Question
package linkedlists; public class CircularlyLinkedList { private static class Node { private E element; private Node next; // a reference to the subsequent node in
package linkedlists; public class CircularlyLinkedList
public Node(E e, Node
public Node
public void setNext(Node
// instance variables of the CircularlyLinkedList private Node
/** Number of nodes in the list */ private int size = 0; // number of nodes in the list
/** Constructs an initially empty list. */ public CircularlyLinkedList() { } // constructs an initially empty list public int size() { return size; } public boolean isEmpty() { return size == 0; } public E first() { // returns (but does not remove) the first element if (isEmpty()) return null; return tail.getNext().getElement(); // the head is *after* the tail } public E last() { // returns (but does not remove) the last element if (isEmpty()) return null; return tail.getElement(); } public void rotate() { // rotate the first element to the back of the list if (tail != null) // if empty, do nothing tail = tail.getNext(); // the old head becomes the new tail } public void addFirst(E e) { // adds element e to the front of the list if (size == 0) { tail = new Node<>(e, null); tail.setNext(tail); // link to itself circularly } else { Node
// } }
In this exercise you will add a clone() method to CircularlyLinkedList class from above example. Write a main method to test the new method. Make sure to properly link the new chain of nodes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
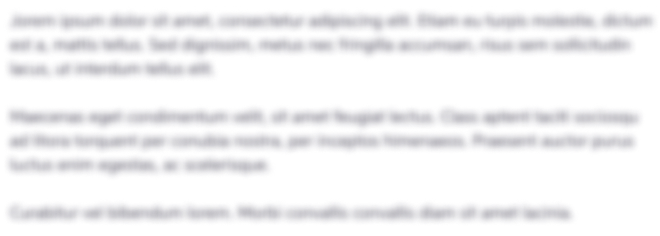
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started