Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Part 1 : Warehouse Create the class Warehouse with the following methods: public void addProduct ( String product, int price, int stock ) , which
Part : Warehouse Create the class Warehouse with the following methods: public void addProductString product, int price, int stock which adds a product to the warehouse with the price and stock balance given as parameters. public int priceString product which returns the price of the product it received as a parameter. If the product hasn't been added to the warehouse, the method must return The products in the warehouse and in the next part their stock must be stored in a variable of the type Map! The object created can be a HashMap, but its type must be the Mapinterface, rather than any implementation of that interface. What it needs to do in Main: add a product, then print out the product with the price. Part : Products stock balance Save the stock balance of products in a variable with the Map type, in the same way the prices were stored. Supplement the warehouse with the following methods: public int stockString product returns the current remaining stock of the product in the warehouse. If the product hasn't been added to the warehouse, the method must return public boolean takeString product reduces the stock remaining for the product it received as a parameter by one, and returns true if there was stock remaining. If the product was not available in the warehouse the method returns false. A products stock can't go below zero. To do in Main: use addProduct method, use the stock, and take to see if it prints true or false for items actually being taken or not. Part : Listing the products Let's add one more method to the warehouse: public Set products returns the names of the products in the warehouse as a Set This method is easy to implement with HashMap. You can get the products in the warehouse from either the Map storing the prices or the one storing current stock, by using the method keySet Needs to: print the all the products in the warehouse. Part : Item Items can be added to the shopping cart which we'll add soon An item is a product with a quantity. You for example add an item representing one bread to the cart, or add an item representing coffees. Create the class Item with the following methods: public ItemString product, int qty int unitPrice; a constructor that creates an item corresponding to the product given as a parameter. qty tells us how many of the product are in the item, while unitPrice is the price of a single product. public int price return the price of the item. You get the items price by multiplying its unit price by its quantityqty public void increaseQuantity increases the quantity by one. public String toString returns the string representation of the item. which must match the format shown in the example below. Needs to: increase the quantity of the item and say how many of that item it contains. Part : Shopping cart We finally get to implement the shopping cart class! Internally, ShoppingCart stores products added there as Itemobjects. ShoppingCart must have an instance variable with either the Map type, or the List type. Don't add any other instance variable to the ShoppingCart class, besides the List or Map used to store the items. NB: If you save the items in a Map type variable, you'll finds its values method to be quite useful for going though all the items objects stored in it for both this part of the exercise and the next. First let's give ShoppingCart a constructor with no parameters and these methods: public void addString product, int price adds an item to the cart that matches the product given as a parameter, with the price given as a parameter. public int price returns the total price of the shopping cart. Needs to: add product and price, then print out cart price. Part : Printing the cart Implement the method public void print for the shopping cart. The method prints the Itemobjects in the cart. The order they are printed in is irrelevant. Eg the print of the cart in the previous example would be: buttermilk: Part : One item per product Let's change our cart so that if a product is being added thats already in the cart, we don't add a new item, but instead update item already in the cart by calling its increaseQuantity method. Ex: add the milk and buttermilk, and they get their own itemobjects. When more milk is added to the cart, increase the quantity in the item representing milk. Part : We now have all the parts we need for our "online store", except the store itself. Let's make that next. Our store has a warehouse that includes all our products. For each 'visit' we have a shopping cart. Every time the customer chooses a product its added to their cart if its available in the warehouse. At the same time, the stock in the warehouse is reduced by one.
Part : Warehouse
Create the class Warehouse with the following methods:
public void addProductString product, int price, int stock which adds a product to the warehouse with the price and stock balance given as parameters.
public int priceString product which returns the price of the product it received as a parameter. If the product hasn't been added to the warehouse, the method must return
The products in the warehouse and in the next part their stock must be stored in a variable of the type Map! The object created can be a HashMap, but its type must be the Mapinterface, rather than any implementation of that interface.
What it needs to do in Main: add a product, then print out the product with the price.
Part : Products stock balance
Save the stock balance of products in a variable with the Map type, in the same way the prices were stored. Supplement the warehouse with the following methods:
public int stockString product returns the current remaining stock of the product in the warehouse. If the product hasn't been added to the warehouse, the method must return
public boolean takeString product reduces the stock remaining for the product it received as a parameter by one, and returns true if there was stock remaining. If the product was not available in the warehouse the method returns false. A products stock can't go below zero.
To do in Main: use addProduct method, use the stock, and take to see if it prints true or false for items actually being taken or not.
Part : Listing the products
Let's add one more method to the warehouse:
public Set products returns the names of the products in the warehouse as a Set
This method is easy to implement with HashMap. You can get the products in the warehouse from either the Map storing the prices or the one storing current stock, by using the method keySet
Needs to: print the all the products in the warehouse.
Part : Item
Items can be added to the shopping cart which we'll add soon An item is a product with a quantity. You for example add an item representing one bread to the cart, or add an item representing coffees.
Create the class Item with the following methods:
public ItemString product, int qty int unitPrice; a constructor that creates an item corresponding to the product given as a parameter. qty tells us how many of the product are in the item, while unitPrice is the price of a single product.
public int price return the price of the item. You get the items price by multiplying its unit price by its quantityqty
public void increaseQuantity increases the quantity by one.
public String toString returns the string representation of the item. which must match the format shown in the example below.
Needs to: increase the quantity of the item and say how many of that item it contains.
Part : Shopping cart
We finally get to implement the shopping cart class!
Internally, ShoppingCart stores products added there as Itemobjects. ShoppingCart must have an instance variable with either the Map type, or the List type. Don't add any other instance variable to the ShoppingCart class, besides the List or Map used to store the items.
NB: If you save the items in a Map type variable, you'll finds its values method to be quite useful for going though all the items objects stored in it for both this part of the exercise and the next.
First let's give ShoppingCart a constructor with no parameters and these methods:
public void addString product, int price adds an item to the cart that matches the product given as a parameter, with the price given as a parameter.
public int price returns the total price of the shopping cart.
Needs to: add product and price, then print out cart price.
Part : Printing the cart
Implement the method public void print for the shopping cart. The method prints the Itemobjects in the cart. The order they are printed in is irrelevant. Eg the print of the cart in the previous example would be:
buttermilk:
Part : One item per product
Let's change our cart so that if a product is being added thats already in the cart, we don't add a new item, but instead update item already in the cart by calling its increaseQuantity method. Ex: add the milk and buttermilk, and they get their own itemobjects. When more milk is added to the cart, increase the quantity in the item representing milk.
Part :
We now have all the parts we need for our "online store", except the store itself. Let's make that next. Our store has a warehouse that includes all our products. For each 'visit' we have a shopping cart. Every time the customer chooses a product its added to their cart if its available in the warehouse. At the same time, the stock in the warehouse is reduced by one.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
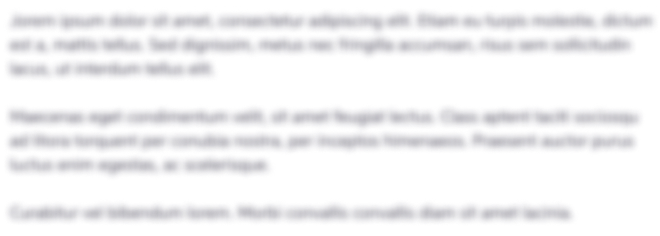
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started