": case "u": { Person p1 = makePerson (in); Person p2 = makePerson (in); fb.removeFriendship (p1, p2); //Trace.draw (); break; } case "Q": case "q": { Person p1 = makePerson (in); Person p2 = makePerson (in); boolean result = fb.queryFriendship (p1, p2); StdOut.println (result ? "Yes" : "No"); break; } case "L": case "l": { Person p = makePerson (in); Iterable result = fb.lookupFriends (p); if (result != null) { for (Person friend : result) { StdOut.print (friend); StdOut.print (" "); } } StdOut.println (); break; } case "R": case "r": fb = new MyFB (); break; case "X": case "x": System.exit (0); } } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
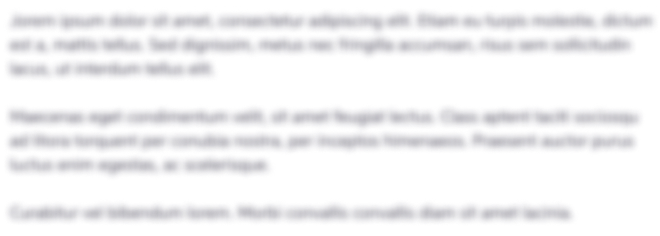
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started