Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Part 2 : The IDialLock Interface Note: All classes and interfaces you develop in Part 2 of the assignment should be placed in the model
Part : The IDialLock Interface
Note: All classes and interfaces you develop in Part of the assignment should be placed in the "model" package.
Develop an interface for a threedigit dial combination lock. The interface should be named IDiallock and should export the following abstract methods:
void reset resets the lock dial back to
void leftint turns the lock left by the specified number of ticks
void rightint turns the lock right by the specified number of ticks
int currentTick returns the tick number the dial is pointing to between maxTicks.
boolean pull attempts to pull open the lock; the method returns true if successful false in all other cases.
The lock opens if one performs the following sequence of turns:
right to the first secret digit.
then left to the second secret digit,
then right to the third secret digit.
The image below is a rough illustration of the sort of dial lock you'll be building:
: A "Helper Class" Representing Dial Turns
Before you implement the interface above, add the following helper class to the model package:
in: "Turnjava" in the model packago public final class Turn
we mark the class "final" to preclude extension
Turn direction Lleft, Rright
enum Direction
public Direction dir; public int stopDigit;
public TurnDirection dir, int stopbigit this.dir dirion
this.dir dir: this.stapoigit stopoigit:
eoverride public string tostring ff
: Developing a "Tracking" Implementation of the IDialLock Interface
To get started, make your class implement the IDialLock interface. The IDE should prompt you to insert 'default stub' implementations of the abstract methods in the interface.
Next, add the following fields to the class:
int maxTicks this stores an upper bound on the digits around the lock.
int these are the three secret digits needed to open the lock.
int currentTick the number the lock's dial is currently pointing towards between maxTicks
ListHere are some hintspseudocode for the methods of the tracking implementation, these methods should all be overridden from the IDialLock interface:
reset should just set currentTick back to and clear out the moves list.
leftint t:
someone turned left past need to account for the negative
if currentTick t then assign currentTick to maxTicks currentTick t
else assign currentTick to currentTick t
instantiate a Turn object, lt with direction left and supply currentTick for the stop digit
add lt to the moves list
rightint t:
currentTick currentTick t maxTicks
instantiate a Turn object, rt with direction right and supply currentTick for the stop digit
add rt to the moves list
pull:
This method is more complicated due to the fact that many consecutive right and left turns can be used to open the lock. In particular, the method needs to verify that:
first: only right turns were made, stopping at s
second: only left turns were made, stopping at s
and third: only right turns were made, finally stopping at s
To motivate a strategy for implementing this method, consider the following. THIS IS NOT THE CODE YOU PUT IN THE PULL METHOD this just goes in a Tester.java file to see if your pull method works:
in some Tester.java file's main method
final int maxTicks ;
instantiating a lock with secret digits and and maxTicks
IDialLock lock new TrLockImpl maxTicks;
ticking right to sie:
lock.right;
lock.right;
lock.right;
ticking left to sie:
lock.left;
lock.left;
ticking right to s
lock.right;
lock.right;
lock.right;
lock.right;
System.out.printlnlockpull; prints true
By the time lock.pull is called on the last line above, the lock object's move list if one were to examine it in the debugger would look like this:
moves R R R L L R R R R
R eg is the toString representation of a Turn object and can be read as: "Right turn that stopped on digit
One possible way of determining whether the lock should be opened given just the above list of Turn objects to work with is to partition the moves list into three separate lists:
firstRightTurns R R Rfirst: all first consecutive right turns in this.moves
secondLeftTurns L Lsecond: all first consecutive left turns in this.moves
thirdRightTurns R R R Rthird: all remaining consecutive right turns
Once you've managed to get the turns into these three lists, one could then say:
if firstRightTurnssize secondLeftTurnssize thirdRightTurns.size moves.size
return false;
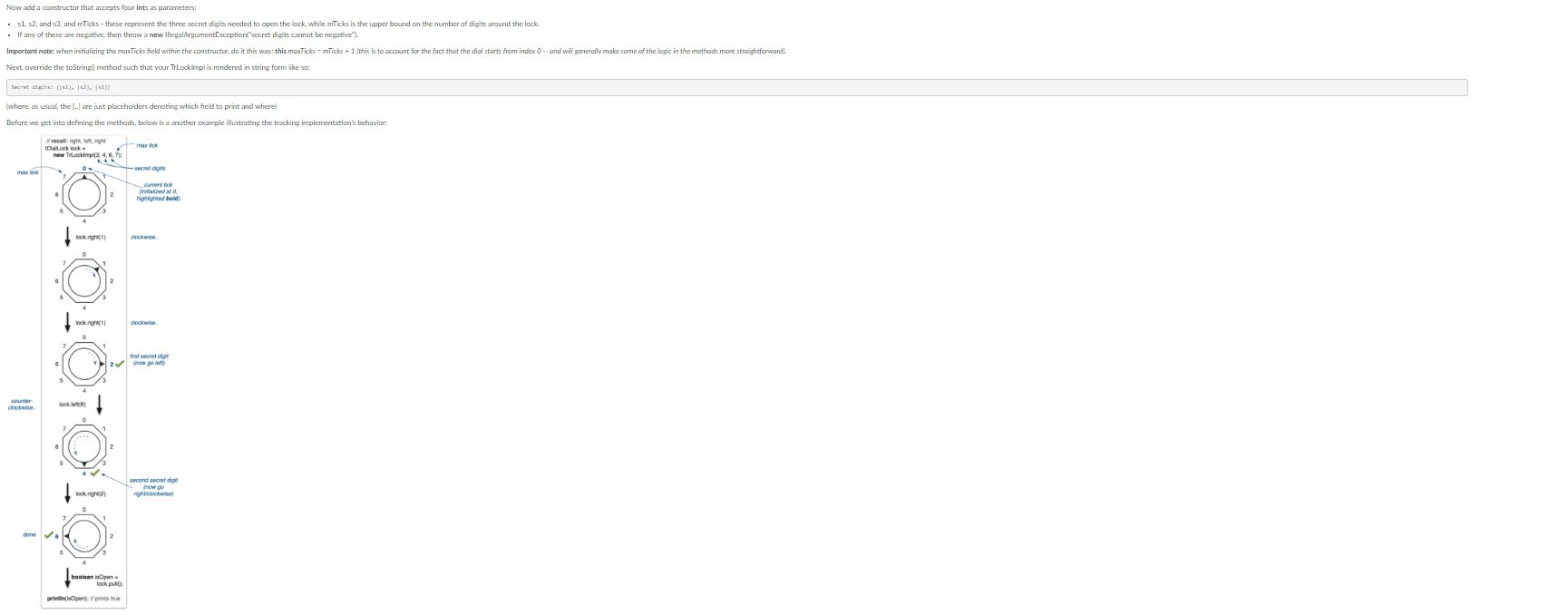
Step by Step Solution
There are 3 Steps involved in it
Step: 1
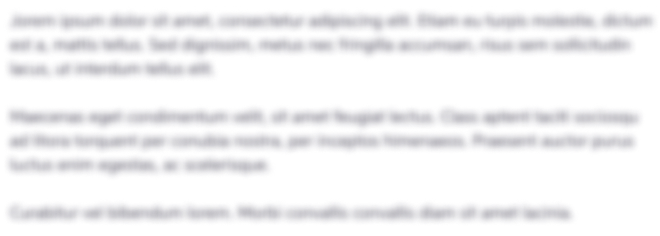
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started