Question
PART 1 1.1 Incremental Building From a design perspective, you should build and test your processor incrementally. This means building one small part and testing
PART 1
1.1 Incremental Building From a design perspective, you should build and test your processor incrementally. This means building one small part and testing that it behaves the way you want before continuing on to the next part. Here is a list of parts that can be tested independently (with more details in upcoming sections): 1. Processor phases 2. ALU function 3. Register updates 4. Program Counter advancing 5. PC memory reference 6. Instruction Register loading 1.2 Clock A processor has a single clock that causes every state change in the whole processor. Different components use the clock, so build your clock in your main processor circuit and create clock inputs for each other chip you create. 1.3 Control Unit The six processor phases, driven by the ticking clock, are the root of the processor. You already implemented this part in a previous lab. If you are happy with your circuit, you can copy/paste it directly! If you want a simpler looking circuit, the Counter component in the Memory directory in Logisim can count from 0-5, to the same effect. For this implementation, I recommend starting with Store as phase 000. That way, the first full phase that runs when you start the processor is the first Fetch phase. The control unit also houses the Instruction Register and the Program Counter. 1.3.1 Interface Each processor component has an interface that defines what its outputs are and what its inputs are. The control unit should have: Inputs Clock Instruction Register Program Counter (for eventual JMP instruction) Outputs 6 phase signals Instruction Program Counter 1 of 3 COMP1200 - Computer Organization Spring 2018 Final Project (part 1 of 2) Due: turned in on Blackboard by April 16 @ 11:59pm 1.4 ALU operations The three LC-3 numeric operations are: ADD, AND, and NOT. The ALU should calculate all three of these operations, given its input. Then, based on which operation the current instruction specifies, use a multiplexer to pick the correct calculation to output. The ALU should also decide whether to use the values from two registers or from one register and the sign-extended immediate value. There is a Bit Extender component under Wiring that will sign-extend a 5-bit value into a 16-bit value. 1.4.1 Interface Inputs Instruction Register 2 source register values Outputs Resulting value 1.5 Register File LC-3 has a bank of 8 registers which can be referenced and modified. An instruction can refer to the values of up to two registers, and change one register. The register chip needs 3 register names as inputs. One of those names will be a destination register, where a value will be stored. The other two names will be source registers, where values will be referenced. Use a demux to decide which of the registers might be changed. Use two muxes to decide, based on the source register names, which values reference. 1.5.1 Interface Inputs Clock Destination register Destination value 2 source registers Write enabled (whether a register is being changed) Outputs Two source register values 1.6 Memory Memory is not actually a separate chip the same way that the other components are, because Logisim has implemented most of the hard work for us. The RAM component will hold all of our memory for instructions and data. I recommend changing the Data Interface property to Separate load and store ports, but you can read the Library Reference documenentation and make your own decision on what makes sense to you. You can use a MAR or MDR if you like, but the memory should work fine without it. For the first part of the lab, the only possible address comes from the PC, the only possible output goes to the IR, and data is never stored, only loaded. 1.7 PC updates The program counter should advance by 1 each decode phase. You can implement this easily by feeding the PC value into an adder with x0001 as the other input. Then, store the result back into the PC. 2 of 3 COMP1200 - Computer Organization Spring 2018 Final Project (part 1 of 2) Due: turned in on Blackboard by April 16 @ 11:59pm 1.8 IR loading The instruction register should be updated each fetch phase, based on the PC value. This involves sending the PC address to the RAM and storing the data result in the IR. 2 Testing Testing each part as you make it is important in the design process. Since the ALU is just a combinational circuit, you can give it certain inputs and check that the outputs are what you expect. For sequential circuits like the registers and control unit, you can set inputs to specific values and then run the clock to see if the result is what you expect. When you are testing instructions, you probably want to go through a testing cycle where you reset the machine, click on the x0000 memory location to set the instruction, and run the clock to see how the instruction runs. If it doesnt run correctly, you can see exactly when it does something incorrect, modify the circuit and start the testing cycle again. 3 Final Project: Part 2 Part 2 of the final project will involve implementing program redirection and loading/storing memory: the JMP instruction, and LD, ST, LDR, STR.
PART 2
Read this document, but do not start implimenting this half of the project until you have finished your arithmetic instructions. The full list of instructions to implement is as follows: ADD (register and immediate) AND (register and immediate) NOT JMP ST STR LD LDR Refer to the instruction reference for JMP functionality. The lecture slides are not correct (there have been some ISA updates). 2 Implementing arithmetic instructions If your ADD/AND/NOT operations are not correctly working yet, spend more time on the documentation in part 1. The only section that might be interesting here is the section on timing. 3 Special Registers In addition to the general registers and PC/IR (which should be functioning already), LC-3 has several special-purpose registers. Depending on your implementation in part 1, you may or may not have MDR and MAR registers connected to the RAM chip. It is possible for your processor to work either way, and timing might be a little easier without needing the address and data values to propagate across an extra register. There are also condition code registers in LC-3. The only time these are referenced is for branch instructions, and our implementation have those! That means that we dont need to worry about condition codes at all. 4 Timing Propogating information through the processor can be tough, especially if you have to move information from a register to the MAR before you can move information from memory to the MDR back to a register. The descriptions of each instruction describe when they should be moving information around, but it isnt always possible to do everything you need in one clock cycle in Logisim. For example, my implementation uses a MDR. I move the next instruction from memory to the MDR at the end of the Store phase, and then move the MDR to the IR at the end of the Fetch phase. For store instructions, I move the MDR to memory at the end of the Execute phase. There is not one specific correct way to time updates for each of your registers, but you have to make sure that the different memory locations are updated for each instruction in the proper order. 1 of 2 COMP1200 - Computer Organization Spring 2018 Final Project (part 2 of 2) Due: turned in on Blackboard by April 16 @ 11:59pm 5 JMP instruction The JMP instruction (as it appears in the LC-3 reference) copies the src1 register to the PC. This means that src1s value should be the value that is input to the control unit, connecting to the program counter. In the control unit, you have to decide, based on the current instruction, whether you want to replace the PC with that src1 value or not. 6 LD/LDR instructions With ALU instructions and the JMP instruction, there is only one interaction with the memory: the PC is sent to memorys address and memorys data output is sent to the IR. This means that memorys Load bit should only be set to 1 during the fetch phase (or whichever phase you move the current instruction to the IR). All of that changes with load instructions. Now, memory should be active in order to copy a value from memory to a register. Based on the instruction and phase, you have to decide which address to send to memory: when you fetch, the address will be the PC. When you execute a load instruction, the address will either be based on the PC and PCoffset9 (for LD) or the value in BaseR (for LDR). You also have to decide where to send the data value output based on the instruction and phase: either to the IR, or to the destination value of the registers file. 7 ST/STR instructions Store instructions are the only instructions that can change memory. That means that the RAM component should only have the str input set to 1 with a store instruction (and at the proper phase). Depending on the instruction and phase, the data input to memory might be the value of the source register (which is, only in this case, the register named in bits 9-11). The input to the address is the value in BaseR + offset6 (for STR) or the relative address formed with PCoffset9 (for ST). 8 README writeup In addition to the Logisim circuit file, write a text file that describes your circuit. The README should include any unusual behavior in your processor (for example, note if your processor has to start in the Store state or the IR needs to be entered manually the first time). You should also include short descriptions of tests that you have run for each instruction. If your tests are working correctly, note what the results were and why that is a good test for the instruction. If an instruction is not working, or is partially working, note what you expected the processor to do. Describe what goes wrong, when it goes wrong, and how it might be fixed. 9 Submission Save your circuit file as LC-3.circ. Upload the circuit and the README text file to Blackboard. 2 of 2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
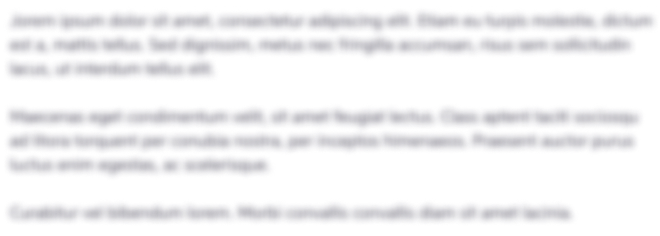
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started